mbed I/F binding for mruby
Dependents: mruby_mbed_web mirb_mbed
hash-ext.c
00001 /* 00002 ** hash.c - Hash class 00003 ** 00004 ** See Copyright Notice in mruby.h 00005 */ 00006 00007 #include "mruby.h" 00008 #include "mruby/array.h" 00009 #include "mruby/hash.h" 00010 00011 /* 00012 * call-seq: 00013 * hsh.values_at(key, ...) -> array 00014 * 00015 * Return an array containing the values associated with the given keys. 00016 * Also see <code>Hash.select</code>. 00017 * 00018 * h = { "cat" => "feline", "dog" => "canine", "cow" => "bovine" } 00019 * h.values_at("cow", "cat") #=> ["bovine", "feline"] 00020 */ 00021 00022 static mrb_value 00023 hash_values_at(mrb_state *mrb, mrb_value hash) 00024 { 00025 mrb_value *argv, result; 00026 mrb_int argc, i; 00027 int ai; 00028 00029 mrb_get_args(mrb, "*", &argv, &argc); 00030 result = mrb_ary_new_capa(mrb, argc); 00031 ai = mrb_gc_arena_save(mrb); 00032 for (i = 0; i < argc; i++) { 00033 mrb_ary_push(mrb, result, mrb_hash_get(mrb, hash, argv[i])); 00034 mrb_gc_arena_restore(mrb, ai); 00035 } 00036 return result; 00037 } 00038 00039 void 00040 mrb_mruby_hash_ext_gem_init(mrb_state *mrb) 00041 { 00042 struct RClass *h; 00043 00044 h = mrb->hash_class; 00045 mrb_define_method(mrb, h, "values_at", hash_values_at, MRB_ARGS_ANY()); 00046 } 00047 00048 void 00049 mrb_mruby_hash_ext_gem_final(mrb_state *mrb) 00050 { 00051 } 00052
Generated on Tue Jul 12 2022 18:00:34 by
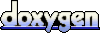