
Eurobot_2012_Secondary
Embed:
(wiki syntax)
Show/hide line numbers
RFSRF05.cpp
00001 00002 #include "RFSRF05.h" 00003 #include "mbed.h" 00004 #include "globals.h" 00005 00006 RFSRF05::RFSRF05(PinName trigger, 00007 PinName echo0, 00008 PinName echo1, 00009 PinName echo2, 00010 PinName echo3, 00011 PinName echo4, 00012 PinName echo5, 00013 PinName SDI, 00014 PinName SDO, 00015 PinName SCK, 00016 PinName NCS, 00017 PinName NIRQ) 00018 : _rf(SDI,SDO,SCK,NCS,NIRQ), 00019 _trigger(trigger), 00020 _echo0(echo0), 00021 _echo1(echo1), 00022 _echo2(echo2), 00023 _echo3(echo3), 00024 _echo4(echo4), 00025 _echo5(echo5) { 00026 00027 // initialises codes 00028 _code[0] = CODE0; 00029 _code[1] = CODE1; 00030 _code[2] = CODE2; 00031 00032 //set callback execute to true 00033 ValidPulse = false; 00034 00035 // Attach interrupts 00036 _echo0.rise(this, &RFSRF05::_rising); 00037 _echo0.fall(this, &RFSRF05::_falling); 00038 _echo1.fall(this, &RFSRF05::_falling); 00039 _echo2.fall(this, &RFSRF05::_falling); 00040 _echo3.fall(this, &RFSRF05::_falling); 00041 _echo4.fall(this, &RFSRF05::_falling); 00042 _echo5.fall(this, &RFSRF05::_falling); 00043 00044 00045 //init callabck function 00046 callbackfunc = NULL; 00047 callbackobj = NULL; 00048 mcallbackfunc = NULL; 00049 00050 // innitialises beacon counter 00051 _beacon_counter = 0; 00052 00053 //Interrupts every 50ms 00054 _ticker.attach(this, &RFSRF05::_startRange, 0.05); 00055 } 00056 00057 00058 void RFSRF05::_startRange() { 00059 00060 //printf("Srange\r\r"); 00061 00062 // increments counter 00063 _beacon_counter = (_beacon_counter + 1) % 3; 00064 00065 00066 // set flags 00067 ValidPulse = false; 00068 expValidPulse = true; 00069 00070 // writes code to RF port 00071 _rf.write(_code[_beacon_counter]); 00072 00073 00074 00075 // send a trigger pulse, 10uS long 00076 _trigger = 1; 00077 wait_us (10); 00078 _trigger = 0; 00079 00080 } 00081 00082 00083 // Clear and start the timer at the begining of the echo pulse 00084 void RFSRF05::_rising(void) { 00085 00086 _timer.reset(); 00087 _timer.start(); 00088 00089 //Set callback execute to ture 00090 if (expValidPulse) { 00091 ValidPulse = true; 00092 expValidPulse = false; 00093 } 00094 } 00095 00096 // Stop and read the timer at the end of the pulse 00097 void RFSRF05::_falling(void) { 00098 _timer.stop(); 00099 00100 if (ValidPulse) { 00101 //printf("Validpulse trig!\r\n"); 00102 ValidPulse = false; 00103 00104 //Calucate distance 00105 _dist[_beacon_counter] = _timer.read_us()/2.9 + 300; 00106 00107 if (callbackfunc) 00108 (*callbackfunc)(_beacon_counter, _dist[_beacon_counter]); 00109 00110 if (callbackobj && mcallbackfunc) 00111 (callbackobj->*mcallbackfunc)(_beacon_counter, _dist[_beacon_counter], sonarvariance); 00112 00113 } 00114 00115 } 00116 00117 float RFSRF05::read0() { 00118 // returns distance 00119 return (_dist[0]); 00120 } 00121 00122 float RFSRF05::read1() { 00123 // returns distance 00124 return (_dist[1]); 00125 } 00126 00127 float RFSRF05::read2() { 00128 // returns distance 00129 return (_dist[2]); 00130 } 00131 00132 float RFSRF05::read(unsigned int beaconnum) { 00133 // returns distance 00134 return (_dist[beaconnum]); 00135 } 00136 00137 //SRF05::operator float() { 00138 // return read(); 00139 //}
Generated on Sat Jul 16 2022 01:26:17 by
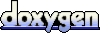