超乗合馬車電光掲示板用制御ソフトです。
Embed:
(wiki syntax)
Show/hide line numbers
15x16fontsLib.cpp
00001 #include "mbed.h" 00002 #include "displayCom.h" 00003 #include "15x16fontsLib.h" 00004 00005 SPI FontROM(p11, p12, p13); // mosi, miso, sclk 00006 DigitalOut FontROM_CS(p14); 00007 00008 static unsigned char matrixdata[32]; 00009 00010 static void read_font(unsigned short code) { 00011 unsigned char c1, c2, MSB,LSB; 00012 uint32_t Address, seq; 00013 00014 // SJIS to kuten code conversion 00015 c1 = (code>>8); 00016 c2 = (code & 0xFF); 00017 seq = (c1<=159 ? c1-129 : c1-193)*188 + (c2<=126 ? c2-64 : c2-65); 00018 MSB = seq / 94 + 1; 00019 LSB = seq % 94 + 1; 00020 Address = 0; 00021 00022 if( MSB >= 1 && MSB <= 15 && LSB >= 1 && LSB <= 94) 00023 Address =( (MSB - 1) * 94 + (LSB - 1))*32; 00024 else if(MSB >= 16 && MSB <= 47 && LSB >= 1 && LSB <= 94) 00025 Address =( (MSB - 16) * 94 + (LSB - 1))*32 + 0x0AA40L; 00026 else if(MSB >= 48 && MSB <= 84 && LSB >= 1 && LSB <= 94) 00027 Address = ((MSB - 48) * 94 + (LSB - 1))*32 + 0x21CDFL; 00028 else if(MSB == 85 && LSB >= 1 && LSB <= 94) 00029 Address = ((MSB - 85) * 94 + (LSB - 1))*32 + 0x3C4A0L; 00030 else if(MSB >= 88 && MSB <= 89 && LSB >= 1 && LSB <= 94) 00031 Address = ((MSB - 88) * 94 + (LSB - 1))*32 + 0x3D060L; 00032 00033 // if ASCII code 00034 int font_width; 00035 if(code >= 0x20 && code <= 0x7F) { 00036 Address = (code - 0x20)*16 + 255968; 00037 font_width = 8; 00038 } 00039 else { 00040 font_width = 16; 00041 } 00042 00043 // Deselect the device 00044 FontROM_CS = 1; 00045 00046 // Setup the spi for 8 bit data, high steady state clock 00047 FontROM.format(8,3); 00048 FontROM.frequency(1000000); 00049 00050 // Select the device by seting chip select low 00051 FontROM_CS = 0; 00052 FontROM.write(0x03); // Read data byte 00053 FontROM.write(Address>>16 & 0xff); 00054 FontROM.write(Address>>8 & 0xff); 00055 FontROM.write(Address & 0xff); 00056 00057 // Send a dummy byte to receive the contents of the WHOAMI register 00058 for(int i=0; i<(font_width*2); i++) 00059 { 00060 matrixdata[i]=FontROM.write(0x00); 00061 } 00062 00063 // Deselect the device 00064 FontROM_CS = 1; 00065 } 00066 00067 static void draw_kanji_15x16(int pos_x, int font_width) 00068 { 00069 for(int i=0; i<font_width; i++) 00070 { 00071 if( ((signed int)(15-i+pos_x) >= 0) && ((15-i+pos_x) <= (DISPLAY_XSIZE-1)) ) 00072 { 00073 ImageBuf[15-i+pos_x] = matrixdata[i]; 00074 ImageBuf[15-i+pos_x] |= matrixdata[i+font_width]<<8; 00075 } 00076 } 00077 } 00078 00079 void drawStr15x16(char *str ,unsigned int pos_x) 00080 { 00081 unsigned char f_SJISChar = 0; 00082 unsigned char c = 0; 00083 unsigned int SJISChar = 0; 00084 unsigned int CountChar = 0; 00085 00086 c = *str; 00087 while(c != '\0') 00088 { 00089 //2バイト文字の判定 00090 if( ((0x81 <= c && c <= 0x9f) || (0xe0 <= c && c <= 0xfc)) && f_SJISChar != 1 ) 00091 { 00092 SJISChar = c; 00093 f_SJISChar = 1; 00094 } 00095 else if(f_SJISChar == 1) 00096 { 00097 SJISChar = (SJISChar<<8) | c; 00098 f_SJISChar = 0; 00099 read_font(SJISChar); 00100 draw_kanji_15x16(pos_x-CountChar*8,16); 00101 CountChar+=2; 00102 } 00103 else //ASCII文字 00104 { 00105 SJISChar = c; 00106 f_SJISChar = 0; 00107 read_font(SJISChar); 00108 draw_kanji_15x16(pos_x-CountChar*8, 8); 00109 CountChar++; 00110 } 00111 str++; 00112 c = *str; 00113 } 00114 }
Generated on Sat Aug 6 2022 07:51:11 by
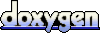