
only read CAN-bus data (not send any data to car)
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // reference : http://mbed.org/users/pangsk/programs/ecu_reader/ 00002 // Only read CAN-bus data, (not send any data) 00003 // v1.0 00004 00005 /* 00006 00007 mbed Can-Bus demo 00008 00009 This program is to demonstrate the CAN-bus capability of the mbed module. 00010 00011 http://www.skpang.co.uk/catalog/product_info.php?products_id=741 00012 00013 v1.0 July 2010 00014 00015 ******************************************************************************** 00016 00017 WARNING: Use at your own risk, sadly this software comes with no guarantees. 00018 This software is provided 'free' and in good faith, but the author does not 00019 accept liability for any damage arising from its use. 00020 00021 ******************************************************************************** 00022 00023 00024 */ 00025 00026 #include "mbed.h" 00027 #include "ecu_reader.h" 00028 #include "globals.h" 00029 #include "TextLCD.h" 00030 #include "SDFileSystem.h" 00031 00032 //GPS gps(p28, p27); 00033 TextLCD lcd(p24, p26, p27, p28, p29, p30); // rs, e, d0, d1, d2, d3, for starboard orange 00034 SDFileSystem sd(p5, p6, p7, p8, "sd"); //for starboard orange 00035 00036 /*DigitalIn click(p21); // Joystick inputs 00037 DigitalIn right(p22); 00038 DigitalIn down(p23); 00039 DigitalIn left(p24); 00040 DigitalIn up(p25);*/ 00041 00042 ecu_reader obdii(CANSPEED_500); //Create object and set CAN speed 00043 //void gps_demo(void); 00044 void sd_demo(void); 00045 //Timer CANTimer; 00046 Timer CANTimer2; 00047 00048 int main() { 00049 00050 // char buffer[20]; 00051 00052 lcd.locate(0,0); // Set LCD cursor position 00053 lcd.printf("CAN-Bus demo"); 00054 00055 lcd.locate(0,1); 00056 lcd.printf("www.skpang.co.uk"); 00057 pc.baud(460800); 00058 pc.printf("\n\rCAN-bus demo..."); 00059 00060 wait(3); 00061 lcd.cls(); 00062 00063 00064 /* while(1) // Wait until option is selected by the joystick 00065 { 00066 00067 if(down == 0) gps_demo(); 00068 if(left == 0) sd_demo(); 00069 if(up == 0) break; 00070 00071 }*/ 00072 00073 while(1) { // Main CAN loop 00074 led2 = 1; 00075 wait(0.1); 00076 led2 = 0; 00077 wait(0.1); 00078 00079 CANTimer2.reset(); 00080 CANTimer2.start(); 00081 pc.printf("CANTimer.start\n"); 00082 00083 while (CANTimer2.read_ms() < TIMEOUT) { 00084 // pc.printf("CANTimer.read_ms(): %dms ", CANTimer2.read_ms()); 00085 00086 // http://mbed.org/cookbook/OBDII-Can-Bus 00087 00088 if (can2.read(can_MsgRx)) { 00089 pc.printf("Message read\n\r"); 00090 00091 //print message id 00092 pc.printf("can_MsgRx.id: %x\n\r", can_MsgRx.id); 00093 00094 //print length of message 00095 pc.printf("Hex: can_MsgRx.len: %x\n\r", can_MsgRx.len); 00096 00097 //print data[2] 00098 //pc.printf("can_MsgRx.data[2]: %x\n\r", can_MsgRx.data[2]); 00099 00100 for (int i = 0; i < (int)can_MsgRx.len; i++) { 00101 pc.printf("can_MsgRx.data[%d]: %x\n\r", i, can_MsgRx.data[i]); 00102 } 00103 } 00104 } 00105 } 00106 00107 00108 00109 00110 00111 00112 /* if(obdii.request(ENGINE_RPM,buffer) == 1) // Get engine rpm and display on LCD 00113 { 00114 lcd.locate(0,0); 00115 lcd.printf(buffer); 00116 } 00117 00118 if(obdii.request(ENGINE_COOLANT_TEMP,buffer) == 1) 00119 { 00120 lcd.locate(9,0); 00121 lcd.printf(buffer); 00122 } 00123 00124 if(obdii.request(VEHICLE_SPEED,buffer) == 1) 00125 { 00126 lcd.locate(0,1); 00127 lcd.printf(buffer); 00128 } 00129 00130 if(obdii.request(THROTTLE,buffer) ==1 ) 00131 { 00132 lcd.locate(9,1); 00133 lcd.printf(buffer); 00134 } 00135 */ 00136 00137 } 00138 00139 /*void gps_demo(void) 00140 { 00141 lcd.cls(); 00142 lcd.printf("GPS demo"); 00143 lcd.locate(0,1); 00144 lcd.printf("Waiting for lock"); 00145 00146 wait(3); 00147 lcd.cls(); 00148 00149 while(1) 00150 { 00151 if(gps.sample()) { 00152 lcd.cls(); 00153 lcd.printf("Long:%f", gps.longitude); 00154 lcd.locate(0,1); 00155 lcd.printf("Lat:%f", gps.latitude); 00156 pc.printf("I'm at %f, %f\n", gps.longitude, gps.latitude); 00157 } else { 00158 pc.printf("Oh Dear! No lock :(\n"); 00159 lcd.cls(); 00160 lcd.printf("Waiting for lock"); 00161 00162 } 00163 } 00164 00165 }*/ 00166 00167 /* 00168 void sd_demo(void) 00169 { 00170 lcd.cls(); 00171 lcd.printf("SD demo"); 00172 wait(2); 00173 lcd.cls(); 00174 00175 FILE *fp = fopen("/sd/sdtest2.txt", "w"); 00176 if(fp == NULL) { 00177 lcd.cls(); 00178 lcd.printf("Could not open file for write\n"); 00179 } 00180 fprintf(fp, "Hello fun SD Card World! testing 1234"); 00181 fclose(fp); 00182 lcd.locate(0,1); 00183 lcd.printf("Writtern to SD card"); 00184 00185 while(1) 00186 { 00187 led2 = 1; 00188 wait(0.1); 00189 led2 = 0; 00190 wait(0.1); 00191 00192 } 00193 00194 } 00195 */
Generated on Fri Jul 15 2022 12:25:07 by
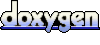