mbed I/F binding for mruby
Dependents: mruby_mbed_web mirb_mbed
class.c
00001 /* 00002 ** class.c - Class class 00003 ** 00004 ** See Copyright Notice in mruby.h 00005 */ 00006 00007 #include <ctype.h> 00008 #include <stdarg.h> 00009 #include "mruby.h" 00010 #include "mruby/array.h" 00011 #include "mruby/class.h" 00012 #include "mruby/numeric.h" 00013 #include "mruby/proc.h" 00014 #include "mruby/string.h" 00015 #include "mruby/variable.h" 00016 #include "mruby/error.h" 00017 #include "mruby/data.h" 00018 00019 KHASH_DEFINE(mt, mrb_sym, struct RProc*, TRUE, kh_int_hash_func, kh_int_hash_equal) 00020 00021 void 00022 mrb_gc_mark_mt(mrb_state *mrb, struct RClass *c) 00023 { 00024 khiter_t k; 00025 khash_t(mt) *h = c->mt; 00026 00027 if (!h) return; 00028 for (k = kh_begin(h); k != kh_end(h); k++) { 00029 if (kh_exist(h, k)) { 00030 struct RProc *m = kh_value(h, k); 00031 if (m) { 00032 mrb_gc_mark(mrb, (struct RBasic*)m); 00033 } 00034 } 00035 } 00036 } 00037 00038 size_t 00039 mrb_gc_mark_mt_size(mrb_state *mrb, struct RClass *c) 00040 { 00041 khash_t(mt) *h = c->mt; 00042 00043 if (!h) return 0; 00044 return kh_size(h); 00045 } 00046 00047 void 00048 mrb_gc_free_mt(mrb_state *mrb, struct RClass *c) 00049 { 00050 kh_destroy(mt, mrb, c->mt); 00051 } 00052 00053 static void 00054 name_class(mrb_state *mrb, struct RClass *c, mrb_sym name) 00055 { 00056 mrb_obj_iv_set(mrb, (struct RObject*)c, 00057 mrb_intern_lit(mrb, "__classid__"), mrb_symbol_value(name)); 00058 } 00059 00060 static void 00061 setup_class(mrb_state *mrb, struct RClass *outer, struct RClass *c, mrb_sym id) 00062 { 00063 name_class(mrb, c, id); 00064 mrb_obj_iv_set(mrb, (struct RObject*)outer, id, mrb_obj_value(c)); 00065 if (outer != mrb->object_class) { 00066 mrb_obj_iv_set(mrb, (struct RObject*)c, mrb_intern_lit(mrb, "__outer__"), 00067 mrb_obj_value(outer)); 00068 } 00069 } 00070 00071 #define make_metaclass(mrb, c) prepare_singleton_class((mrb), (struct RBasic*)(c)) 00072 00073 static void 00074 prepare_singleton_class(mrb_state *mrb, struct RBasic *o) 00075 { 00076 struct RClass *sc, *c; 00077 00078 if (o->c->tt == MRB_TT_SCLASS) return; 00079 sc = (struct RClass*)mrb_obj_alloc(mrb, MRB_TT_SCLASS, mrb->class_class); 00080 sc->mt = 0; 00081 sc->iv = 0; 00082 if (o->tt == MRB_TT_CLASS) { 00083 c = (struct RClass*)o; 00084 if (!c->super) { 00085 sc->super = mrb->class_class; 00086 } 00087 else { 00088 sc->super = c->super->c; 00089 } 00090 } 00091 else if (o->tt == MRB_TT_SCLASS) { 00092 c = (struct RClass*)o; 00093 while (c->super->tt == MRB_TT_ICLASS) 00094 c = c->super; 00095 make_metaclass(mrb, c->super); 00096 sc->super = c->super->c; 00097 } 00098 else { 00099 sc->super = o->c; 00100 } 00101 o->c = sc; 00102 mrb_field_write_barrier(mrb, (struct RBasic*)o, (struct RBasic*)sc); 00103 mrb_field_write_barrier(mrb, (struct RBasic*)sc, (struct RBasic*)o); 00104 mrb_obj_iv_set(mrb, (struct RObject*)sc, mrb_intern_lit(mrb, "__attached__"), mrb_obj_value(o)); 00105 } 00106 00107 static struct RClass * 00108 class_from_sym(mrb_state *mrb, struct RClass *klass, mrb_sym id) 00109 { 00110 mrb_value c = mrb_const_get(mrb, mrb_obj_value(klass), id); 00111 00112 mrb_check_type(mrb, c, MRB_TT_CLASS); 00113 return mrb_class_ptr(c); 00114 } 00115 00116 static struct RClass * 00117 module_from_sym(mrb_state *mrb, struct RClass *klass, mrb_sym id) 00118 { 00119 mrb_value c = mrb_const_get(mrb, mrb_obj_value(klass), id); 00120 00121 mrb_check_type(mrb, c, MRB_TT_MODULE); 00122 return mrb_class_ptr(c); 00123 } 00124 00125 MRB_API struct RClass* 00126 mrb_class_outer_module(mrb_state *mrb, struct RClass *c) 00127 { 00128 mrb_value outer; 00129 00130 outer = mrb_obj_iv_get(mrb, (struct RObject*)c, mrb_intern_lit(mrb, "__outer__")); 00131 if (mrb_nil_p(outer)) return NULL; 00132 return mrb_class_ptr(outer); 00133 } 00134 00135 static struct RClass* 00136 define_module(mrb_state *mrb, mrb_sym name, struct RClass *outer) 00137 { 00138 struct RClass *m; 00139 00140 if (mrb_const_defined_at(mrb, mrb_obj_value(outer), name)) { 00141 return module_from_sym(mrb, outer, name); 00142 } 00143 m = mrb_module_new(mrb); 00144 setup_class(mrb, outer, m, name); 00145 00146 return m; 00147 } 00148 00149 MRB_API struct RClass* 00150 mrb_define_module_id(mrb_state *mrb, mrb_sym name) 00151 { 00152 return define_module(mrb, name, mrb->object_class); 00153 } 00154 00155 MRB_API struct RClass* 00156 mrb_define_module(mrb_state *mrb, const char *name) 00157 { 00158 return define_module(mrb, mrb_intern_cstr(mrb, name), mrb->object_class); 00159 } 00160 00161 MRB_API struct RClass* 00162 mrb_vm_define_module(mrb_state *mrb, mrb_value outer, mrb_sym id) 00163 { 00164 return define_module(mrb, id, mrb_class_ptr(outer)); 00165 } 00166 00167 MRB_API struct RClass* 00168 mrb_define_module_under(mrb_state *mrb, struct RClass *outer, const char *name) 00169 { 00170 mrb_sym id = mrb_intern_cstr(mrb, name); 00171 struct RClass * c = define_module(mrb, id, outer); 00172 00173 setup_class(mrb, outer, c, id); 00174 return c; 00175 } 00176 00177 static struct RClass* 00178 define_class(mrb_state *mrb, mrb_sym name, struct RClass *super, struct RClass *outer) 00179 { 00180 struct RClass * c; 00181 00182 if (mrb_const_defined_at(mrb, mrb_obj_value(outer), name)) { 00183 c = class_from_sym(mrb, outer, name); 00184 if (super && mrb_class_real(c->super) != super) { 00185 mrb_raisef(mrb, E_TYPE_ERROR, "superclass mismatch for Class %S (%S not %S)", 00186 mrb_sym2str(mrb, name), 00187 mrb_obj_value(c->super), mrb_obj_value(super)); 00188 } 00189 return c; 00190 } 00191 00192 c = mrb_class_new(mrb, super); 00193 setup_class(mrb, outer, c, name); 00194 00195 return c; 00196 } 00197 00198 MRB_API struct RClass* 00199 mrb_define_class_id(mrb_state *mrb, mrb_sym name, struct RClass *super) 00200 { 00201 if (!super) { 00202 mrb_warn(mrb, "no super class for `%S', Object assumed", mrb_sym2str(mrb, name)); 00203 } 00204 return define_class(mrb, name, super, mrb->object_class); 00205 } 00206 00207 MRB_API struct RClass* 00208 mrb_define_class(mrb_state *mrb, const char *name, struct RClass *super) 00209 { 00210 return mrb_define_class_id(mrb, mrb_intern_cstr(mrb, name), super); 00211 } 00212 00213 static void 00214 mrb_class_inherited(mrb_state *mrb, struct RClass *super, struct RClass *klass) 00215 { 00216 if (!super) 00217 super = mrb->object_class; 00218 mrb_funcall(mrb, mrb_obj_value(super), "inherited", 1, mrb_obj_value(klass)); 00219 } 00220 00221 MRB_API struct RClass* 00222 mrb_vm_define_class(mrb_state *mrb, mrb_value outer, mrb_value super, mrb_sym id) 00223 { 00224 struct RClass *s; 00225 struct RClass *c; 00226 00227 if (!mrb_nil_p(super)) { 00228 if (mrb_type(super) != MRB_TT_CLASS) { 00229 mrb_raisef(mrb, E_TYPE_ERROR, "superclass must be a Class (%S given)", super); 00230 } 00231 s = mrb_class_ptr(super); 00232 } 00233 else { 00234 s = 0; 00235 } 00236 switch (mrb_type(outer)) { 00237 case MRB_TT_CLASS: 00238 case MRB_TT_SCLASS: 00239 case MRB_TT_MODULE: 00240 break; 00241 default: 00242 mrb_raisef(mrb, E_TYPE_ERROR, "%S is not a class/module", outer); 00243 break; 00244 } 00245 c = define_class(mrb, id, s, mrb_class_ptr(outer)); 00246 mrb_class_inherited(mrb, mrb_class_real(c->super), c); 00247 00248 return c; 00249 } 00250 00251 MRB_API mrb_bool 00252 mrb_class_defined(mrb_state *mrb, const char *name) 00253 { 00254 mrb_value sym = mrb_check_intern_cstr(mrb, name); 00255 if (mrb_nil_p(sym)) { 00256 return FALSE; 00257 } 00258 return mrb_const_defined(mrb, mrb_obj_value(mrb->object_class), mrb_symbol(sym)); 00259 } 00260 00261 MRB_API struct RClass * 00262 mrb_class_get_under(mrb_state *mrb, struct RClass *outer, const char *name) 00263 { 00264 return class_from_sym(mrb, outer, mrb_intern_cstr(mrb, name)); 00265 } 00266 00267 MRB_API struct RClass * 00268 mrb_class_get(mrb_state *mrb, const char *name) 00269 { 00270 return mrb_class_get_under(mrb, mrb->object_class, name); 00271 } 00272 00273 MRB_API struct RClass * 00274 mrb_module_get_under(mrb_state *mrb, struct RClass *outer, const char *name) 00275 { 00276 return module_from_sym(mrb, outer, mrb_intern_cstr(mrb, name)); 00277 } 00278 00279 MRB_API struct RClass * 00280 mrb_module_get(mrb_state *mrb, const char *name) 00281 { 00282 return mrb_module_get_under(mrb, mrb->object_class, name); 00283 } 00284 00285 /*! 