
a
Dependencies: BLE_API SDFileSystem mbed-rtos mbed nRF51822
Fork of SSmbed_BLE by
main.cpp
00001 #include "mbed.h" 00002 #include "ble/BLE.h" 00003 #include "ble/services/HeartRateService.h" 00004 #include "ble/services/BatteryService.h" 00005 #include "ble/services/DeviceInformationService.h" 00006 #include "rtos.h" 00007 #include <string.h> 00008 #include "SDFileSystem.h" 00009 #define N 100//addressを保存する個数 00010 #define M 6//addressとtimeを入れる 00011 #define GET_TIME 10000 00012 #define SEND_TIME 20000 00013 #define AA 0xAA 00014 #define BB 0xBB 00015 #define CC 0xCC 00016 #define DD 0xDD 00017 00018 DigitalOut myled3(LED3); 00019 00020 Semaphore one_slot(1); 00021 00022 Timer timer; 00023 BLE ble; 00024 char address[N][M]={};//保存する配列を初期化 00025 const GapScanningParams scanningParams; 00026 int wt;//threadの時間 00027 int wt1=0;//central mode の時間 00028 int ran; 00029 00030 SDFileSystem sd(p25, p28, p29, p21, "sd"); // the pinout on the mbed Cool Components workshop board 00031 FILE *fp; 00032 int counter=0,i,b,flag; 00033 00034 void onScanCallback(const Gap::AdvertisementCallbackParams_t *params){ 00035 00036 flag=0;//重複確認のためのフラグ 00037 for(i=0;i<counter;i++){ 00038 if(address[i][0] == params->peerAddr[0]){ 00039 flag=1; 00040 break; 00041 } 00042 } 00043 00044 if(flag==0){ 00045 for(i=0; i<6; i++){ 00046 address[counter][i]=params->peerAddr[i]; 00047 } 00048 time_t seconds = time(NULL); 00049 00050 00051 char buff[10]={}; 00052 sprintf(buff, "%d", seconds); 00053 strcat(address[counter],buff); 00054 00055 for(b=0; b<6 ; b++){ 00056 fprintf(fp,"%02x", address[counter][b]); 00057 printf("%02x", address[counter][b]); 00058 } 00059 fprintf(fp, ",%d\r\n",seconds); 00060 printf(" %d\r\n",seconds); 00061 counter++; 00062 00063 /* printf("DEV:"); 00064 for(b=0; b<6 ; b++)printf("%02x ", address[counter][b]); 00065 printf("%04d/%02d/%02d %02d:%02d:%02d \r\n",address[counter][6],address[counter][7],address[counter][8],address[counter][9],address[counter][10],address[counter][11]);*/ 00066 00067 00068 /*配列の中身をすべて表示 00069 for(a=0; a<counter; a++){ 00070 for(b=0; b<6 ; b++){ 00071 if(b==0)printf("DEV:"); 00072 printf("%02x ", address[a][b]); 00073 if(b==5){ 00074 printf("%04d/%02d/%02d %02d:%02d:%02d \r\n",address[a][6],address[a][7],address[a][8],address[a][9],address[a][10],address[a][11]); 00075 } 00076 } 00077 if(a==counter-1)printf("----------\n\r"); 00078 } 00079 */ 00080 } 00081 } 00082 00083 void test_thread(void const *name) { 00084 00085 while (true) { 00086 //Timer timer; 00087 one_slot.wait(); 00088 00089 if(!strcmp((const char*)name, "1")){ 00090 //printf("**startAdvertising thread**\n\r"); 00091 wt=1000; 00092 ble.gap().stopAdvertising(); 00093 ble.startScan(&onScanCallback); 00094 } 00095 //2 00096 if(!strcmp((const char*)name, "2")){ 00097 memset(address, 0, sizeof(address));//配列の初期化 00098 counter = 0; 00099 printf("**get**\n\r"); 00100 myled3 = 0; 00101 wt=0;//central modeの際はthreadの時間は0sec 00102 wt1 = GET_TIME ;//centralmode の時間はこっち 00103 fp = fopen("/sd/test.csv", "a"); 00104 00105 printf("%d seconds receive\n\r",wt1/1000); 00106 timer.start(); 00107 while(1){ 00108 ble.waitForEvent(); 00109 if(timer.read() > wt1/1000){ 00110 timer.stop(); 00111 timer.reset(); 00112 ble.stopScan(); 00113 fclose(fp); 00114 break; 00115 } 00116 } 00117 00118 } 00119 00120 //3 00121 if(!strcmp((const char*)name, "3")){ 00122 printf("**send**\n\r"); 00123 myled3 = 1; 00124 ran = rand() % 10; 00125 wt=SEND_TIME - ran*1000; 00126 printf("%d seconds sendin\n\r",wt/1000); 00127 ble.gap().startAdvertising();//BLEの送信 00128 } 00129 Thread::wait(wt); 00130 one_slot.release(); 00131 } 00132 } 00133 00134 // const static char DEVICE_NAME[] = "BLE1"; 00135 00136 void bleInitComplete(BLE::InitializationCompleteCallbackContext *params) 00137 { 00138 BLE &ble= params->ble; 00139 ble_error_t error = params->error; 00140 00141 if (error != BLE_ERROR_NONE) { 00142 return; 00143 } 00144 const uint8_t address1[] = {AA,AA,AA,AA,AA,AA}; 00145 ble.gap().setAddress(BLEProtocol::AddressType::PUBLIC, address1); 00146 /* ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); 00147 ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00148 ble.gap().setAdvertisingInterval(1000);*/ 00149 } 00150 00151 int main (void) { 00152 ble.init(bleInitComplete); 00153 ble.setScanParams(GapScanningParams::SCAN_INTERVAL_MAX,GapScanningParams::SCAN_WINDOW_MAX,0); 00154 00155 Thread t2(test_thread, (void *)"2"); 00156 Thread t3(test_thread, (void *)"3"); 00157 00158 test_thread((void *)"1"); 00159 }
Generated on Mon Jul 18 2022 18:24:19 by
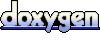