
Simple "hello world" style program for X-NUCLEO-IKS01A1 MEMS Inertial
Dependencies: BLE_API X_NUCLEO_IDB0XA1 X_NUCLEO_IKS01A1 mbed
Fork of HelloWorld_IKS01A1 by
stm32f4xx_usart.h
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f4xx_usart.h 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 30-September-2011 00007 * @brief This file contains all the functions prototypes for the USART 00008 * firmware library. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * THE PRESENT FIRMWARE WHICH IS FOR GUIDANCE ONLY AIMS AT PROVIDING CUSTOMERS 00013 * WITH CODING INFORMATION REGARDING THEIR PRODUCTS IN ORDER FOR THEM TO SAVE 00014 * TIME. AS A RESULT, STMICROELECTRONICS SHALL NOT BE HELD LIABLE FOR ANY 00015 * DIRECT, INDIRECT OR CONSEQUENTIAL DAMAGES WITH RESPECT TO ANY CLAIMS ARISING 00016 * FROM THE CONTENT OF SUCH FIRMWARE AND/OR THE USE MADE BY CUSTOMERS OF THE 00017 * CODING INFORMATION CONTAINED HEREIN IN CONNECTION WITH THEIR PRODUCTS. 00018 * 00019 * <h2><center>© COPYRIGHT 2011 STMicroelectronics</center></h2> 00020 ****************************************************************************** 00021 */ 00022 00023 /* Define to prevent recursive inclusion -------------------------------------*/ 00024 #ifndef __STM32F4xx_USART_H 00025 #define __STM32F4xx_USART_H 00026 00027 #ifdef __cplusplus 00028 extern "C" { 00029 #endif 00030 00031 /* Includes ------------------------------------------------------------------*/ 00032 #include "stm32f4xx.h" 00033 00034 /** @addtogroup STM32F4xx_StdPeriph_Driver 00035 * @{ 00036 */ 00037 00038 /** @addtogroup USART 00039 * @{ 00040 */ 00041 00042 /* Exported types ------------------------------------------------------------*/ 00043 00044 /** 00045 * @brief USART Init Structure definition 00046 */ 00047 00048 typedef struct 00049 { 00050 uint32_t USART_BaudRate; /*!< This member configures the USART communication baud rate. 00051 The baud rate is computed using the following formula: 00052 - IntegerDivider = ((PCLKx) / (8 * (OVR8+1) * (USART_InitStruct->USART_BaudRate))) 00053 - FractionalDivider = ((IntegerDivider - ((u32) IntegerDivider)) * 8 * (OVR8+1)) + 0.5 00054 Where OVR8 is the "oversampling by 8 mode" configuration bit in the CR1 register. */ 00055 00056 uint16_t USART_WordLength; /*!< Specifies the number of data bits transmitted or received in a frame. 00057 This parameter can be a value of @ref USART_Word_Length */ 00058 00059 uint16_t USART_StopBits; /*!< Specifies the number of stop bits transmitted. 00060 This parameter can be a value of @ref USART_Stop_Bits */ 00061 00062 uint16_t USART_Parity; /*!< Specifies the parity mode. 00063 This parameter can be a value of @ref USART_Parity 00064 @note When parity is enabled, the computed parity is inserted 00065 at the MSB position of the transmitted data (9th bit when 00066 the word length is set to 9 data bits; 8th bit when the 00067 word length is set to 8 data bits). */ 00068 00069 uint16_t USART_Mode; /*!< Specifies wether the Receive or Transmit mode is enabled or disabled. 00070 This parameter can be a value of @ref USART_Mode */ 00071 00072 uint16_t USART_HardwareFlowControl; /*!< Specifies wether the hardware flow control mode is enabled 00073 or disabled. 