00286 * Defines a class under the namespace of \a outer. 00287 * \param outer a class which contains the new class. 00288 * \param id name of the new class 00289 * \param super a class from which the new class will derive. 00290 * NULL means \c Object class. 00291 * \return the created class 00292 * \throw TypeError if the constant name \a name is already taken but 00293 * the constant is not a \c Class. 00294 * \throw NameError if the class is already defined but the class can not 00295 * be reopened because its superclass is not \a super. 00296 * \post top-level constant named \a name refers the returned class. 00297 * 00298 * \note if a class named \a name is already defined and its superclass is 00299 * \a super, the function just returns the defined class. 00300 */ 00301 MRB_API struct RClass * 00302 mrb_define_class_under(mrb_state *mrb, struct RClass *outer, const char *name, struct RClass *super) 00303 { 00304 mrb_sym id = mrb_intern_cstr(mrb, name); 00305 struct RClass * c; 00306 00307 #if 0 00308 if (!super) { 00309 mrb_warn(mrb, "no super class for `%S::%S', Object assumed", 00310 mrb_obj_value(outer), mrb_sym2str(mrb, id)); 00311 } 00312 #endif 00313 c = define_class(mrb, id, super, outer); 00314 setup_class(mrb, outer, c, id); 00315 return c; 00316 } 00317 00318 MRB_API void 00319 mrb_define_method_raw(mrb_state *mrb, struct RClass *c, mrb_sym mid, struct RProc *p) 00320 { 00321 khash_t(mt) *h = c->mt; 00322 khiter_t k; 00323 00324 if (!h) h = c->mt = kh_init(mt, mrb); 00325 k = kh_put(mt, mrb, h, mid); 00326 kh_value(h, k) = p; 00327 if (p) { 00328 mrb_field_write_barrier(mrb, (struct RBasic *)c, (struct RBasic *)p); 00329 } 00330 } 00331 00332 MRB_API void 00333 mrb_define_method_id(mrb_state *mrb, struct RClass *c, mrb_sym mid, mrb_func_t func, mrb_aspec aspec) 00334 { 00335 struct RProc *p; 00336 int ai = mrb_gc_arena_save(mrb); 00337 00338 p = mrb_proc_new_cfunc(mrb, func); 00339 p->target_class = c; 00340 mrb_define_method_raw(mrb, c, mid, p); 00341 mrb_gc_arena_restore(mrb, ai); 00342 } 00343 00344 MRB_API void 00345 mrb_define_method(mrb_state *mrb, struct RClass *c, const char *name, mrb_func_t func, mrb_aspec aspec) 00346 { 00347 mrb_define_method_id(mrb, c, mrb_intern_cstr(mrb, name), func, aspec); 00348 } 00349 00350 MRB_API void 00351 mrb_define_method_vm(mrb_state *mrb, struct RClass *c, mrb_sym name, mrb_value body) 00352 { 00353 khash_t(mt) *h = c->mt; 00354 khiter_t k; 00355 struct RProc *p; 00356 00357 if (!h) h = c->mt = kh_init(mt, mrb); 00358 k = kh_put(mt, mrb, h, name); 00359 p = mrb_proc_ptr(body); 00360 kh_value(h, k) = p; 00361 if (p) { 00362 mrb_field_write_barrier(mrb, (struct RBasic *)c, (struct RBasic *)p); 00363 } 00364 } 00365 00366 /* a function to raise NotImplementedError with current method name */ 00367 MRB_API void 00368 mrb_notimplement(mrb_state *mrb) 00369 { 00370 const char *str; 00371 mrb_int len; 00372 mrb_callinfo *ci = mrb->c->ci; 00373 00374 if (ci->mid) { 00375 str = mrb_sym2name_len(mrb, ci->mid, &len); 00376 mrb_raisef(mrb, E_NOTIMP_ERROR, 00377 "%S() function is unimplemented on this machine", 00378 mrb_str_new_static(mrb, str, (size_t)len)); 00379 } 00380 } 00381 00382 /* a function to be replacement of unimplemented method */ 00383 MRB_API mrb_value 00384 mrb_notimplement_m(mrb_state *mrb, mrb_value self) 00385 { 00386 mrb_notimplement(mrb); 00387 /* not reached */ 00388 return mrb_nil_value(); 00389 } 00390 00391 static mrb_value 00392 check_type(mrb_state *mrb, mrb_value val, enum mrb_vtype t, const char *c, const char *m) 00393 { 00394 mrb_value tmp; 00395 00396 tmp = mrb_check_convert_type(mrb, val, t, c, m); 00397 if (mrb_nil_p(tmp)) { 00398 mrb_raisef(mrb, E_TYPE_ERROR, "expected %S", mrb_str_new_cstr(mrb, c)); 00399 } 00400 return tmp; 00401 } 00402 00403 static mrb_value 00404 to_str(mrb_state *mrb, mrb_value val) 00405 { 00406 return check_type(mrb, val, MRB_TT_STRING, "String", "to_str"); 00407 } 00408 00409 static mrb_value 00410 to_ary(mrb_state *mrb, mrb_value val) 00411 { 00412 return check_type(mrb, val, MRB_TT_ARRAY, "Array", "to_ary"); 00413 } 00414 00415 static mrb_value 00416 to_hash(mrb_state *mrb, mrb_value val) 00417 { 00418 return check_type(mrb, val, MRB_TT_HASH, "Hash", "to_hash"); 00419 } 00420 00421 static mrb_sym 00422 to_sym(mrb_state *mrb, mrb_value ss) 00423 { 00424 if (mrb_type(ss) == MRB_TT_SYMBOL) { 00425 return mrb_symbol(ss); 00426 } 00427 else if (mrb_string_p(ss)) { 00428 return mrb_intern_str(mrb, to_str(mrb, ss)); 00429 } 00430 else { 00431 mrb_value obj = mrb_funcall(mrb, ss, "inspect", 0); 00432 mrb_raisef(mrb, E_TYPE_ERROR, "%S is not a symbol", obj); 00433 } 00434 } 00435 00436 /* 00437 retrieve arguments from mrb_state. 00438 00439 mrb_get_args(mrb, format, ...) 00440 00441 returns number of arguments parsed. 00442 00443 format specifiers: 00444 00445 string mruby type C type note 00446 ---------------------------------------------------------------------------------------------- 00447 o: Object [mrb_value] 00448 C: class/module [mrb_value] 00449 S: String [mrb_value] 00450 A: Array [mrb_value] 00451 H: Hash [mrb_value] 00452 s: String [char*,mrb_int] Receive two arguments. 00453 z: String [char*] NUL terminated string. 00454 a: Array [mrb_value*,mrb_int] Receive two arguments. 00455 f: Float [mrb_float] 00456 i: Integer [mrb_int] 00457 b: Boolean [mrb_bool] 00458 n: Symbol [mrb_sym] 00459 d: Data [void*,mrb_data_type const] 2nd argument will be used to check data type so it won't be modified 00460 &: Block [mrb_value] 00461 *: rest argument [mrb_value*,mrb_int] Receive the rest of the arguments as an array. 00462 |: optional Next argument of '|' and later are optional. 00463 ?: optional given [mrb_bool] true if preceding argument (optional) is given. 00464 */ 00465 MRB_API mrb_int 00466 mrb_get_args(mrb_state *mrb, const char *format, ...) 00467 { 00468 char c; 00469 int i = 0; 00470 mrb_value *sp = mrb->c->stack + 1; 00471 va_list ap; 00472 int argc = mrb->c->ci->argc; 00473 mrb_bool opt = FALSE; 00474 mrb_bool given = TRUE; 00475 00476 va_start(ap, format); 00477 if (argc < 0) { 00478 struct RArray *a = mrb_ary_ptr(mrb->c->stack[1]); 00479 00480 argc = a->len; 00481 sp = a->ptr; 00482 } 00483 while ((c = *format++)) { 00484 switch (c) { 00485 case '|': case '*': case '&': case '?': 00486 break; 00487 default: 00488 if (argc <= i) { 00489 if (opt) { 00490 given = FALSE; 00491 } 00492 else { 00493 mrb_raise(mrb, E_ARGUMENT_ERROR, "wrong number of arguments"); 00494 } 00495 } 00496 break; 00497 } 00498 00499 switch (c) { 00500 case 'o': 00501 { 00502 mrb_value *p; 00503 00504 p = va_arg(ap, mrb_value*); 00505 if (i < argc) { 00506 *p = *sp++; 00507 i++; 00508 } 00509 } 00510 break; 00511 case 'C': 00512 { 00513 mrb_value *p; 00514 00515 p = va_arg(ap, mrb_value*); 00516 if (i < argc) { 00517 mrb_value ss; 00518 00519 ss = *sp++; 00520 switch (mrb_type(ss)) { 00521 case MRB_TT_CLASS: 00522 case MRB_TT_MODULE: 00523 case MRB_TT_SCLASS: 00524 break; 00525 default: 00526 mrb_raisef(mrb, E_TYPE_ERROR, "%S