modified for VS1033
Dependents: 11U68_MP3Player with TFTLCD 11U68_MP3Player-with-TFTLCD Mp3_1
Fork of VS1033 by
VS1053.h
00001 // ==================================================== Dec 21 2013, kayeks == 00002 // VS1053.h 00003 // =========================================================================== 00004 // Just a simple library for VLSI's mp3/midi codec chip 00005 // - Minimal and simple implementation (and dirty too) 00006 00007 #ifndef KAYX_VS1053_H_ 00008 #define KAYX_VS1053_H_ 00009 #pragma O3 00010 //SCI_MODE register bits 00011 #define SM_DIFF 0x0001 00012 #define SM_SETTOZERO 0x0002 00013 #define SM_RESET 0x0004 00014 #define SM_OUTOFWAV 0x0008 00015 #define SM_PDOWN 0x0010 00016 #define SM_TESTS 0x0020 00017 #define SM_STREAM 0x0040 00018 #define SM_PLUSV 0x0080 00019 #define SM_DACT 0x0100 00020 #define SM_SDIORD 0x0200 00021 #define SM_SDISHARE 0x0400 00022 #define SM_SDINEW 0x0800 00023 #define SM_ADPCM 0x1000 00024 #define SM_ADPCM_HP 0x2000 00025 00026 #define SineWave_10k (0x1D) 00027 #define SineWave_1k (0xA8) 00028 00029 00030 /** Class VS1053. Drives VLSI's mp3/midi codec chip. */ 00031 class VS1053 { 00032 private: 00033 SPI spi; 00034 DigitalOut cs; 00035 DigitalOut dcs; 00036 DigitalIn dreq; 00037 DigitalOut rst; 00038 00039 public: 00040 static const uint8_t SCI_MODE = 0x00; 00041 static const uint8_t SCI_STATUS = 0x01; 00042 static const uint8_t SCI_BASS = 0x02; 00043 static const uint8_t SCI_CLOCKF = 0x03; 00044 static const uint8_t SCI_DECODE_TIME = 0x04; 00045 static const uint8_t SCI_AUDATA = 0x05; 00046 static const uint8_t SCI_WRAM = 0x06; 00047 static const uint8_t SCI_WRAMADDR = 0x07; 00048 static const uint8_t SCI_HDAT0 = 0x08; 00049 static const uint8_t SCI_HDAT1 = 0x09; 00050 static const uint8_t SCI_AIADDR = 0x0a; 00051 static const uint8_t SCI_VOL = 0x0b; 00052 static const uint8_t SCI_AICTRL0 = 0x0c; 00053 static const uint8_t SCI_AICTRL1 = 0x0d; 00054 static const uint8_t SCI_AICTRL2 = 0x0e; 00055 static const uint8_t SCI_AICTRL3 = 0x0f; 00056 00057 VS1053(PinName mosiPin, PinName misoPin, PinName sckPin, 00058 PinName csPin, PinName dcsPin, PinName dreqPin, PinName rstPin, 00059 uint32_t spiFrequency=7000000); 00060 ~VS1053(); 00061 void hardwareReset(); 00062 void sendDataByte(uint8_t data); 00063 size_t sendDataBlock(uint8_t* data, size_t length); 00064 bool checkDREQ(); 00065 void sci_init(); 00066 void sdi_init(); 00067 bool sendCancel(); 00068 bool stop(); 00069 void sine_test_activate(unsigned char wave); 00070 void sine_test_deactivate(); 00071 void VolControl(uint16_t vol); 00072 uint16_t readReg(uint8_t); 00073 00074 private: 00075 void writeReg(uint8_t, uint16_t); 00076 uint32_t useSpiFreq; 00077 void sci_en(); 00078 void sci_dis(); 00079 void sdi_en(); 00080 void sdi_dis(); 00081 }; 00082 00083 #endif
Generated on Sat Jul 23 2022 07:29:08 by
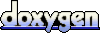