Fork for marginal changes to UI library.
Dependents: Data-Management-Honka
Fork of UI by
UserInterface.cpp
00001 #include "mbed.h" 00002 #include "initDatabed.h" 00003 #include "UserInterface.h" 00004 00005 // UI button variables 00006 //int buttonA = 1; // state of remote button 00007 //int buttonA_prev = 1; 00008 00009 // push hold vars 00010 //float tHold = .6; // hold time for sit/stand 00011 //float tIdle = 2; // time out to confirm sit or stance (s) 00012 //int SSconfirm = 0; // sit/stand variable for confirmation process 00013 //float tRelease = .3; // time since last transmission to determine button release 00014 00015 00016 // various timers 00017 //Timer time_StateChange; 00018 //Timer time_pressA; 00019 //Timer time_pressB; 00020 00021 //int buttonB = 1; // state of remote button 00022 //int buttonB_prev = 1; 00023 //char __xbeeBuffer[250]; 00024 //int _dataCnt=0; 00025 UI_t UI = BUTTON_NONE; 00026 00027 //float _time_sinceA, _time_sinceB; //time since the button was first pressed 00028 00029 UserInterface::UserInterface(void): _buttonA(1), _buttonA_prev(1), _tHold(.6), _tIdle(2), _SSconfirm(0), _tRelease(.3), _buttonB(1), _buttonB_prev(1), _dataCnt(0) { 00030 } 00031 00032 void UserInterface::readBuffer() 00033 { 00034 while (xbeeUI.readable() && _dataCnt<250) { 00035 _xbeeBuffer[_dataCnt] = xbeeUI.getc(); 00036 _dataCnt++; 00037 } 00038 } 00039 00040 /** 00041 * This function returns an array of information about the received characters. count[0] is # of data bytes, count[1] is expected length of the message, given the data length bytes and presence 00042 * of escape characters. count[2] is position of the first data byte. 00043 * @param idx char array containing received data, beginning with the start byte 0x7e 00044 * @param count array that will contain the returned values 00045 * @author Michael Ling 00046 * @date 2/2/2015 00047 */ 00048 void UserInterface::find_length(char *idx, int *count) 00049 { 00050 int pos = 0; 00051 short length; 00052 //This segment reads the length bytes (bytes 2-3 of the message) 00053 if (*(idx+1) == 0x7d) { 00054 length = (*(idx+2) ^ 0x20) << 8; 00055 pos = 3; 00056 } else { 00057 length = *(idx+1) << 8; 00058 pos = 2; 00059 } 00060 if (*(idx+pos) == 0x7d) { 00061 length = length | (*(idx+pos+1)^0x20); 00062 pos += 2; 00063 } else { 00064 length = length | *(idx+pos); 00065 pos += 1; 00066 } 00067 count[0] = (int)length; 00068 //Length incremented by 1--we treat the checksum as a data byte, since it can also be escaped 00069 length += 1; 00070 //Checks for escape characters--for every escape char found, the data section gets 1 byte longer 00071 for (short i = 0; i < length; i += 1) { 00072 if (*(idx+i) == 0x7d) { 00073 length += 1; 00074 } 00075 } 00076 count[1] = (int) length + 3; 00077 count[2] = pos; 00078 } 00079 00080 /** 00081 * This function returns true if calculated checksum matches the checksum at the end of the message. 00082 * @param idx char array containing received data, starting with start byte 0x7e 00083 * @param length number of data bytes in the message 00084 * @author Michael Ling 00085 * @date 2/2/2015 00086 */ 00087 bool UserInterface::checksum_check(char *idx, int length) 00088 { 00089 int pos = 0; 00090 int sum = 0; 00091 for(int i = 0; i < length; i++) { 00092 //In case of an escape character, the true value of the byte is the following byte XOR with 0x20 00093 if (*(idx+pos) == 0x7d) { 00094 sum += (*(idx+pos+1) ^ 0x20); 00095 pos += 2; 00096 } else { 00097 sum += *(idx+pos); 00098 pos += 1; 00099 } 00100 } 00101 //XBEE checksum: sum all data bytes, truncate the sum to the rightmost 8 bytes, and subtract that from 0xff 00102 sum = sum & 0x0ff; 00103 char calcsum = (char)(0xff - sum); 00104 char checksum; 00105 if (*(idx+pos) == 0x7d) { 00106 checksum = *(idx+pos)^0x20; 00107 } else { 00108 checksum = *(idx+pos); 00109 } 00110 if (checksum != calcsum) { 00111 return false; 00112 } 00113 return true; 00114 } 00115 00116 void UserInterface::checkUI_XBee() 00117 { 00118 int sum = 0; 00119 _buttonA_prev = _buttonA; 00120 _buttonB_prev = _buttonB; 00121 char * idx = strchr(_xbeeBuffer,0x7e); 00122 if (idx != NULL) { 00123 int size[3]; 00124 find_length(idx, size); 00125 printf("Size: %d, Datacount: %d\r\n", size[1], _dataCnt); 00126 if(_dataCnt >= size[1]) { 00127 00128 _buttonA = (_xbeeBuffer[idx-_xbeeBuffer+21]>>1) & 1; // on DIO1 00129 _buttonB = (_xbeeBuffer[idx-_xbeeBuffer+21]>>2) & 1; // on DIO2 00130 00131 if (checksum_check(idx+size[2], size[0])) { 00132 printf("Checksums match\r\n"); 00133 } else { 00134 printf("Checksums don't match\r\n"); 00135 } 00136 _dataCnt = 0; 00137 } 00138 if(sum == 0x79c) { 00139 if (_buttonA == 0 && _buttonA_prev == 1) {//buton was just pressed 00140 _time_pressA.reset(); 00141 _time_pressA.start(); 00142 _time_sinceA = 0; 00143 } 00144 00145 else if(_buttonA == 0) { 00146 _time_sinceA = _time_pressA; //button is still pressed 00147 } 00148 00149 if (_buttonB == 0 && _buttonB_prev == 1) {//button was just pressed 00150 _time_pressB.reset(); 00151 _time_pressB.start(); 00152 _time_sinceB = 0; 00153 } else if(_buttonB == 0) { 00154 _time_sinceB = _time_pressB; //button is still pressed 00155 } 00156 } 00157 00158 if((_time_pressA-_time_sinceA) >= _tRelease) { //button was released 00159 if(_time_pressA-_tRelease >= _tHold) { //if the button was held before released 00160 UI = BUTTON_A_HOLD; 00161 } else { 00162 UI = BUTTON_A_PRESS; 00163 } 00164 _buttonA = 1; //button A is released 00165 _time_pressA.stop(); //reset the button A timer 00166 _time_pressA.reset(); 00167 } 00168 if(_time_pressB-_time_sinceB >= _tRelease) { //button was released 00169 if(_time_pressB-_tRelease >= _tHold) { //if the button was held before released 00170 UI = BUTTON_B_HOLD; 00171 } else { 00172 UI = BUTTON_B_PRESS; 00173 } 00174 _buttonB = 1; //button B is released 00175 _time_pressB.stop(); //reset the button B timer 00176 _time_pressB.reset(); 00177 } 00178 } 00179 memset(_xbeeBuffer,0xFF,250); 00180 _dataCnt = 0; 00181 } 00182 00183 /*void checkUI_XBee() 00184 { 00185 00186 _buttonA_prev = _buttonA; 00187 buttonB_prev = buttonB; 00188 while (xbeeUI.readable() && _dataCnt<250) { 00189 __xbeeBuffer[_dataCnt] = xbeeUI.getc(); 00190 _dataCnt++; 00191 if (__xbeeBuffer[_dataCnt]==0x7e) { 00192 for(int i=0; i<22; i++) { 00193 if(xbeeUI.readable() { 00194 __xbeeBuffer[_dataCnt] = xbeeUI.getc(); 00195 _dataCnt++; 00196 } 00197 } 00198 } 00199 char * idx=strchr(__xbeeBuffer,0x7e); 00200 _buttonA = (_xbeeBuffer[idx-_xbeeBuffer+21]>>1) & 1; // on DIO1 00201 buttonB = (_xbeeBuffer[idx-_xbeeBuffer+21]>>2) & 1; // on DIO2 00202 pc.printf("%x\r\n", *(idx+2)); 00203 _dataCnt=0; 00204 if (buttonA == 0 && buttonA_prev==1) {//buton was just pressed 00205 time_pressA.reset(); 00206 time_pressA.start(); 00207 _time_sinceA=0; 00208 } 00209 00210 else if(buttonA==0) { 00211 _time_sinceA=time_pressA; //button is still pressed 00212 } 00213 00214 if (buttonB == 0 && buttonB_prev==1) {//button was just pressed 00215 time_pressB.reset(); 00216 time_pressB.start(); 00217 _time_sinceB=0; 00218 } else if(buttonB==0) { 00219 _time_sinceB=time_pressB; //button is still pressed 00220 } 00221 } 00222 if((time_pressA-_time_sinceA)>=tRelease) { //button was released 00223 if(time_pressA-tRelease>=tHold) { //if the button was held before released 00224 UI = BUTTON_A_HOLD; 00225 } else { 00226 UI = BUTTON_A_PRESS; 00227 } 00228 buttonA=1; //button A is released 00229 time_pressA.stop(); //reset the button A timer 00230 time_pressA.reset(); 00231 } 00232 if(time_pressB-_time_sinceB>=tRelease) { //button was released 00233 if(time_pressB-tRelease>=tHold) { //if the button was held before released 00234 UI = BUTTON_B_HOLD; 00235 } else { 00236 UI = BUTTON_B_PRESS; 00237 } 00238 buttonB=1; //button B is released 00239 time_pressB.stop(); //reset the button B timer 00240 time_pressB.reset(); 00241 } 00242 00243 _dataCnt=0; 00244 memset(__xbeeBuffer,0xF,250); 00245 }*/ 00246 00247 void UserInterface::initializeUI() 00248 { 00249 xbeeUI.baud(115200); 00250 mainPower = 0; 00251 00252 }
Generated on Thu Jul 21 2022 21:05:54 by
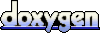