add LPC4337
Fork of FastIO by
Embed:
(wiki syntax)
Show/hide line numbers
FastIO.h
00001 #ifndef __FAST_IO_H 00002 #define __FAST_IO_H 00003 00004 #include "FastIO_LPC1768.h" 00005 #include "FastIO_LPC11UXX.h" 00006 #include "FastIO_LPC11U6X.h" 00007 #include "FastIO_LPC81X.h" 00008 #include "FastIO_KLXX.h" 00009 #include "FastIO_K20D50M_KPSDK.h" 00010 #include "FastIO_STM32F4.h" 00011 #include "FastIO_NUCLEO_F030.h" 00012 #include "FastIO_LPC11XX.h" 00013 #include "FastIO_EFM32.h" 00014 #include "FastIO_LPC43XX.h" 00015 00016 #ifndef INIT_PIN 00017 #warning Target is not supported by FastIO 00018 #warning Reverting to regular DigitalInOut 00019 #include "FastIO_Unsupported.h" 00020 #endif 00021 00022 #include "mbed.h" 00023 00024 /** 00025 * Faster alternative compared to regular DigitalInOut 00026 * 00027 * Except the constructor it is compatible with regular DigitalInOut. 00028 * Code is based on Igor Skochinsky's code (http://mbed.org/users/igorsk/code/FastIO/) 00029 */ 00030 template <PinName pin> class FastInOut 00031 { 00032 public: 00033 /** 00034 * Construct new FastInOut object 00035 * 00036 * @code 00037 * FastInOut<LED1> led1; 00038 * @endcode 00039 * 00040 * No initialization is done regarding input/output mode, 00041 * FastIn/FastOut can be used if that is required 00042 * 00043 * @param pin pin the FastOut object should be used for 00044 */ 00045 FastInOut() { 00046 INIT_PIN; 00047 } 00048 00049 ~FastInOut() { 00050 DESTROY_PIN; 00051 } 00052 00053 void write(int value) { 00054 if ( value ) 00055 WRITE_PIN_SET; 00056 else 00057 WRITE_PIN_CLR; 00058 } 00059 int read() { 00060 return READ_PIN; 00061 } 00062 00063 void mode(PinMode pull) { 00064 SET_MODE(pull); 00065 } 00066 00067 void output() { 00068 SET_DIR_OUTPUT; 00069 } 00070 00071 void input() { 00072 SET_DIR_INPUT; 00073 } 00074 00075 FastInOut& operator= (int value) { 00076 write(value); 00077 return *this; 00078 }; 00079 FastInOut& operator= (FastInOut& rhs) { 00080 return write(rhs.read()); 00081 }; 00082 operator int() { 00083 return read(); 00084 }; 00085 00086 protected: 00087 fastio_vars container; 00088 }; 00089 00090 /** 00091 * Faster alternative compared to regular DigitalOut 00092 * 00093 * Except the constructor it is compatible with regular DigitalOut. Aditionally all 00094 * functions from DigitalInOut are also available (only initialization is different) 00095 * Code is based on Igor Skochinsky's code (http://mbed.org/users/igorsk/code/FastIO/) 00096 */ 00097 template <PinName pin, int initial = 0> class FastOut : public FastInOut<pin> 00098 { 00099 public: 00100 /** 00101 * Construct new FastOut object 00102 * 00103 * @code 00104 * FastOut<LED1> led1; 00105 * @endcode 00106 * 00107 * @param pin pin the FastOut object should be used for 00108 * @param initial (optional) initial state of the pin after construction: default is 0 (low) 00109 */ 00110 FastOut() : FastInOut<pin>::FastInOut() { 00111 write(initial); 00112 SET_DIR_OUTPUT; 00113 } 00114 00115 FastOut& operator= (int value) { 00116 this->write(value); 00117 return *this; 00118 }; 00119 FastOut& operator= (FastOut& rhs) { 00120 return this->write(rhs.read()); 00121 }; 00122 operator int() { 00123 return this->read(); 00124 }; 00125 }; 00126 00127 /** 00128 * Faster alternative compared to regular DigitalIn 00129 * 00130 * Except the constructor it is compatible with regular DigitalIn. Aditionally all 00131 * functions from DigitalInOut are also available (only initialization is different) 00132 * Code is based on Igor Skochinsky's code (http://mbed.org/users/igorsk/code/FastIO/) 00133 */ 00134 template <PinName pin, PinMode pinmode = PullDefault> class FastIn : public FastInOut<pin> 00135 { 00136 public: 00137 /** 00138 * Construct new FastIn object 00139 * 00140 * @code 00141 * FastIn<LED1> led1; 00142 * @endcode 00143 * 00144 * @param pin pin the FastIn object should be used for 00145 * @param pinmode (optional) initial mode of the pin after construction: default is PullDefault 00146 */ 00147 FastIn() : FastInOut<pin>::FastInOut() { 00148 SET_MODE(pinmode); 00149 SET_DIR_INPUT; 00150 } 00151 00152 FastIn& operator= (int value) { 00153 this->write(value); 00154 return *this; 00155 }; 00156 FastIn& operator= (FastIn& rhs) { 00157 return this->write(rhs.read()); 00158 }; 00159 operator int() { 00160 return this->read(); 00161 }; 00162 }; 00163 00164 #endif
Generated on Tue Jul 12 2022 22:57:17 by
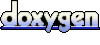