00074 This parameter can be a value of @ref USART_Hardware_Flow_Control */ 00075 } USART_InitTypeDef; 00076 00077 /** 00078 * @brief USART Clock Init Structure definition 00079 */ 00080 00081 typedef struct 00082 { 00083 00084 uint16_t USART_Clock; /*!< Specifies whether the USART clock is enabled or disabled. 00085 This parameter can be a value of @ref USART_Clock */ 00086 00087 uint16_t USART_CPOL; /*!< Specifies the steady state of the serial clock. 00088 This parameter can be a value of @ref USART_Clock_Polarity */ 00089 00090 uint16_t USART_CPHA; /*!< Specifies the clock transition on which the bit capture is made. 00091 This parameter can be a value of @ref USART_Clock_Phase */ 00092 00093 uint16_t USART_LastBit; /*!< Specifies whether the clock pulse corresponding to the last transmitted 00094 data bit (MSB) has to be output on the SCLK pin in synchronous mode. 00095 This parameter can be a value of @ref USART_Last_Bit */ 00096 } USART_ClockInitTypeDef; 00097 00098 /* Exported constants --------------------------------------------------------*/ 00099 00100 /** @defgroup USART_Exported_Constants 00101 * @{ 00102 */ 00103 00104 #define IS_USART_ALL_PERIPH(PERIPH) (((PERIPH) == USART1) || \ 00105 ((PERIPH) == USART2) || \ 00106 ((PERIPH) == USART3) || \ 00107 ((PERIPH) == UART4) || \ 00108 ((PERIPH) == UART5) || \ 00109 ((PERIPH) == USART6)) 00110 00111 #define IS_USART_1236_PERIPH(PERIPH) (((PERIPH) == USART1) || \ 00112 ((PERIPH) == USART2) || \ 00113 ((PERIPH) == USART3) || \ 00114 ((PERIPH) == USART6)) 00115 00116 /** @defgroup USART_Word_Length 00117 * @{ 00118 */ 00119 00120 #define USART_WordLength_8b ((uint16_t)0x0000) 00121 #define USART_WordLength_9b ((uint16_t)0x1000) 00122 00123 #define IS_USART_WORD_LENGTH(LENGTH) (((LENGTH) == USART_WordLength_8b) || \ 00124 ((LENGTH) == USART_WordLength_9b)) 00125 /** 00126 * @} 00127 */ 00128 00129 /** @defgroup USART_Stop_Bits 00130 * @{ 00131 */ 00132 00133 #define USART_StopBits_1 ((uint16_t)0x0000) 00134 #define USART_StopBits_0_5 ((uint16_t)0x1000) 00135 #define USART_StopBits_2 ((uint16_t)0x2000) 00136 #define USART_StopBits_1_5 ((uint16_t)0x3000) 00137 #define IS_USART_STOPBITS(STOPBITS) (((STOPBITS) == USART_StopBits_1) || \ 00138 ((STOPBITS) == USART_StopBits_0_5) || \ 00139 ((STOPBITS) == USART_StopBits_2) || \ 00140 ((STOPBITS) == USART_StopBits_1_5)) 00141 /** 00142 * @} 00143 */ 00144 00145 /** @defgroup USART_Parity 00146 * @{ 00147 */ 00148 00149 #define USART_Parity_No ((uint16_t)0x0000) 00150 #define USART_Parity_Even ((uint16_t)0x0400) 00151 #define USART_Parity_Odd ((uint16_t)0x0600) 00152 #define IS_USART_PARITY(PARITY) (((PARITY) == USART_Parity_No) || \ 00153 ((PARITY) == USART_Parity_Even) || \ 00154 ((PARITY) == USART_Parity_Odd)) 00155 /** 00156 * @} 00157 */ 00158 00159 /** @defgroup USART_Mode 00160 * @{ 00161 */ 00162 00163 #define USART_Mode_Rx ((uint16_t)0x0004) 00164 #define USART_Mode_Tx ((uint16_t)0x0008) 00165 #define IS_USART_MODE(MODE) ((((MODE) & (uint16_t)0xFFF3) == 0x00) && ((MODE) != (uint16_t)0x00)) 00166 /** 00167 * @} 00168 */ 00169 00170 /** @defgroup USART_Hardware_Flow_Control 00171 * @{ 00172 */ 00173 #define USART_HardwareFlowControl_None ((uint16_t)0x0000) 00174 #define USART_HardwareFlowControl_RTS ((uint16_t)0x0100) 00175 #define USART_HardwareFlowControl_CTS ((uint16_t)0x0200) 00176 #define USART_HardwareFlowControl_RTS_CTS ((uint16_t)0x0300) 00177 #define IS_USART_HARDWARE_FLOW_CONTROL(CONTROL)\ 00178 (((CONTROL) == USART_HardwareFlowControl_None) || \ 00179 ((CONTROL) == USART_HardwareFlowControl_RTS) || \ 00180 ((CONTROL) == USART_HardwareFlowControl_CTS) || \ 00181 ((CONTROL) == USART_HardwareFlowControl_RTS_CTS)) 00182 /** 00183 * @} 00184 */ 00185 00186 /** @defgroup USART_Clock 00187 * @{ 00188 */ 00189 #define USART_Clock_Disable ((uint16_t)0x0000) 00190 #define USART_Clock_Enable ((uint16_t)0x0800) 00191 #define IS_USART_CLOCK(CLOCK) (((CLOCK) == USART_Clock_Disable) || \ 00192 ((CLOCK) == USART_Clock_Enable)) 00193 /** 00194 * @} 00195 */ 00196 00197 /** @defgroup USART_Clock_Polarity 00198 * @{ 00199 */ 00200 00201 #define USART_CPOL_Low ((uint16_t)0x0000) 00202 #define USART_CPOL_High ((uint16_t)0x0400) 00203 #define IS_USART_CPOL(CPOL) (((CPOL) == USART_CPOL_Low) || ((CPOL) == USART_CPOL_High)) 00204 00205 /** 00206 * @} 00207 */ 00208 00209 /** @defgroup USART_Clock_Phase 00210 * @{ 00211 */ 00212 00213 #define USART_CPHA_1Edge ((uint16_t)0x0000) 00214 #define USART_CPHA_2Edge ((uint16_t)0x0200) 00215 #define IS_USART_CPHA(CPHA) (((CPHA) == USART_CPHA_1Edge) || ((CPHA) == USART_CPHA_2Edge)) 00216 00217 /** 00218 * @} 00219 */ 00220 00221 /** @defgroup USART_Last_Bit 00222 * @{ 00223 */ 00224 00225 #define USART_LastBit_Disable ((uint16_t)0x0000) 00226 #define USART_LastBit_Enable ((uint16_t)0x0100) 00227 #define IS_USART_LASTBIT(LASTBIT) (((LASTBIT) == USART_LastBit_Disable) || \ 00228 ((LASTBIT) == USART_LastBit_Enable)) 00229 /** 00230 * @} 00231 */ 00232 00233 /** @defgroup USART_Interrupt_definition 00234 * @{ 00235 */ 00236 00237 #define USART_IT_PE ((uint16_t)0x0028) 00238 #define USART_IT_TXE ((uint16_t)0x0727) 00239 #define USART_IT_TC ((uint16_t)0x0626) 00240 #define USART_IT_RXNE ((uint16_t)0x0525) 00241 #define USART_IT_ORE_RX ((uint16_t)0x0325) /* In case interrupt is generated if the RXNEIE bit is set */ 00242 #define USART_IT_IDLE ((uint16_t)0x0424) 00243 #define USART_IT_LBD ((uint16_t)0x0846) 00244 #define USART_IT_CTS ((uint16_t)0x096A) 00245 #define USART_IT_ERR ((uint16_t)0x0060) 00246 #define USART_IT_ORE_ER ((uint16_t)0x0360) /* In case interrupt is generated if the EIE bit is set */ 00247 #define USART_IT_NE ((uint16_t)0x0260) 00248 #define USART_IT_FE ((uint16_t)0x0160) 00249 00250 /** @defgroup USART_Legacy 00251 * @{ 00252 */ 00253 #define USART_IT_ORE USART_IT_ORE_ER 00254 /** 00255 * @} 00256 */ 00257 00258 #define IS_USART_CONFIG_IT(IT) (((IT) == USART_IT_PE) || ((IT) == USART_IT_TXE) || \ 00259 ((IT) == USART_IT_TC) || ((IT) == USART_IT_RXNE) || \ 00260 ((IT) == USART_IT_IDLE) || ((IT) == USART_IT_LBD) || \ 00261 ((IT) == USART_IT_CTS) || ((IT) == USART_IT_ERR)) 00262 #define IS_USART_GET_IT(IT) (((IT) == USART_IT_PE) || ((IT) == USART_IT_TXE) || \ 00263 ((IT) == USART_IT_TC) || ((IT) == USART_IT_RXNE) || \ 00264 ((IT) == USART_IT_IDLE) || ((IT) == USART_IT_LBD) || \ 00265 ((IT) == USART_IT_CTS) || ((IT) == USART_IT_ORE) || \ 00266 ((IT) == USART_IT_ORE_RX) || ((IT) == USART_IT_ORE_ER) || \ 00267 ((IT) == USART_IT_NE) || ((IT) == USART_IT_FE)) 00268 #define IS_USART_CLEAR_IT(IT) (((IT) == USART_IT_TC) || ((IT) == USART_IT_RXNE) || \ 