
Eurobot2012_Primary
Dependencies: mbed Eurobot_2012_Primary
RFSRF05.h
00001 00002 #ifndef MBED_RFSRF05_H 00003 #define MBED_RFSRF05_H 00004 00005 00006 00007 #include "mbed.h" 00008 #include "RF12B.h" 00009 #include "globals.h" 00010 00011 00012 #define CODE0 0x22 00013 #define CODE1 0x44 00014 #define CODE2 0x88 00015 00016 /* SAMPLE IMPLEMENTATION! 00017 RFSRF05 my_srf(p13,p21,p22,p23,p24,p25,p26,p5,p6,p7,p8,p9); 00018 00019 00020 void callbinmain(int num, float dist) { 00021 //Here is where you deal with your brand new reading ;D 00022 } 00023 00024 int main() { 00025 pc.printf("Hello World of RobotSonar!\r\n"); 00026 my_srf.callbackfunc = callbinmain; 00027 00028 while (1); 00029 } 00030 00031 */ 00032 00033 class DummyCT; 00034 00035 class RFSRF05 { 00036 public: 00037 00038 RFSRF05( 00039 PinName trigger, 00040 PinName echo0, 00041 PinName echo1, 00042 PinName echo2, 00043 PinName echo3, 00044 PinName echo4, 00045 PinName echo5, 00046 PinName SDI, 00047 PinName SDO, 00048 PinName SCK, 00049 PinName NCS, 00050 PinName NIRQ); 00051 00052 /** A non-blocking function that will return the last measurement 00053 * 00054 * @returns floating point representation of distance in mm 00055 */ 00056 float read0(); 00057 float read1(); 00058 float read2(); 00059 float read(unsigned int beaconnum); 00060 00061 00062 /** A assigns a callback function when a new reading is available **/ 00063 void (*callbackfunc)(int beaconnum, float distance); 00064 DummyCT* callbackobj; 00065 void (DummyCT::*mcallbackfunc)(int beaconnum, float distance, float variance); 00066 00067 //triggers a read 00068 #ifndef ROBOT_PRIMARY 00069 void startRange(unsigned char rx_code); 00070 #endif 00071 00072 //set codes 00073 void setCode(int code_index, unsigned char code); 00074 unsigned char codes[3]; 00075 00076 /** A short hand way of using the read function */ 00077 //operator float(); 00078 00079 private : 00080 RF12B _rf; 00081 DigitalOut _trigger; 00082 InterruptIn _echo0; 00083 InterruptIn _echo1; 00084 InterruptIn _echo2; 00085 InterruptIn _echo3; 00086 InterruptIn _echo4; 00087 InterruptIn _echo5; 00088 Timer _timer; 00089 Ticker _ticker; 00090 #ifdef ROBOT_PRIMARY 00091 void _startRange(void); 00092 #endif 00093 void _rising (void); 00094 void _falling (void); 00095 float _dist[3]; 00096 int _beacon_counter; 00097 bool ValidPulse; 00098 bool expValidPulse; 00099 00100 }; 00101 00102 #endif
Generated on Tue Jul 12 2022 21:49:58 by
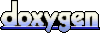