is not class/module", ss); 00527 break; 00528 } 00529 *p = ss; 00530 i++; 00531 } 00532 } 00533 break; 00534 case 'S': 00535 { 00536 mrb_value *p; 00537 00538 p = va_arg(ap, mrb_value*); 00539 if (i < argc) { 00540 *p = to_str(mrb, *sp++); 00541 i++; 00542 } 00543 } 00544 break; 00545 case 'A': 00546 { 00547 mrb_value *p; 00548 00549 p = va_arg(ap, mrb_value*); 00550 if (i < argc) { 00551 *p = to_ary(mrb, *sp++); 00552 i++; 00553 } 00554 } 00555 break; 00556 case 'H': 00557 { 00558 mrb_value *p; 00559 00560 p = va_arg(ap, mrb_value*); 00561 if (i < argc) { 00562 *p = to_hash(mrb, *sp++); 00563 i++; 00564 } 00565 } 00566 break; 00567 case 's': 00568 { 00569 mrb_value ss; 00570 char **ps = 0; 00571 mrb_int *pl = 0; 00572 00573 ps = va_arg(ap, char**); 00574 pl = va_arg(ap, mrb_int*); 00575 if (i < argc) { 00576 ss = to_str(mrb, *sp++); 00577 *ps = RSTRING_PTR(ss); 00578 *pl = RSTRING_LEN(ss); 00579 i++; 00580 } 00581 } 00582 break; 00583 case 'z': 00584 { 00585 mrb_value ss; 00586 const char **ps; 00587 00588 ps = va_arg(ap, const char**); 00589 if (i < argc) { 00590 ss = to_str(mrb, *sp++); 00591 *ps = mrb_string_value_cstr(mrb, &ss); 00592 i++; 00593 } 00594 } 00595 break; 00596 case 'a': 00597 { 00598 mrb_value aa; 00599 struct RArray *a; 00600 mrb_value **pb; 00601 mrb_int *pl; 00602 00603 pb = va_arg(ap, mrb_value**); 00604 pl = va_arg(ap, mrb_int*); 00605 if (i < argc) { 00606 aa = to_ary(mrb, *sp++); 00607 a = mrb_ary_ptr(aa); 00608 *pb = a->ptr; 00609 *pl = a->len; 00610 i++; 00611 } 00612 } 00613 break; 00614 case 'f': 00615 { 00616 mrb_float *p; 00617 00618 p = va_arg(ap, mrb_float*); 00619 if (i < argc) { 00620 *p = mrb_to_flo(mrb, *sp); 00621 sp++; 00622 i++; 00623 } 00624 } 00625 break; 00626 case 'i': 00627 { 00628 mrb_int *p; 00629 00630 p = va_arg(ap, mrb_int*); 00631 if (i < argc) { 00632 switch (mrb_type(*sp)) { 00633 case MRB_TT_FIXNUM: 00634 *p = mrb_fixnum(*sp); 00635 break; 00636 case MRB_TT_FLOAT: 00637 { 00638 mrb_float f = mrb_float(*sp); 00639 00640 if (!FIXABLE(f)) { 00641 mrb_raise(mrb, E_RANGE_ERROR, "float too big for int"); 00642 } 00643 *p = (mrb_int)f; 00644 } 00645 break; 00646 case MRB_TT_STRING: 00647 mrb_raise(mrb, E_TYPE_ERROR, "no implicit conversion of String into Integer"); 00648 break; 00649 default: 00650 *p = mrb_fixnum(mrb_Integer(mrb, *sp)); 00651 break; 00652 } 00653 sp++; 00654 i++; 00655 } 00656 } 00657 break; 00658 case 'b': 00659 { 00660 mrb_bool *boolp = va_arg(ap, mrb_bool*); 00661 00662 if (i < argc) { 00663 mrb_value b = *sp++; 00664 *boolp = mrb_test(b); 00665 i++; 00666 } 00667 } 00668 break; 00669 case 'n': 00670 { 00671 mrb_sym *symp; 00672 00673 symp = va_arg(ap, mrb_sym*); 00674 if (i < argc) { 00675 mrb_value ss; 00676 00677 ss = *sp++; 00678 *symp = to_sym(mrb, ss); 00679 i++; 00680 } 00681 } 00682 break; 00683 case 'd': 00684 { 00685 void** datap; 00686 struct mrb_data_type const* type; 00687 00688 datap = va_arg(ap, void**); 00689 type = va_arg(ap, struct mrb_data_type const*); 00690 if (i < argc) { 00691 *datap = mrb_data_get_ptr(mrb, *sp++, type); 00692 ++i; 00693 } 00694 } 00695 break; 00696 00697 case '&': 00698 { 00699 mrb_value *p, *bp; 00700 00701 p = va_arg(ap, mrb_value*); 00702 if (mrb->c->ci->argc < 0) { 00703 bp = mrb->c->stack + 2; 00704 } 00705 else { 00706 bp = mrb->c->stack + mrb->c->ci->argc + 1; 00707 } 00708 *p = *bp; 00709 } 00710 break; 00711 case '|': 00712 opt = TRUE; 00713 break; 00714 case '?': 00715 { 00716 mrb_bool *p; 00717 00718 p = va_arg(ap, mrb_bool*); 00719 *p = given; 00720 } 00721 break; 00722 00723 case '*': 00724 { 00725 mrb_value **var; 00726 mrb_int *pl; 00727 00728 var = va_arg(ap, mrb_value**); 00729 pl = va_arg(ap, mrb_int*); 00730 if (argc > i) { 00731 *pl = argc-i; 00732 if (*pl > 0) { 00733 *var = sp; 00734 } 00735 i = argc; 00736 sp += *pl; 00737 } 00738 else { 00739 *pl = 0; 00740 *var = NULL; 00741 } 00742 } 00743 break; 00744 default: 00745 mrb_raisef(mrb, E_ARGUMENT_ERROR, "invalid argument specifier %S", mrb_str_new(mrb, &c, 1)); 00746 break; 00747 } 00748 } 00749 if (!c && argc > i) { 00750 mrb_raise(mrb, E_ARGUMENT_ERROR, "wrong number of arguments"); 00751 } 00752 va_end(ap); 00753 return i; 00754 } 00755 00756 static struct RClass* 00757 boot_defclass(mrb_state *mrb, struct RClass *super) 00758 { 00759 struct RClass *c; 00760 00761 c = (struct RClass*)mrb_obj_alloc(mrb, MRB_TT_CLASS, mrb->class_class); 00762 if (super) { 00763 c->super = super; 00764 mrb_field_write_barrier(mrb, (struct RBasic*)c, (struct RBasic*)super); 00765 } 00766 else { 00767 c->super = mrb->object_class; 00768 } 00769 c->mt = kh_init(mt, mrb); 00770 return c; 00771 } 00772 00773 MRB_API void 00774 mrb_include_module(mrb_state *mrb, struct RClass *c, struct RClass *m) 00775 { 00776 struct RClass *ins_pos; 00777 00778 ins_pos = c; 00779 while (m) { 00780 struct RClass *p = c, *ic; 00781 int superclass_seen = 0; 00782 00783 if (c->mt && c->mt == m->mt) { 00784 mrb_raise(mrb, E_ARGUMENT_ERROR, "cyclic include detected"); 00785 } 00786 while (p) { 00787 if (c != p && p->tt == MRB_TT_CLASS) { 00788 superclass_seen = 1; 00789 } 00790 else if (p->mt == m->mt) { 00791 if (p->tt == MRB_TT_ICLASS && !superclass_seen) { 00792 ins_pos = p; 00793 } 00794 goto skip; 00795 } 00796 p = p->super; 00797 } 00798 ic = (struct RClass*)mrb_obj_alloc(mrb, MRB_TT_ICLASS, mrb->class_class); 00799 if (m->tt == MRB_TT_ICLASS) { 00800 ic->c = m->c; 00801 } 00802 else { 00803 ic->c = m; 00804 } 00805 ic->mt = m->mt; 00806 ic->iv = m->iv; 00807 ic->super = ins_pos->super; 00808 ins_pos->super = ic; 00809 mrb_field_write_barrier(mrb, (struct RBasic*)ins_pos, (struct RBasic*)ic); 00810 ins_pos = ic; 00811 skip: 00812 m = m->super; 00813 } 00814 } 00815 00816 static mrb_value 00817 mrb_mod_append_features(mrb_state *mrb, mrb_value mod) 00818 { 00819 mrb_value klass; 00820 00821 mrb_check_type(mrb, mod, MRB_TT_MODULE); 00822 mrb_get_args(mrb, "C", &klass); 00823 mrb_include_module(mrb, mrb_class_ptr(klass), mrb_class_ptr(mod)); 00824 return mod; 00825 } 00826 00827 static mrb_value 00828 mrb_mod_include(mrb_state *mrb, mrb_value klass) 00829 { 00830 mrb_value *argv; 00831 mrb_int argc, i; 00832 00833 mrb_get_args(mrb, "*", &argv, &argc); 00834 for (i=0; i<argc; i++) { 00835 mrb_check_type(mrb, argv[i], MRB_TT_MODULE); 00836 } 00837 while (argc--) { 00838 mrb_funcall(mrb, argv[argc], "append_features", 1, klass); 00839 mrb_funcall(mrb, argv[argc], "included", 1, klass); 00840 } 00841 00842 return klass; 00843 } 00844 00845 /* 15.2.2.4.28 */ 00846 /* 00847 * call-seq: 00848 * mod.include?(module) -> true or false 00849 * 00850 * Returns <code>true</code> if <i>module</i> is included in 00851 * <i>mod</i> or one of <i>mod</i>'s ancestors. 00852 * 00853 * module A 00854 * end 00855 * class B 00856 * include A 00857 * end 00858 * class C < B 00859 * end 00860 * B.include?(A) #=> true 00861 * C.include?(A) #=> true 00862 * A.include?