00269 ((IT) == USART_IT_LBD) || ((IT) == USART_IT_CTS)) 00270 /** 00271 * @} 00272 */ 00273 00274 /** @defgroup USART_DMA_Requests 00275 * @{ 00276 */ 00277 00278 #define USART_DMAReq_Tx ((uint16_t)0x0080) 00279 #define USART_DMAReq_Rx ((uint16_t)0x0040) 00280 #define IS_USART_DMAREQ(DMAREQ) ((((DMAREQ) & (uint16_t)0xFF3F) == 0x00) && ((DMAREQ) != (uint16_t)0x00)) 00281 00282 /** 00283 * @} 00284 */ 00285 00286 /** @defgroup USART_WakeUp_methods 00287 * @{ 00288 */ 00289 00290 #define USART_WakeUp_IdleLine ((uint16_t)0x0000) 00291 #define USART_WakeUp_AddressMark ((uint16_t)0x0800) 00292 #define IS_USART_WAKEUP(WAKEUP) (((WAKEUP) == USART_WakeUp_IdleLine) || \ 00293 ((WAKEUP) == USART_WakeUp_AddressMark)) 00294 /** 00295 * @} 00296 */ 00297 00298 /** @defgroup USART_LIN_Break_Detection_Length 00299 * @{ 00300 */ 00301 00302 #define USART_LINBreakDetectLength_10b ((uint16_t)0x0000) 00303 #define USART_LINBreakDetectLength_11b ((uint16_t)0x0020) 00304 #define IS_USART_LIN_BREAK_DETECT_LENGTH(LENGTH) \ 00305 (((LENGTH) == USART_LINBreakDetectLength_10b) || \ 00306 ((LENGTH) == USART_LINBreakDetectLength_11b)) 00307 /** 00308 * @} 00309 */ 00310 00311 /** @defgroup USART_IrDA_Low_Power 00312 * @{ 00313 */ 00314 00315 #define USART_IrDAMode_LowPower ((uint16_t)0x0004) 00316 #define USART_IrDAMode_Normal ((uint16_t)0x0000) 00317 #define IS_USART_IRDA_MODE(MODE) (((MODE) == USART_IrDAMode_LowPower) || \ 00318 ((MODE) == USART_IrDAMode_Normal)) 00319 /** 00320 * @} 00321 */ 00322 00323 /** @defgroup USART_Flags 00324 * @{ 00325 */ 00326 00327 #define USART_FLAG_CTS ((uint16_t)0x0200) 00328 #define USART_FLAG_LBD ((uint16_t)0x0100) 00329 #define USART_FLAG_TXE ((uint16_t)0x0080) 00330 #define USART_FLAG_TC ((uint16_t)0x0040) 00331 #define USART_FLAG_RXNE ((uint16_t)0x0020) 00332 #define USART_FLAG_IDLE ((uint16_t)0x0010) 00333 #define USART_FLAG_ORE ((uint16_t)0x0008) 00334 #define USART_FLAG_NE ((uint16_t)0x0004) 00335 #define USART_FLAG_FE ((uint16_t)0x0002) 00336 #define USART_FLAG_PE ((uint16_t)0x0001) 00337 #define IS_USART_FLAG(FLAG) (((FLAG) == USART_FLAG_PE) || ((FLAG) == USART_FLAG_TXE) || \ 00338 ((FLAG) == USART_FLAG_TC) || ((FLAG) == USART_FLAG_RXNE) || \ 00339 ((FLAG) == USART_FLAG_IDLE) || ((FLAG) == USART_FLAG_LBD) || \ 00340 ((FLAG) == USART_FLAG_CTS) || ((FLAG) == USART_FLAG_ORE) || \ 00341 ((FLAG) == USART_FLAG_NE) || ((FLAG) == USART_FLAG_FE)) 00342 00343 #define IS_USART_CLEAR_FLAG(FLAG) ((((FLAG) & (uint16_t)0xFC9F) == 0x00) && ((FLAG) != (uint16_t)0x00)) 00344 00345 #define IS_USART_BAUDRATE(BAUDRATE) (((BAUDRATE) > 0) && ((BAUDRATE) < 7500001)) 00346 #define IS_USART_ADDRESS(ADDRESS) ((ADDRESS) <= 0xF) 00347 #define IS_USART_DATA(DATA) ((DATA) <= 0x1FF) 00348 00349 /** 00350 * @} 00351 */ 00352 00353 /** 00354 * @} 00355 */ 00356 00357 /* Exported macro ------------------------------------------------------------*/ 00358 /* Exported functions --------------------------------------------------------*/ 00359 00360 /* Function used to set the USART configuration to the default reset state ***/ 00361 void USART_DeInit(USART_TypeDef* USARTx); 00362 00363 /* Initialization and Configuration functions *********************************/ 00364 void