mbed I/F binding for mruby
Dependents: mruby_mbed_web mirb_mbed
object.h
00001 /* 00002 ** mruby/object.h - mruby object definition 00003 ** 00004 ** See Copyright Notice in mruby.h 00005 */ 00006 00007 #ifndef MRUBY_OBJECT_H 00008 #define MRUBY_OBJECT_H 00009 00010 #define MRB_OBJECT_HEADER \ 00011 enum mrb_vtype tt:8;\ 00012 uint32_t color:3;\ 00013 uint32_t flags:21;\ 00014 struct RClass *c;\ 00015 struct RBasic *gcnext 00016 00017 /* white: 011, black: 100, gray: 000 */ 00018 #define MRB_GC_GRAY 0 00019 #define MRB_GC_WHITE_A 1 00020 #define MRB_GC_WHITE_B (1 << 1) 00021 #define MRB_GC_BLACK (1 << 2) 00022 #define MRB_GC_WHITES (MRB_GC_WHITE_A | MRB_GC_WHITE_B) 00023 #define MRB_GC_COLOR_MASK 7 00024 00025 #define paint_gray(o) ((o)->color = MRB_GC_GRAY) 00026 #define paint_black(o) ((o)->color = MRB_GC_BLACK) 00027 #define paint_white(o) ((o)->color = MRB_GC_WHITES) 00028 #define paint_partial_white(s, o) ((o)->color = (s)->current_white_part) 00029 #define is_gray(o) ((o)->color == MRB_GC_GRAY) 00030 #define is_white(o) ((o)->color & MRB_GC_WHITES) 00031 #define is_black(o) ((o)->color & MRB_GC_BLACK) 00032 #define is_dead(s, o) (((o)->color & other_white_part(s) & MRB_GC_WHITES) || (o)->tt == MRB_TT_FREE) 00033 #define flip_white_part(s) ((s)->current_white_part = other_white_part(s)) 00034 #define other_white_part(s) ((s)->current_white_part ^ MRB_GC_WHITES) 00035 00036 struct RBasic { 00037 MRB_OBJECT_HEADER; 00038 }; 00039 #define mrb_basic_ptr(v) ((struct RBasic*)(mrb_ptr(v))) 00040 00041 struct RObject { 00042 MRB_OBJECT_HEADER; 00043 struct iv_tbl *iv; 00044 }; 00045 #define mrb_obj_ptr(v) ((struct RObject*)(mrb_ptr(v))) 00046 00047 #define mrb_immediate_p(x) (mrb_type(x) < MRB_TT_HAS_BASIC) 00048 #define mrb_special_const_p(x) mrb_immediate_p(x) 00049 00050 struct RFiber { 00051 MRB_OBJECT_HEADER; 00052 struct mrb_context *cxt; 00053 }; 00054 00055 #endif /* MRUBY_OBJECT_H */ 00056
Generated on Tue Jul 12 2022 18:00:34 by
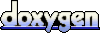