(A) #=> false 00863 */ 00864 static mrb_value 00865 mrb_mod_include_p(mrb_state *mrb, mrb_value mod) 00866 { 00867 mrb_value mod2; 00868 struct RClass *c = mrb_class_ptr(mod); 00869 00870 mrb_get_args(mrb, "C", &mod2); 00871 mrb_check_type(mrb, mod2, MRB_TT_MODULE); 00872 00873 while (c) { 00874 if (c->tt == MRB_TT_ICLASS) { 00875 if (c->c == mrb_class_ptr(mod2)) return mrb_true_value(); 00876 } 00877 c = c->super; 00878 } 00879 return mrb_false_value(); 00880 } 00881 00882 static mrb_value 00883 mrb_mod_ancestors(mrb_state *mrb, mrb_value self) 00884 { 00885 mrb_value result; 00886 struct RClass *c = mrb_class_ptr(self); 00887 00888 result = mrb_ary_new(mrb); 00889 mrb_ary_push(mrb, result, mrb_obj_value(c)); 00890 c = c->super; 00891 while (c) { 00892 if (c->tt == MRB_TT_ICLASS) { 00893 mrb_ary_push(mrb, result, mrb_obj_value(c->c)); 00894 } 00895 else if (c->tt != MRB_TT_SCLASS) { 00896 mrb_ary_push(mrb, result, mrb_obj_value(c)); 00897 } 00898 c = c->super; 00899 } 00900 00901 return result; 00902 } 00903 00904 static mrb_value 00905 mrb_mod_extend_object(mrb_state *mrb, mrb_value mod) 00906 { 00907 mrb_value obj; 00908 00909 mrb_check_type(mrb, mod, MRB_TT_MODULE); 00910 mrb_get_args(mrb, "o", &obj); 00911 mrb_include_module(mrb, mrb_class_ptr(mrb_singleton_class(mrb, obj)), mrb_class_ptr(mod)); 00912 return mod; 00913 } 00914 00915 static mrb_value 00916 mrb_mod_included_modules(mrb_state *mrb, mrb_value self) 00917 { 00918 mrb_value result; 00919 struct RClass *c = mrb_class_ptr(self); 00920 00921 result = mrb_ary_new(mrb); 00922 while (c) { 00923 if (c->tt == MRB_TT_ICLASS) { 00924 mrb_ary_push(mrb, result, mrb_obj_value(c->c)); 00925 } 00926 c = c->super; 00927 } 00928 00929 return result; 00930 } 00931 00932 static mrb_value 00933 mrb_mod_initialize(mrb_state *mrb, mrb_value mod) 00934 { 00935 mrb_value b; 00936 00937 mrb_get_args(mrb, "&", &b); 00938 if (!mrb_nil_p(b)) { 00939 mrb_yield_with_class(mrb, b, 1, &mod, mod, mrb_class_ptr(mod)); 00940 } 00941 return mod; 00942 } 00943 00944 mrb_value mrb_class_instance_method_list(mrb_state*, mrb_bool, struct RClass*, int); 00945 00946 /* 15.2.2.4.33 */ 00947 /* 00948 * call-seq: 00949 * mod.instance_methods(include_super=true) -> array 00950 * 00951 * Returns an array containing the names of the public and protected instance 00952 * methods in the receiver. For a module, these are the public and protected methods; 00953 * for a class, they are the instance (not singleton) methods. With no 00954 * argument, or with an argument that is <code>false</code>, the 00955 * instance methods in <i>mod</i> are returned, otherwise the methods 00956 * in <i>mod</i> and <i>mod</i>'s superclasses are returned. 00957 * 00958 * module A 00959 * def method1() end 00960 * end 00961 * class B 00962 * def method2() end 00963 * end 00964 * class C < B 00965 * def method3() end 00966 * end 00967 * 00968 * A.instance_methods #=> [:method1] 00969 * B.instance_methods(false) #=> [:method2] 00970 * C.instance_methods(false) #=> [:method3] 00971 * C.instance_methods(true).length #=> 43 00972 */ 00973 00974 static mrb_value 00975 mrb_mod_instance_methods(mrb_state *mrb, mrb_value mod) 00976 { 00977 struct RClass *c = mrb_class_ptr(mod); 00978 mrb_bool recur = TRUE; 00979 mrb_get_args(mrb, "|b", &recur); 00980 return mrb_class_instance_method_list(mrb, recur, c, 0); 00981 } 00982 00983 /* implementation of module_eval/class_eval */ 00984 mrb_value mrb_mod_module_eval(mrb_state*, mrb_value); 00985 00986 static mrb_value 00987 mrb_mod_dummy_visibility(mrb_state *mrb, mrb_value mod) 00988 { 00989 return mod; 00990 } 00991 00992 MRB_API mrb_value 00993 mrb_singleton_class(mrb_state *mrb, mrb_value v) 00994 { 00995 struct RBasic *obj; 00996 00997 switch (mrb_type(v)) { 00998 case MRB_TT_FALSE: 00999 if (mrb_nil_p(v)) 01000 return mrb_obj_value(mrb->nil_class); 01001 return mrb_obj_value(mrb->false_class); 01002 case MRB_TT_TRUE: 01003 return mrb_obj_value(mrb->true_class); 01004 case MRB_TT_CPTR: 01005 return mrb_obj_value(mrb->object_class); 01006 case MRB_TT_SYMBOL: 01007 case MRB_TT_FIXNUM: 01008 case MRB_TT_FLOAT: 01009 mrb_raise(mrb, E_TYPE_ERROR, "can't define singleton"); 01010 return mrb_nil_value(); /* not reached */ 01011 default: 01012 break; 01013 } 01014 obj = mrb_basic_ptr(v); 01015 prepare_singleton_class(mrb, obj); 01016 if (mrb->c && mrb->c->ci && mrb->c->ci->target_class) { 01017 mrb_obj_iv_set(mrb, (struct RObject*)obj->c, mrb_intern_lit(mrb, "__outer__"), 01018 mrb_obj_value(mrb->c->ci->target_class)); 01019 } 01020 return mrb_obj_value(obj->c); 01021 } 01022 01023 MRB_API void 01024 mrb_define_singleton_method(mrb_state *mrb, struct RObject *o, const char *name, mrb_func_t func, mrb_aspec aspec) 01025 { 01026 prepare_singleton_class(mrb, (struct RBasic*)o); 01027 mrb_define_method_id(mrb, o->c, mrb_intern_cstr(mrb, name), func, aspec); 01028 } 01029 01030 MRB_API void 01031 mrb_define_class_method(mrb_state *mrb, struct RClass *c, const char *name, mrb_func_t func, mrb_aspec aspec) 01032 { 01033 mrb_define_singleton_method(mrb, (struct RObject*)c, name, func, aspec); 01034 } 01035 01036 MRB_API void 01037 mrb_define_module_function(mrb_state *mrb, struct RClass *c, const char *name, mrb_func_t func, mrb_aspec aspec) 01038 { 01039 mrb_define_class_method(mrb, c, name, func, aspec); 01040 mrb_define_method(mrb, c, name, func, aspec); 01041 } 01042 01043 MRB_API struct RProc* 01044 mrb_method_search_vm(mrb_state *mrb, struct RClass **cp, mrb_sym mid) 01045 { 01046 khiter_t k; 01047 struct RProc *m; 01048 struct RClass *c = *cp; 01049 01050 while (c) { 01051 khash_t(mt) *h = c->mt; 01052 01053 if (h) { 01054 k = kh_get(mt, mrb, h, mid); 01055 if (k != kh_end(h)) { 01056 m = kh_value(h, k); 01057 if (!m) break; 01058 *cp = c; 01059 return m; 01060 } 01061 } 01062 c = c->super; 01063 } 01064 return NULL; /* no method */ 01065 } 01066 01067 MRB_API struct RProc* 01068 mrb_method_search(mrb_state *mrb, struct RClass* c, mrb_sym mid) 01069 { 01070 struct RProc *m; 01071 01072 m = mrb_method_search_vm(mrb, &c, mid); 01073 if (!m) { 01074 mrb_value inspect = mrb_funcall(mrb, mrb_obj_value(c), "inspect", 0); 01075 if (RSTRING_LEN(inspect) > 64) { 01076 inspect = mrb_any_to_s(mrb, mrb_obj_value(c)); 01077 } 01078 mrb_name_error(mrb, mid, "undefined method '%S' for class %S", 01079 mrb_sym2str(mrb, mid), inspect); 01080 } 01081 return m; 01082 } 01083 01084 static mrb_value 01085 attr_reader(mrb_state *mrb, mrb_value obj) 01086 { 01087 mrb_value name = mrb_proc_cfunc_env_get(mrb, 0); 01088 return mrb_iv_get(mrb, obj, to_sym(mrb, name)); 01089 } 01090 01091 static mrb_value 01092 mrb_mod_attr_reader(mrb_state *mrb, mrb_value mod) 01093 { 01094 struct RClass *c = mrb_class_ptr(mod); 01095 mrb_value *argv; 01096 mrb_int argc, i; 01097 int ai; 01098 01099 mrb_get_args(mrb, "*", &argv, &argc); 01100 ai = mrb_gc_arena_save(mrb); 01101 for (i=0; i<argc; i++) { 01102 mrb_value name, str; 01103 mrb_sym method, sym; 01104 01105 method = to_sym(mrb, argv[i]); 01106 name = mrb_sym2str(mrb, method); 01107 str = mrb_str_buf_new(mrb, RSTRING_LEN(name)+1); 01108 mrb_str_cat_lit(mrb, str, "@"); 01109 mrb_str_cat_str(mrb, str, name); 01110 sym = mrb_intern_str(mrb, str); 01111 mrb_iv_check(mrb, sym); 01112 name = mrb_symbol_value(sym); 01113 mrb_define_method_raw(mrb, c, method, 01114 mrb_proc_new_cfunc_with_env(mrb, attr_reader, 1, &name)); 01115 mrb_gc_arena_restore(mrb, ai); 01116 } 01117 return mrb_nil_value(); 01118 } 01119 01120 static mrb_value 01121 attr_writer(mrb_state *mrb, mrb_value obj) 01122 { 01123 mrb_value name = mrb_proc_cfunc_env_get(mrb, 0); 01124 mrb_value val; 01125 01126 mrb_get_args(mrb, "o", &val); 01127 mrb_iv_set(mrb, obj, to_sym(mrb, name), val); 01128 return val; 01129 } 01130 01131 static mrb_value 01132 mrb_mod_attr_writer(mrb_state *mrb, mrb_value mod) 01133 { 01134 struct RClass *c = mrb_class_ptr(mod); 01135 mrb_value *argv; 01136 mrb_int argc, i; 01137 int ai; 01138 01139 mrb_get_args(mrb, "*", &argv, &argc); 01140 ai = mrb_gc_arena_save(mrb); 01141 for (i=0; i<argc; i++) { 01142 mrb_value name, str, attr; 01143 mrb_sym method, sym; 01144 01145 method = to_sym(mrb, argv[i]); 01146 01147 /* prepare iv name (@name) */ 01148 name = mrb_sym2str(mrb, method); 01149 str = mrb_str_buf_new(mrb, RSTRING_LEN(name)+1); 01150 mrb_str_cat_lit(mrb, str, "@"); 01151 mrb_str_cat_str(mrb, str, name); 01152 sym = mrb_intern_str(mrb, str); 01153 mrb_iv_check(mrb, sym); 