
aaa
Dependencies: mbed BNO055_fusion Adafruit_GFX ros_lib_kinetic
type.h
00001 #ifndef _TYPE_H_ 00002 #define _TYPE_H_ 00003 00004 #include <ros.h> 00005 #include <geometry_msgs/Point.h> 00006 00007 class Vec3f{ 00008 private: 00009 float x_; 00010 float y_; 00011 float z_; 00012 geometry_msgs::Point data_; 00013 00014 public: 00015 //Constructor 00016 Vec3f(float x = 0.0f, float y = 0.0f, float z = 0.0f) : x_(x), y_(y), z_(z){ 00017 //Create point msgs 00018 data_.x = x; 00019 data_.y = y; 00020 data_.z = z; 00021 } 00022 00023 //Setter 00024 void x(float x){ 00025 data_.x = x_ = x; 00026 } 00027 void y(float y){ 00028 data_.y = y_ = y; 00029 } 00030 void z(float z){ 00031 data_.z = z_ = z; 00032 } 00033 void angle(float angle){ 00034 z(angle); 00035 } 00036 00037 //Getter 00038 float x(){ 00039 return x_; 00040 } 00041 float y(){ 00042 return y_; 00043 } 00044 float z(){ 00045 return z_; 00046 } 00047 float angle(){ 00048 return z_; 00049 } 00050 00051 geometry_msgs::Point get_point_msgs(){ 00052 return data_; 00053 } 00054 00055 Vec3f operator + (Vec3f add){ 00056 return Vec3f(x_ + add.x(), y_ + add.y(), z_ + add.z()); 00057 } 00058 }; 00059 00060 class Vec4f : public Vec3f 00061 { 00062 private: 00063 float w_; 00064 00065 public: 00066 Vec4f(float x = 0.0f, float y = 0.0f, float z = 0.0f, float w = 0.0f) : Vec3f(x, y, z), w_(w){ 00067 00068 } 00069 00070 //Setter 00071 void w(float w){ 00072 w_ = w; 00073 } 00074 00075 //Getter 00076 float w(){ 00077 return w_; 00078 } 00079 }; 00080 00081 #endif
Generated on Mon Jul 18 2022 12:07:51 by
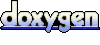