USART_Init(USART_TypeDef* USARTx, USART_InitTypeDef* USART_InitStruct); 00365 void USART_StructInit(USART_InitTypeDef* USART_InitStruct); 00366 void USART_ClockInit(USART_TypeDef* USARTx, USART_ClockInitTypeDef* USART_ClockInitStruct); 00367 void USART_ClockStructInit(USART_ClockInitTypeDef* USART_ClockInitStruct); 00368 void USART_Cmd(USART_TypeDef* USARTx, FunctionalState NewState); 00369 void USART_SetPrescaler(USART_TypeDef* USARTx, uint8_t USART_Prescaler); 00370 void USART_OverSampling8Cmd(USART_TypeDef* USARTx, FunctionalState NewState); 00371 void USART_OneBitMethodCmd(USART_TypeDef* USARTx, FunctionalState NewState); 00372 00373 /* Data transfers functions ***************************************************/ 00374 void USART_SendData(USART_TypeDef* USARTx, uint16_t Data); 00375 uint16_t USART_ReceiveData(USART_TypeDef* USARTx); 00376 00377 /* Multi-Processor Communication functions ************************************/ 00378 void USART_SetAddress(USART_TypeDef* USARTx, uint8_t USART_Address); 00379 void USART_WakeUpConfig(USART_TypeDef* USARTx, uint16_t USART_WakeUp); 00380 void USART_ReceiverWakeUpCmd(USART_TypeDef* USARTx, FunctionalState NewState); 00381 00382 /* LIN mode functions *********************************************************/ 00383 void USART_LINBreakDetectLengthConfig(USART_TypeDef* USARTx, uint16_t USART_LINBreakDetectLength); 00384 void USART_LINCmd(USART_TypeDef* USARTx, FunctionalState NewState); 00385 void USART_SendBreak(USART_TypeDef* USARTx); 00386 00387 /* Half-duplex mode function **************************************************/ 00388 void USART_HalfDuplexCmd(USART_TypeDef* USARTx, FunctionalState NewState); 00389 00390 /* Smartcard mode functions ***************************************************/ 00391 void USART_SmartCardCmd(USART_TypeDef* USARTx, FunctionalState NewState); 00392 void USART_SmartCardNACKCmd(USART_TypeDef* USARTx, FunctionalState NewState); 00393 void USART_SetGuardTime(USART_TypeDef* USARTx, uint8_t USART_GuardTime); 00394 00395 /* IrDA mode functions ********************************************************/ 00396 void USART_IrDAConfig(USART_TypeDef* USARTx, uint16_t USART_IrDAMode); 00397 void USART_IrDACmd(USART_TypeDef* USARTx, FunctionalState NewState); 00398 00399 /* DMA transfers management functions *****************************************/ 00400 void USART_DMACmd(USART_TypeDef* USARTx, uint16_t USART_DMAReq, FunctionalState NewState); 00401 00402 /* Interrupts and flags management functions **********************************/ 00403 void USART_ITConfig(USART_TypeDef* USARTx, uint16_t USART_IT, FunctionalState NewState); 00404 FlagStatus USART_GetFlagStatus(USART_TypeDef* USARTx, uint16_t USART_FLAG); 00405 void USART_ClearFlag(USART_TypeDef* USARTx, uint16_t USART_FLAG); 00406 ITStatus USART_GetITStatus(USART_TypeDef* USARTx, uint16_t USART_IT); 00407 void USART_ClearITPendingBit(USART_TypeDef* USARTx, uint16_t USART_IT); 00408 00409 #ifdef __cplusplus 00410 } 00411 #endif 00412 00413 #endif /* __STM32F4xx_USART_H */ 00414 00415 /** 00416 * @} 00417 */ 00418 00419 /** 00420 * @} 00421 */ 00422 00423 /******************* (C) COPYRIGHT 2011 STMicroelectronics *****END OF FILE****/ 00424
Generated on Tue Jul 12 2022 19:53:13 by
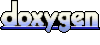