01154 attr = mrb_symbol_value(sym); 01155 01156 /* prepare method name (name=) */ 01157 str = mrb_str_buf_new(mrb, RSTRING_LEN(str)); 01158 mrb_str_cat_str(mrb, str, name); 01159 mrb_str_cat_lit(mrb, str, "="); 01160 method = mrb_intern_str(mrb, str); 01161 01162 mrb_define_method_raw(mrb, c, method, 01163 mrb_proc_new_cfunc_with_env(mrb, attr_writer, 1, &attr)); 01164 mrb_gc_arena_restore(mrb, ai); 01165 } 01166 return mrb_nil_value(); 01167 } 01168 01169 static mrb_value 01170 mrb_instance_alloc(mrb_state *mrb, mrb_value cv) 01171 { 01172 struct RClass *c = mrb_class_ptr(cv); 01173 struct RObject *o; 01174 enum mrb_vtype ttype = MRB_INSTANCE_TT(c); 01175 01176 if (c->tt == MRB_TT_SCLASS) 01177 mrb_raise(mrb, E_TYPE_ERROR, "can't create instance of singleton class"); 01178 01179 if (ttype == 0) ttype = MRB_TT_OBJECT; 01180 o = (struct RObject*)mrb_obj_alloc(mrb, ttype, c); 01181 return mrb_obj_value(o); 01182 } 01183 01184 /* 01185 * call-seq: 01186 * class.new(args, ...) -> obj 01187 * 01188 * Calls <code>allocate</code> to create a new object of 01189 * <i>class</i>'s class, then invokes that object's 01190 * <code>initialize</code> method, passing it <i>args</i>. 01191 * This is the method that ends up getting called whenever 01192 * an object is constructed using .new. 01193 * 01194 */ 01195 01196 MRB_API mrb_value 01197 mrb_instance_new(mrb_state *mrb, mrb_value cv) 01198 { 01199 mrb_value obj, blk; 01200 mrb_value *argv; 01201 mrb_int argc; 01202 01203 mrb_get_args(mrb, "*&", &argv, &argc, &blk); 01204 obj = mrb_instance_alloc(mrb, cv); 01205 mrb_funcall_with_block(mrb, obj, mrb_intern_lit(mrb, "initialize"), argc, argv, blk); 01206 01207 return obj; 01208 } 01209 01210 MRB_API mrb_value 01211 mrb_obj_new(mrb_state *mrb, struct RClass *c, mrb_int argc, const mrb_value *argv) 01212 { 01213 mrb_value obj; 01214 01215 obj = mrb_instance_alloc(mrb, mrb_obj_value(c)); 01216 mrb_funcall_argv(mrb, obj, mrb_intern_lit(mrb, "initialize"), argc, argv); 01217 01218 return obj; 01219 } 01220 01221 static mrb_value 01222 mrb_class_initialize(mrb_state *mrb, mrb_value c) 01223 { 01224 mrb_value a, b; 01225 01226 mrb_get_args(mrb, "|C&", &a, &b); 01227 if (!mrb_nil_p(b)) { 01228 mrb_yield_with_class(mrb, b, 1, &c, c, mrb_class_ptr(c)); 01229 } 01230 return c; 01231 } 01232 01233 static mrb_value 01234 mrb_class_new_class(mrb_state *mrb, mrb_value cv) 01235 { 01236 mrb_int n; 01237 mrb_value super, blk; 01238 mrb_value new_class; 01239 01240 n = mrb_get_args(mrb, "|C&", &super, &blk); 01241 if (n == 0) { 01242 super = mrb_obj_value(mrb->object_class); 01243 } 01244 new_class = mrb_obj_value(mrb_class_new(mrb, mrb_class_ptr(super))); 01245 mrb_funcall_with_block(mrb, new_class, mrb_intern_lit(mrb, "initialize"), n, &super, blk); 01246 mrb_class_inherited(mrb, mrb_class_ptr(super), mrb_class_ptr(new_class)); 01247 return new_class; 01248 } 01249 01250 static mrb_value 01251 mrb_class_superclass(mrb_state *mrb, mrb_value klass) 01252 { 01253 struct RClass *c; 01254 01255 c = mrb_class_ptr(klass); 01256 c = c->super; 01257 while (c && c->tt == MRB_TT_ICLASS) { 01258 c = c->super; 01259 } 01260 if (!c) return mrb_nil_value(); 01261 return mrb_obj_value(c); 01262 } 01263 01264 static mrb_value 01265 mrb_bob_init(mrb_state *mrb, mrb_value cv) 01266 { 01267 return mrb_nil_value(); 01268 } 01269 01270 static mrb_value 01271 mrb_bob_not(mrb_state *mrb, mrb_value cv) 01272 { 01273 return mrb_bool_value(!mrb_test(cv)); 01274 } 01275 01276 /* 15.3.1.3.30 */ 01277 /* 01278 * call-seq: 01279 * obj.method_missing(symbol [, *args] ) -> result 01280 * 01281 * Invoked by Ruby when <i>obj</i> is sent a message it cannot handle. 01282 * <i>symbol</i> is the symbol for the method called, and <i>args</i> 01283 * are any arguments that were passed to it. By default, the interpreter 01284 * raises an error when this method is called. However, it is possible 01285 * to override the method to provide more dynamic behavior. 01286 * If it is decided that a particular method should not be handled, then 01287 * <i>super</i> should be called, so that ancestors can pick up the 01288 * missing method. 01289 * The example below creates 01290 * a class <code>Roman</code>, which responds to methods with names 01291 * consisting of roman numerals, returning the corresponding integer 01292 * values. 01293 * 01294 * class Roman 01295 * def romanToInt(str) 01296 * # ... 01297 * end 01298 * def method_missing(methId) 01299 * str = methId.id2name 01300 * romanToInt(str) 01301 * end 01302 * end 01303 * 01304 * r = Roman.new 01305 * r.iv #=> 4 01306 * r.xxiii #=> 23 01307 * r.mm #=> 2000 01308 */ 01309 static mrb_value 01310 mrb_bob_missing(mrb_state *mrb, mrb_value mod) 01311 { 01312 mrb_sym name; 01313 mrb_value *a; 01314 mrb_int alen; 01315 mrb_sym inspect; 01316 mrb_value repr; 01317 01318 mrb_get_args(mrb, "n*", &name, &a, &alen); 01319 01320 inspect = mrb_intern_lit(mrb, "inspect"); 01321 if (mrb->c->ci > mrb->c->cibase && mrb->c->ci[-1].mid == inspect) { 01322 /* method missing in inspect; avoid recursion */ 01323 repr = mrb_any_to_s(mrb, mod); 01324 } 01325 else if (mrb_respond_to(mrb, mod, inspect) && mrb->c->ci - mrb->c->cibase < 64) { 01326 repr = mrb_funcall_argv(mrb, mod, inspect, 0, 0); 01327 if (RSTRING_LEN(repr) > 64) { 01328 repr = mrb_any_to_s(mrb, mod); 01329 } 01330 } 01331 else { 01332 repr = mrb_any_to_s(mrb, mod); 01333 } 01334 01335 mrb_no_method_error(mrb, name, alen, a, "undefined method '%S' for %S", mrb_sym2str(mrb, name), repr); 01336 /* not reached */ 01337 return mrb_nil_value(); 01338 } 01339 01340 MRB_API mrb_bool 01341 mrb_obj_respond_to(mrb_state *mrb, struct RClass* c, mrb_sym mid) 01342 { 01343 khiter_t k; 01344 01345 while (c) { 01346 khash_t(mt) *h = c->mt; 01347 01348 if (h) { 01349 k = kh_get(mt, mrb, h, mid); 01350 if (k != kh_end(h)) { 01351 if (kh_value(h, k)) { 01352 return TRUE; /* method exists */ 01353 } 01354 else { 01355 return FALSE; /* undefined method */ 01356 } 01357 } 01358 } 01359 c = c->super; 01360 } 01361 return FALSE; /* no method */ 01362 } 01363 01364 MRB_API mrb_bool 01365 mrb_respond_to(mrb_state *mrb, mrb_value obj, mrb_sym mid) 01366 { 01367 return mrb_obj_respond_to(mrb, mrb_class(mrb, obj), mid); 01368 } 01369 01370 MRB_API mrb_value 01371 mrb_class_path(mrb_state *mrb, struct RClass *c) 01372 { 01373 mrb_value path; 01374 const char *name; 01375 mrb_sym classpath = mrb_intern_lit(mrb, "__classpath__"); 01376 01377 path = mrb_obj_iv_get(mrb, (struct RObject*)c, classpath); 01378 if (mrb_nil_p(path)) { 01379 struct RClass *outer = mrb_class_outer_module(mrb, c); 01380 mrb_sym sym = mrb_class_sym(mrb, c, outer); 01381 mrb_int len; 01382 01383 if (sym == 0) { 01384 return mrb_nil_value(); 01385 } 01386 else if (outer && outer != mrb->object_class) { 01387 mrb_value base = mrb_class_path(mrb, outer); 01388 path = mrb_str_buf_new(mrb, 0); 01389 if (mrb_nil_p(base)) { 01390 mrb_str_cat_lit(mrb, path, "#<Class:"); 01391 mrb_str_concat(mrb, path, mrb_ptr_to_str(mrb, outer)); 01392 mrb_str_cat_lit(mrb, path, ">"); 01393 } 01394 else { 01395 mrb_str_concat(mrb, path, base); 01396 } 01397 mrb_str_cat_lit(mrb, path, "::"); 01398 name = mrb_sym2name_len(mrb, sym, &len); 01399 mrb_str_cat(mrb, path, name, len); 01400 } 01401 else { 01402 name = mrb_sym2name_len(mrb, sym, &len); 01403 path = mrb_str_new(mrb, name, len); 01404 } 01405 mrb_obj_iv_set(mrb, (struct RObject*)c, classpath, path); 01406 } 01407 return path; 01408 } 01409 01410 MRB_API struct RClass * 01411 mrb_class_real(struct RClass* cl) 01412 { 01413 if (cl == 0) 01414 return NULL; 01415 while ((cl->tt == MRB_TT_SCLASS) || (cl->tt == MRB_TT_ICLASS)) { 01416 cl = cl->super; 01417 } 01418 return cl; 01419 } 01420 01421 MRB_API const char* 01422 mrb_class_name(mrb_state *mrb, struct RClass* c) 01423 { 01424 mrb_value path = mrb_class_path(mrb, c); 01425 if (mrb_nil_p(path)) { 01426 path = mrb_str_new_lit(mrb, "#<Class:"); 01427 mrb_str_concat(mrb, path, mrb_ptr_to_str(mrb, c)); 01428 mrb_str_cat_lit(mrb, path, ">"); 01429 } 01430 return RSTRING_PTR(path); 01431 } 01432 01433 MRB_API const char* 01434 mrb_obj_classname(mrb_state *mrb, mrb_value obj) 01435 { 01436 return mrb_class_name(mrb, mrb_obj_class(mrb, obj)); 01437 } 01438 01439 /*! 