commit!
Embed:
(wiki syntax)
Show/hide line numbers
TSH.h
00001 #ifndef TSH_H 00002 #define TSH_H 00003 00004 #include "rtos.h" 00005 00006 //Thread Safe Hardware 00007 00008 class TSI2C : public I2C { 00009 public: 00010 00011 TSI2C( PinName sda, 00012 PinName scl, 00013 const char* name=NULL ) 00014 : I2C(sda, scl, name) { } 00015 00016 00017 int read( int address, 00018 char* data, 00019 int length, 00020 bool repeated = false ) { 00021 00022 rlock.lock(); 00023 int retval = I2C::read(address, data, length, repeated); 00024 rlock.unlock(); 00025 00026 return retval; 00027 } 00028 00029 int read(int ack) { 00030 rlock.lock(); 00031 int retval = I2C::read(ack); 00032 rlock.unlock(); 00033 00034 return retval; 00035 } 00036 00037 int write( int address, 00038 const char* data, 00039 int length, 00040 bool repeated = false ) { 00041 00042 wlock.lock(); 00043 int retval = I2C::write(address, data, length, repeated); 00044 wlock.unlock(); 00045 00046 return retval; 00047 } 00048 00049 int write(int data) { 00050 wlock.lock(); 00051 int retval = I2C::write(data); 00052 wlock.unlock(); 00053 00054 return retval; 00055 } 00056 00057 private: 00058 Mutex rlock; 00059 Mutex wlock; 00060 }; 00061 00062 #endif
Generated on Wed Jul 13 2022 17:39:34 by
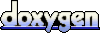