F303K8のタイマ6を動作させています。 LED Blink work on TIM6 of F303K8
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "stm32f3xx_hal_rcc.h" 00003 #include "cmsis_nvic.h" 00004 00005 DigitalOut myled(LED1); 00006 static void MX_TIM6_Init(void); 00007 static void MX_NVIC_Init(void); 00008 00009 void TIM6_DAC1_IRQHandler2(void); 00010 00011 TIM_HandleTypeDef htim6; 00012 void TIM6_DAC1_IRQHandler2(void) 00013 { 00014 static uint8_t flag = 0; 00015 myled = flag; 00016 flag = !flag; 00017 } 00018 00019 static void MX_NVIC_Init(void) 00020 { 00021 /* TIM6_DAC1_IRQn interrupt configuration */ 00022 HAL_NVIC_SetPriority(TIM6_DAC1_IRQn, 0, 0); 00023 HAL_NVIC_EnableIRQ(TIM6_DAC1_IRQn); 00024 } 00025 00026 /* TIM6 init function */ 00027 static void MX_TIM6_Init(void) 00028 { 00029 __TIM6_CLK_ENABLE(); 00030 htim6.Instance = TIM6; 00031 htim6.Init.Prescaler = 1; 00032 htim6.Init.CounterMode = TIM_COUNTERMODE_UP; 00033 htim6.Init.Period = 256; 00034 if (HAL_TIM_Base_Init(&htim6) != HAL_OK) { 00035 while(1) { 00036 } 00037 } 00038 HAL_TIM_Base_Start_IT(&htim6); 00039 } 00040 00041 int main() 00042 { 00043 MX_TIM6_Init(); 00044 NVIC_SetVector(TIM6_DAC1_IRQn, (uint32_t)&TIM6_DAC1_IRQHandler2); 00045 MX_NVIC_Init(); 00046 while(1) { 00047 } 00048 while(0) { 00049 myled = 1; // LED is ON 00050 wait(0.2); // 200 ms 00051 myled = 0; // LED is OFF 00052 wait(1.0); // 1 sec 00053 } 00054 }
Generated on Fri Jul 15 2022 16:34:50 by
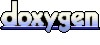