01440 * Ensures a class can be derived from super. 01441 * 01442 * \param super a reference to an object. 01443 * \exception TypeError if \a super is not a Class or \a super is a singleton class. 01444 */ 01445 static void 01446 mrb_check_inheritable(mrb_state *mrb, struct RClass *super) 01447 { 01448 if (super->tt != MRB_TT_CLASS) { 01449 mrb_raisef(mrb, E_TYPE_ERROR, "superclass must be a Class (%S given)", mrb_obj_value(super)); 01450 } 01451 if (super->tt == MRB_TT_SCLASS) { 01452 mrb_raise(mrb, E_TYPE_ERROR, "can't make subclass of singleton class"); 01453 } 01454 if (super == mrb->class_class) { 01455 mrb_raise(mrb, E_TYPE_ERROR, "can't make subclass of Class"); 01456 } 01457 } 01458 01459 /*! 01460 * Creates a new class. 01461 * \param super a class from which the new class derives. 01462 * \exception TypeError \a super is not inheritable. 01463 * \exception TypeError \a super is the Class class. 01464 */ 01465 MRB_API struct RClass* 01466 mrb_class_new(mrb_state *mrb, struct RClass *super) 01467 { 01468 struct RClass *c; 01469 01470 if (super) { 01471 mrb_check_inheritable(mrb, super); 01472 } 01473 c = boot_defclass(mrb, super); 01474 if (super) { 01475 MRB_SET_INSTANCE_TT(c, MRB_INSTANCE_TT(super)); 01476 } 01477 make_metaclass(mrb, c); 01478 01479 return c; 01480 } 01481 01482 /*! 01483 * Creates a new module. 01484 */ 01485 MRB_API struct RClass* 01486 mrb_module_new(mrb_state *mrb) 01487 { 01488 struct RClass *m = (struct RClass*)mrb_obj_alloc(mrb, MRB_TT_MODULE, mrb->module_class); 01489 m->mt = kh_init(mt, mrb); 01490 01491 return m; 01492 } 01493 01494 /* 01495 * call-seq: 01496 * obj.class => class 01497 * 01498 * Returns the class of <i>obj</i>, now preferred over 01499 * <code>Object#type</code>, as an object's type in Ruby is only 01500 * loosely tied to that object's class. This method must always be 01501 * called with an explicit receiver, as <code>class</code> is also a 01502 * reserved word in Ruby. 01503 * 01504 * 1.class #=> Fixnum 01505 * self.class #=> Object 01506 */ 01507 01508 MRB_API struct RClass* 01509 mrb_obj_class(mrb_state *mrb, mrb_value obj) 01510 { 01511 return mrb_class_real(mrb_class(mrb, obj)); 01512 } 01513 01514 MRB_API void 01515 mrb_alias_method(mrb_state *mrb, struct RClass *c, mrb_sym a, mrb_sym b) 01516 { 01517 struct RProc *m = mrb_method_search(mrb, c, b); 01518 01519 mrb_define_method_vm(mrb, c, a, mrb_obj_value(m)); 01520 } 01521 01522 /*! 01523 * Defines an alias of a method. 01524 * \param klass the class which the original method belongs to 01525 * \param name1 a new name for the method 01526 * \param name2 the original name of the method 01527 */ 01528 MRB_API void 01529 mrb_define_alias(mrb_state *mrb, struct RClass *klass, const char *name1, const char *name2) 01530 { 01531 mrb_alias_method(mrb, klass, mrb_intern_cstr(mrb, name1), mrb_intern_cstr(mrb, name2)); 01532 } 01533 01534 /* 01535 * call-seq: 01536 * mod.to_s -> string 01537 * 01538 * Return a string representing this module or class. For basic 01539 * classes and modules, this is the name. For singletons, we 01540 * show information on the thing we're attached to as well. 01541 */ 01542 01543 static mrb_value 01544 mrb_mod_to_s(mrb_state *mrb, mrb_value klass) 01545 { 01546 mrb_value str; 01547 01548 if (mrb_type(klass) == MRB_TT_SCLASS) { 01549 mrb_value v = mrb_iv_get(mrb, klass, mrb_intern_lit(mrb, "__attached__")); 01550 01551 str = mrb_str_new_lit(mrb, "#<Class:"); 01552 01553 switch (mrb_type(v)) { 01554 case MRB_TT_CLASS: 01555 case MRB_TT_MODULE: 01556 case MRB_TT_SCLASS: 01557 mrb_str_append(mrb, str, mrb_inspect(mrb, v)); 01558 break; 01559 default: 01560 mrb_str_append(mrb, str, mrb_any_to_s(mrb, v)); 01561 break; 01562 } 01563 return mrb_str_cat_lit(mrb, str, ">"); 01564 } 01565 else { 01566 struct RClass *c; 01567 mrb_value path; 01568 01569 str = mrb_str_buf_new(mrb, 32); 01570 c = mrb_class_ptr(klass); 01571 path = mrb_class_path(mrb, c); 01572 01573 if (mrb_nil_p(path)) { 01574 switch (mrb_type(klass)) { 01575 case MRB_TT_CLASS: 01576 mrb_str_cat_lit(mrb, str, "#<Class:"); 01577 break; 01578 01579 case MRB_TT_MODULE: 01580 mrb_str_cat_lit(mrb, str, "#<Module:"); 01581 break; 01582 01583 default: 01584 /* Shouldn't be happened? */ 01585 mrb_str_cat_lit(mrb, str, "#<??????:"); 01586 break; 01587 } 01588 mrb_str_concat(mrb, str, mrb_ptr_to_str(mrb, c)); 01589 return mrb_str_cat_lit(mrb, str, ">"); 01590 } 01591 else { 01592 return path; 01593 } 01594 } 01595 } 01596 01597 static mrb_value 01598 mrb_mod_alias(mrb_state *mrb, mrb_value mod) 01599 { 01600 struct RClass *c = mrb_class_ptr(mod); 01601 mrb_sym new_name, old_name; 01602 01603 mrb_get_args(mrb, "nn", &new_name, &old_name); 01604 mrb_alias_method(mrb, c, new_name, old_name); 01605 return mrb_nil_value(); 01606 } 01607 01608 static void 01609 undef_method(mrb_state *mrb, struct RClass *c, mrb_sym a) 01610 { 01611 mrb_value m; 01612 01613 if (!mrb_obj_respond_to(mrb, c, a)) { 01614 mrb_name_error(mrb, a, "undefined method '%S' for class '%S'", mrb_sym2str(mrb, a), mrb_obj_value(c)); 01615 } 01616 else { 01617 SET_PROC_VALUE(m, 0); 01618 mrb_define_method_vm(mrb, c, a, m); 01619 } 01620 } 01621 01622 MRB_API void 01623 mrb_undef_method(mrb_state *mrb, struct RClass *c, const char *name) 01624 { 01625 undef_method(mrb, c, mrb_intern_cstr(mrb, name)); 01626 } 01627 01628 MRB_API void 01629 mrb_undef_class_method(mrb_state *mrb, struct RClass *c, const char *name) 01630 { 01631 mrb_undef_method(mrb, mrb_class_ptr(mrb_singleton_class(mrb, mrb_obj_value(c))), name); 01632 } 01633 01634 static mrb_value 01635 mrb_mod_undef(mrb_state *mrb, mrb_value mod) 01636 { 01637 struct RClass *c = mrb_class_ptr(mod); 01638 mrb_int argc; 01639 mrb_value *argv; 01640 01641 mrb_get_args(mrb, "*", &argv, &argc); 01642 while (argc--) { 01643 undef_method(mrb, c, mrb_symbol(*argv)); 01644 argv++; 01645 } 01646 return mrb_nil_value(); 01647 } 01648 01649 static mrb_value 01650 mod_define_method(mrb_state *mrb, mrb_value self) 01651 { 01652 struct RClass *c = mrb_class_ptr(self); 01653 struct RProc *p; 01654 mrb_sym mid; 01655 mrb_value blk; 01656 01657 mrb_get_args(mrb, "n&", &mid, &blk); 01658 if (mrb_nil_p(blk)) { 01659 mrb_raise(mrb, E_ARGUMENT_ERROR, "no block given"); 01660 } 01661 p = (struct RProc*)mrb_obj_alloc(mrb, MRB_TT_PROC, mrb->proc_class); 01662 mrb_proc_copy(p, mrb_proc_ptr(blk)); 01663 p->flags |= MRB_PROC_STRICT; 01664 mrb_define_method_raw(mrb, c, mid, p); 01665 return mrb_symbol_value(mid); 01666 } 01667 01668 static void 01669 check_cv_name_str(mrb_state *mrb, mrb_value str) 01670 { 01671 const char *s = RSTRING_PTR(str); 01672 mrb_int len = RSTRING_LEN(str); 01673 01674 if (len < 3 || !(s[0] == '@' && s[1] == '@')) { 01675 mrb_name_error(mrb, mrb_intern_str(mrb, str), "`%S' is not allowed as a class variable name", str); 01676 } 01677 } 01678 01679 static void 01680 check_cv_name_sym(mrb_state *mrb, mrb_sym id) 01681 { 01682 check_cv_name_str(mrb, mrb_sym2str(mrb, id)); 01683 } 01684 01685 /* 15.2.2.4.16 */ 01686 /* 01687 * call-seq: 01688 * obj.class_variable_defined?(symbol) -> true or false 01689 * 01690 * Returns <code>true</code> if the given class variable is defined 01691 * in <i>obj</i>. 01692 * 01693 * class Fred 01694 * @@foo = 99 01695 * end 01696 * Fred.class_variable_defined?(:@@foo) #=> true 01697 * Fred.class_variable_defined?(:@@bar) #=> false 01698 */ 01699 01700 static mrb_value 01701 mrb_mod_cvar_defined(mrb_state *mrb, mrb_value mod) 01702 { 01703 mrb_sym id; 01704 01705 mrb_get_args(mrb, "n", &id); 01706 check_cv_name_sym(mrb, id); 01707 return mrb_bool_value(mrb_cv_defined(mrb, mod, id)); 01708 } 01709 01710 /* 15.2.2.4.17 */ 01711 /* 01712 * call-seq: 01713 * mod.class_variable_get(symbol) -> obj 01714 * 01715 * Returns the value of the given class variable (or throws a 01716 * <code>NameError</code> exception). The <code>@@</code> part of the 01717 * variable name should be included for regular class variables 01718 * 01719 * class Fred 01720 * @@foo = 99 01721 * end 01722 * Fred.class_variable_get(:@@foo) #=> 99 01723 */ 01724 01725 static mrb_value 01726 mrb_mod_cvar_get(mrb_state *mrb, mrb_value mod) 01727 { 01728 mrb_sym id; 01729 01730 mrb_get_args(mrb, "n", &id); 01731 check_cv_name_sym(mrb, id); 01732 return mrb_cv_get(mrb, mod, id); 01733 } 01734 01735 /* 15.2.2.4.18 */ 01736 /* 01737 * call-seq: 01738 * obj.class_variable_set(symbol, obj) -> obj 01739 * 01740 * Sets the class variable names by <i>symbol</i> to 01741 * <i>object</i>. 01742 * 01743 * class Fred 01744 * @@foo = 99 01745 * def foo 01746 * @@foo 01747 * end 01748 * end 01749 * Fred.class_variable_set(:@@foo, 101) #=> 101 01750 * Fred.new.foo #=> 101 01751 */ 01752 01753 static mrb_value 01754 mrb_mod_cvar_set(mrb_state *mrb, mrb_value mod) 01755 { 01756 mrb_value value; 01757 mrb_sym id; 01758 01759 mrb_get_args(mrb, "no", &id, &value); 01760 check_cv_name_sym(mrb, id); 01761 mrb_cv_set(mrb, mod, id, value); 01762 return value; 01763 } 01764 01765 /* 15.2.2.4.39 */ 01766 /* 01767 * call-seq: 01768 * remove_class_variable(sym) -> obj 01769 * 01770 * Removes the definition of the <i>sym</i>, returning that 01771 * constant's value. 01772 * 01773 * class Dummy 01774 * @@var = 99 01775 * puts @@var 01776 * p class_variables 01777 * remove_class_variable(:@@var) 01778 * p class_variables 01779 * end 01780 * 01781 * <em>produces:</em> 01782 * 01783 * 99 01784 * [:@@var] 01785 * [] 01786 */ 01787 01788 static mrb_value 01789 mrb_mod_remove_cvar(mrb_state *mrb, mrb_value mod) 01790 { 01791 mrb_value val; 01792 mrb_sym id; 01793 01794 mrb_get_args(mrb, "n", &id); 01795 check_cv_name_sym(mrb, id); 01796 01797 val = mrb_iv_remove(mrb, mod, id); 01798 if (!mrb_undef_p(val)) return val; 01799 01800 if (mrb_cv_defined(mrb, mod, id)) { 01801 mrb_name_error(mrb, id, "cannot remove %S for %S", 01802 mrb_sym2str(mrb, id), mod); 01803 } 01804 01805 mrb_name_error(mrb, id, "class variable %S not defined for %S", 01806 mrb_sym2str(mrb, id), mod); 01807 01808 /* not reached */ 01809 return mrb_nil_value(); 01810 } 01811 01812 /* 15.2.2.4.34 */ 01813 /* 01814 * call-seq: 01815 * mod.method_defined?(symbol) -> true or false 01816 * 01817 * Returns +true+ if the named method is defined by 01818 * _mod_ (or its included modules and, if _mod_ is a class, 01819 * its ancestors). Public and protected methods are matched. 01820 * 01821 * module A 01822 * def method1() end 01823 * end 01824 * class B 01825 * def method2() end 01826 * end 01827 * class C < B 01828 * include A 01829 * def method3() end 01830 * end 01831 * 01832 * A.method_defined? :method1 #=> true 01833 * C.method_defined? "method1" #=> true 01834 * C.method_defined? "method2" #=> true 01835 * C.method_defined? "method3" #=> true 01836 * C.method_defined? "method4" #=> false 01837 */ 01838 01839 static mrb_value 01840 mrb_mod_method_defined(mrb_state *mrb, mrb_value mod) 01841 { 01842 mrb_sym id; 01843 01844 mrb_get_args(mrb, "n", &id); 01845 return mrb_bool_value(mrb_obj_respond_to(mrb, mrb_class_ptr(mod), id)); 01846 } 01847 01848 static void 01849 remove_method(mrb_state *mrb, mrb_value mod, mrb_sym mid) 01850 { 01851 struct RClass *c = mrb_class_ptr(mod); 01852 khash_t(mt) *h = c->mt; 01853 khiter_t k; 01854 01855 if (h) { 01856 k = kh_get(mt, mrb, h, mid); 01857 if (k != kh_end(h)) { 01858 kh_del(mt, mrb, h, k); 01859 return; 01860 } 01861 } 01862 01863 mrb_name_error(mrb, mid, "method `%S' not defined in %S", 01864 mrb_sym2str(mrb, mid), mod); 01865 } 01866 01867 /* 15.2.2.4.41 */ 01868 /* 01869 * call-seq: 01870 * remove_method(symbol) -> self 01871 * 01872 * Removes the method identified by _symbol_ from the current 01873 * class. For an example, see <code>Module.undef_method</code>. 01874 */ 01875 01876 static mrb_value 01877 mrb_mod_remove_method(mrb_state *mrb, mrb_value mod) 01878 { 01879 mrb_int argc; 01880 mrb_value *argv; 01881 01882 mrb_get_args(mrb, "*", &argv, &argc); 01883 while (argc--) { 01884 remove_method(mrb, mod, mrb_symbol(*argv)); 01885 argv++; 01886 } 01887 return mod; 01888 } 01889 01890 01891 01892 static void 01893 check_const_name_str(mrb_state *mrb, mrb_value str) 01894 { 01895 if (RSTRING_LEN(str) < 1 || !ISUPPER(*RSTRING_PTR(str))) { 01896 mrb_name_error(mrb, mrb_intern_str(mrb, str), "wrong constant name %S", str); 01897 } 01898 } 01899 01900 static void 01901 check_const_name_sym(mrb_state *mrb, mrb_sym id) 01902 { 01903 check_const_name_str(mrb, mrb_sym2str(mrb, id)); 01904 } 01905 01906 static mrb_value 01907 const_defined(mrb_state *mrb, mrb_value mod, mrb_sym id, mrb_bool inherit) 01908 { 01909 if (inherit) { 01910 return mrb_bool_value(mrb_const_defined(mrb, mod, id)); 01911 } 01912 return mrb_bool_value(mrb_const_defined_at(mrb, mod, id)); 01913 } 01914 01915 static mrb_value 01916 mrb_mod_const_defined(mrb_state *mrb, mrb_value mod) 01917 { 01918 mrb_sym id; 01919 mrb_bool inherit = TRUE; 01920 01921 mrb_get_args(mrb, "n|b", &id, &inherit); 01922 check_const_name_sym(mrb, id); 01923 return const_defined(mrb, mod, id, inherit); 01924 } 01925 01926 static mrb_value 01927 mrb_mod_const_get(mrb_state *mrb, mrb_value mod) 01928 { 01929 mrb_sym id; 01930 01931 mrb_get_args(mrb, "n", &id); 01932 check_const_name_sym(mrb, id); 01933 return mrb_const_get(mrb, mod, id); 01934 } 01935 01936 static mrb_value 01937 mrb_mod_const_set(mrb_state *mrb, mrb_value mod) 01938 { 01939 mrb_sym id; 01940 mrb_value value; 01941 01942 mrb_get_args(mrb, "no", &id, &value); 01943 check_const_name_sym(mrb, id); 01944 mrb_const_set(mrb, mod, id, value); 01945 return value; 01946 } 01947 01948 static mrb_value 01949 mrb_mod_remove_const(mrb_state *mrb, mrb_value mod) 01950 { 01951 mrb_sym id; 01952 mrb_value val; 01953 01954 mrb_get_args(mrb, "n", &id); 01955 check_const_name_sym(mrb, id); 01956 val = mrb_iv_remove(mrb, mod, id); 01957 if (mrb_undef_p(val)) { 01958 mrb_name_error(mrb, id, "constant %S not defined", mrb_sym2str(mrb, id)); 01959 } 01960 return val; 01961 } 01962 01963 static mrb_value 01964 mrb_mod_const_missing(mrb_state *mrb, mrb_value mod) 01965 { 01966 mrb_sym sym; 01967 01968 mrb_get_args(mrb, "n", &sym); 01969 01970 if (mrb_class_real(mrb_class_ptr(mod)) != mrb->object_class) { 01971 mrb_name_error(mrb, sym, "uninitialized constant %S::%S", 01972 mod, 01973 mrb_sym2str(mrb, sym)); 01974 } 01975 else { 01976 mrb_name_error(mrb, sym, "uninitialized constant %S", 01977 mrb_sym2str(mrb, sym)); 01978 } 01979 /* not reached */ 01980 return mrb_nil_value(); 01981 } 01982 01983 static mrb_value 01984 mrb_mod_s_constants(mrb_state *mrb, mrb_value mod) 01985 { 01986 mrb_raise(mrb, E_NOTIMP_ERROR, "Module.constants not implemented"); 01987 return mrb_nil_value(); /* not reached */ 01988 } 01989 01990 static mrb_value 01991 mrb_mod_eqq(mrb_state *mrb, mrb_value mod) 01992 { 01993 mrb_value obj; 01994 mrb_bool eqq; 01995 01996 mrb_get_args(mrb, "o", &obj); 01997 eqq = mrb_obj_is_kind_of(mrb, obj, mrb_class_ptr(mod)); 01998 01999 return mrb_bool_value(eqq); 02000 } 02001 02002 MRB_API mrb_value 02003 mrb_mod_module_function(mrb_state *mrb, mrb_value mod) 02004 { 02005 mrb_value *argv; 02006 mrb_int argc, i; 02007 mrb_sym mid; 02008 struct RProc *method_rproc; 02009 struct RClass *rclass; 02010 int ai; 02011 02012 mrb_check_type(mrb, mod, MRB_TT_MODULE); 02013 02014 mrb_get_args(mrb, "*", &argv, &argc); 02015 if(argc == 0) { 02016 /* set MODFUNC SCOPE if implemented */ 02017 return mod; 02018 } 02019 02020 /* set PRIVATE method visibility if implemented */ 02021 /* mrb_mod_dummy_visibility(mrb, mod); */ 02022 02023 for (i=0; i<argc; i++) { 02024 mrb_check_type(mrb, argv[i], MRB_TT_SYMBOL); 02025 02026 mid = mrb_symbol(argv[i]); 02027 rclass = mrb_class_ptr(mod); 02028 method_rproc = mrb_method_search(mrb, rclass, mid); 02029 02030 prepare_singleton_class(mrb, (struct RBasic*)rclass); 02031 ai = mrb_gc_arena_save(mrb); 02032 mrb_define_method_raw(mrb, rclass->c, mid, method_rproc); 02033 mrb_gc_arena_restore(mrb, ai); 02034 } 02035 02036 return mod; 02037 } 02038 02039 void 02040 mrb_init_class(mrb_state *mrb) 02041 { 02042 struct RClass *bob; /* BasicObject */ 02043 struct RClass *obj; /* Object */ 02044 struct RClass *mod; /* Module */ 02045 struct RClass *cls; /* Class */ 02046 02047 /* boot class hierarchy */ 02048 bob = boot_defclass(mrb, 0); 02049 obj = boot_defclass(mrb, bob); mrb->object_class = obj; 02050 mod = boot_defclass(mrb, obj); mrb->module_class = mod;/* obj -> mod */ 02051 cls = boot_defclass(mrb, mod); mrb->class_class = cls; /* obj -> cls */ 02052 /* fix-up loose ends */ 02053 bob->c = obj->c = mod->c = cls->c = cls; 02054 make_metaclass(mrb, bob); 02055 make_metaclass(mrb, obj); 02056 make_metaclass(mrb, mod); 02057 make_metaclass(mrb, cls); 02058 02059 /* name basic classes */ 02060 mrb_define_const(mrb, bob, "BasicObject", mrb_obj_value(bob)); 02061 mrb_define_const(mrb, obj, "BasicObject", mrb_obj_value(bob)); 02062 mrb_define_const(mrb, obj, "Object", mrb_obj_value(obj)); 02063 mrb_define_const(mrb, obj, "Module", mrb_obj_value(mod)); 02064 mrb_define_const(mrb, obj, "Class", mrb_obj_value(cls)); 02065 02066 /* name each classes */ 02067 name_class(mrb, bob, mrb_intern_lit(mrb, "BasicObject")); 02068 name_class(mrb, obj, mrb_intern_lit(mrb, "Object")); /* 15.2.1 */ 02069 name_class(mrb, mod, mrb_intern_lit(mrb, "Module")); /* 15.2.2 */ 02070 name_class(mrb, cls, mrb_intern_lit(mrb, "Class")); /* 15.2.3 */ 02071 02072 mrb->proc_class = mrb_define_class(mrb, "Proc", mrb->object_class); /* 15.2.17 */ 02073 MRB_SET_INSTANCE_TT(mrb->proc_class, MRB_TT_PROC); 02074 02075 MRB_SET_INSTANCE_TT(cls, MRB_TT_CLASS); 02076 mrb_define_method(mrb, bob, "initialize", mrb_bob_init, MRB_ARGS_NONE()); 02077 mrb_define_method(mrb, bob, "!", mrb_bob_not, MRB_ARGS_NONE()); 02078 mrb_define_method(mrb, bob, "method_missing", mrb_bob_missing, MRB_ARGS_ANY()); /* 15.3.1.3.30 */ 02079 02080 mrb_define_class_method(mrb, cls, "new", mrb_class_new_class, MRB_ARGS_OPT(1)); 02081 mrb_define_method(mrb, cls, "superclass", mrb_class_superclass, MRB_ARGS_NONE()); /* 15.2.3.3.4 */ 02082 mrb_define_method(mrb, cls, "new", mrb_instance_new, MRB_ARGS_ANY()); /* 15.2.3.3.3 */ 02083 mrb_define_method(mrb, cls, "initialize", mrb_class_initialize, MRB_ARGS_OPT(1)); /* 15.2.3.3.1 */ 02084 mrb_define_method(mrb, cls, "inherited", mrb_bob_init, MRB_ARGS_REQ(1)); 02085 02086 MRB_SET_INSTANCE_TT(mod, MRB_TT_MODULE); 02087 mrb_define_method(mrb, mod, "class_variable_defined?", mrb_mod_cvar_defined, MRB_ARGS_REQ(1)); /* 15.2.2.4.16 */ 02088 mrb_define_method(mrb, mod, "class_variable_get", mrb_mod_cvar_get, MRB_ARGS_REQ(1)); /* 15.2.2.4.17 */ 02089 mrb_define_method(mrb, mod, "class_variable_set", mrb_mod_cvar_set, MRB_ARGS_REQ(2)); /* 15.2.2.4.18 */ 02090 mrb_define_method(mrb, mod, "extend_object", mrb_mod_extend_object, MRB_ARGS_REQ(1)); /* 15.2.2.4.25 */ 02091 mrb_define_method(mrb, mod, "extended", mrb_bob_init, MRB_ARGS_REQ(1)); /* 15.2.2.4.26 */ 02092 mrb_define_method(mrb, mod, "include", mrb_mod_include, MRB_ARGS_ANY()); /* 15.2.2.4.27 */ 02093 mrb_define_method(mrb, mod, "include?", mrb_mod_include_p, MRB_ARGS_REQ(1)); /* 15.2.2.4.28 */ 02094 mrb_define_method(mrb, mod, "append_features", mrb_mod_append_features, MRB_ARGS_REQ(1)); /* 15.2.2.4.10 */ 02095 mrb_define_method(mrb, mod, "class_eval", mrb_mod_module_eval, MRB_ARGS_ANY()); /* 15.2.2.4.15 */ 02096 mrb_define_method(mrb, mod, "included", mrb_bob_init, MRB_ARGS_REQ(1)); /* 15.2.2.4.29 */ 02097 mrb_define_method(mrb, mod, "included_modules", mrb_mod_included_modules, MRB_ARGS_NONE()); /* 15.2.2.4.30 */ 02098 mrb_define_method(mrb, mod, "initialize", mrb_mod_initialize, MRB_ARGS_NONE()); /* 15.2.2.4.31 */ 02099 mrb_define_method(mrb, mod, "instance_methods", mrb_mod_instance_methods, MRB_ARGS_ANY()); /* 15.2.2.4.33 */ 02100 mrb_define_method(mrb, mod, "method_defined?", mrb_mod_method_defined, MRB_ARGS_REQ(1)); /* 15.2.2.4.34 */ 02101 mrb_define_method(mrb, mod, "module_eval", mrb_mod_module_eval, MRB_ARGS_ANY()); /* 15.2.2.4.35 */ 02102 mrb_define_method(mrb, mod, "module_function", mrb_mod_module_function, MRB_ARGS_ANY()); 02103 mrb_define_method(mrb, mod, "private", mrb_mod_dummy_visibility, MRB_ARGS_ANY()); /* 15.2.2.4.36 */ 02104 mrb_define_method(mrb, mod, "protected", mrb_mod_dummy_visibility, MRB_ARGS_ANY()); /* 15.2.2.4.37 */ 02105 mrb_define_method(mrb, mod, "public", mrb_mod_dummy_visibility, MRB_ARGS_ANY()); /* 15.2.2.4.38 */ 02106 mrb_define_method(mrb, mod, "remove_class_variable", mrb_mod_remove_cvar, MRB_ARGS_REQ(1)); /* 15.2.2.4.39 */ 02107 mrb_define_method(mrb, mod, "remove_method", mrb_mod_remove_method, MRB_ARGS_ANY()); /* 15.2.2.4.41 */ 02108 mrb_define_method(mrb, mod, "attr_reader", mrb_mod_attr_reader, MRB_ARGS_ANY()); /* 15.2.2.4.13 */ 02109 mrb_define_method(mrb, mod, "attr_writer", mrb_mod_attr_writer, MRB_ARGS_ANY()); /* 15.2.2.4.14 */ 02110 mrb_define_method(mrb, mod, "to_s", mrb_mod_to_s, MRB_ARGS_NONE()); 02111 mrb_define_method(mrb, mod, "inspect", mrb_mod_to_s, MRB_ARGS_NONE()); 02112 mrb_define_method(mrb, mod, "alias_method", mrb_mod_alias, MRB_ARGS_ANY()); /* 15.2.2.4.8 */ 02113 mrb_define_method(mrb, mod, "ancestors", mrb_mod_ancestors, MRB_ARGS_NONE()); /* 15.2.2.4.9 */ 02114 mrb_define_method(mrb, mod, "undef_method", mrb_mod_undef, MRB_ARGS_ANY()); /* 15.2.2.4.41 */ 02115 mrb_define_method(mrb, mod, "const_defined?", mrb_mod_const_defined, MRB_ARGS_ARG(1,1)); /* 15.2.2.4.20 */ 02116 mrb_define_method(mrb, mod, "const_get", mrb_mod_const_get, MRB_ARGS_REQ(1)); /* 15.2.2.4.21 */ 02117 mrb_define_method(mrb, mod, "const_set", mrb_mod_const_set, MRB_ARGS_REQ(2)); /* 15.2.2.4.23 */ 02118 mrb_define_method(mrb, mod, "constants", mrb_mod_constants, MRB_ARGS_OPT(1)); /* 15.2.2.4.24 */ 02119 mrb_define_method(mrb, mod, "remove_const", mrb_mod_remove_const, MRB_ARGS_REQ(1)); /* 15.2.2.4.40 */ 02120 mrb_define_method(mrb, mod, "const_missing", mrb_mod_const_missing, MRB_ARGS_REQ(1)); 02121 mrb_define_method(mrb, mod, "define_method", mod_define_method, MRB_ARGS_REQ(1)); 02122 mrb_define_method(mrb, mod, "class_variables", mrb_mod_class_variables, MRB_ARGS_NONE()); /* 15.2.2.4.19 */ 02123 mrb_define_method(mrb, mod, "===", mrb_mod_eqq, MRB_ARGS_REQ(1)); 02124 mrb_define_class_method(mrb, mod, "constants", mrb_mod_s_constants, MRB_ARGS_ANY()); /* 15.2.2.3.1 */ 02125 02126 mrb_undef_method(mrb, cls, "append_features"); 02127 mrb_undef_method(mrb, cls, "extend_object"); 02128 } 02129
Generated on Tue Jul 12 2022 18:00:34 by
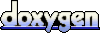