SDFileSystem, slightly modified for the ExoController
Dependents: Data-Management-Honka
Fork of SDFileSystem_HelloWorld by
SDFile.cpp
00001 #include "mbed.h" 00002 #include "SDFileSystem.h" 00003 #include "SDFile.h" 00004 #include "errno.h" 00005 #include "initDatabed.h" 00006 00007 00008 SDFile::SDFile(string path, string filename, bool readOnly) 00009 { 00010 //Creates the necessary directory 00011 printf("Creating SDFile object\r\n"); 00012 char *a = new char[path.size()+1]; 00013 a[path.size()] = 0; 00014 memcpy(a,path.c_str(),path.size()); 00015 //Calculates the full filename, then creates the file 00016 std::string fullname = path + filename; 00017 00018 char *b = new char[fullname.size()+1]; 00019 b[fullname.size()] = 0; 00020 memcpy(b,fullname.c_str(),fullname.size()); 00021 if (readOnly) { 00022 //printf("Opening readonly file\r\n"); 00023 //_fp = fopen((const char*)b, "r"); 00024 } else { 00025 printf("Opening writable file %s\r\n", b); 00026 _fp = fopen((const char*)b, "w+"); 00027 if (_fp == NULL) { 00028 //boardLed3 = 1; 00029 newFile = true; 00030 printf("file %s not found\r\n", b); 00031 _fp = fopen((const char*)b, "w+"); 00032 } else { 00033 newFile = false; 00034 printf("file %s found\r\n", b); 00035 } 00036 } 00037 //printf("fp is %d\r\n", _fp); 00038 } 00039 00040 /** 00041 * This function reads bytes from the SDFile object. 00042 * @author Michael Ling 00043 * @param length The number of bytes to read 00044 * @param array The int array the bytes are copied to 00045 * @date 2/2/2015 00046 */ 00047 int* SDFile::read(int length, int *array) 00048 { 00049 00050 //shift to the end of the file and go back accounting for the commas, spaces, \n, and \r (6 places per data) 00051 fseek(_fp, -6*length, SEEK_END); 00052 00053 //pc.printf("reading to array at %d\r\n", &array[0]); 00054 //cycle through the length of the vector and read the values. 00055 for(int i = 0; i < length; i++) { 00056 //pc.printf("File %d, array %d\r\n", _fp, &array[i]); 00057 fscanf(_fp, "%x, ", &array[i]); 00058 // pc.printf("HHHHH\r\n", s); 00059 //pc.printf("read %x now at %ld\r\n", array[i], ftell(_fp)); 00060 } 00061 00062 return array; 00063 } 00064 00065 int* SDFile::read_from_start(int length, int *array) { 00066 fseek(_fp, 0, SEEK_SET); 00067 for(int i = 0; i < length; i++) { 00068 fscanf(_fp, "%x, ", &array[i]); 00069 } 00070 00071 return array; 00072 } 00073 00074 //Debug only--prints file contents, 6 chars to a line for up to 23 lines 00075 void SDFile::debug_print() { 00076 fseek(_fp, 0, SEEK_SET); 00077 char c; 00078 for (int i = 0; i < 23; i++) { 00079 for (int j = 0; j < 6; j++) { 00080 fscanf(_fp, "%c", &c); 00081 pc.printf("%c", c); 00082 } 00083 pc.printf("\r\n"); 00084 } 00085 } 00086 00087 /** 00088 * This function writes from an array to the file pointed to by fp 00089 * @param length length of data to write 00090 * @param array array to draw written data from 00091 * @author Michael Ling 00092 * @date 2/2/2015 00093 */ 00094 void SDFile::write(int length, int *array) 00095 { 00096 //printf("Seeking START...\r\n"); 00097 fseek(_fp, 0, SEEK_SET); 00098 // printf("Writing %d\r\n", array[0]); 00099 //printf("Starting to write\r\n"); 00100 // int w; 00101 for(int i = 0; i < length-1; i++) { 00102 /* This line fails when run on testFile, reason unknown...*/ 00103 //printf("%d\r\n", fprintf(_fp, "%04x, ", array[i])); 00104 //pc.printf("Writing at %ld\r\n", ftell(_fp)); 00105 fprintf(_fp, "%04x, ", array[i]); 00106 //pc.printf("Wrote %x, now at %ld\r\n", array[i], ftell(_fp)); 00107 } 00108 //printf("%d\r\n", fprintf(_fp, "%04x\r\n", array[length-1])); 00109 fprintf(_fp, "%04x\r\n", array[length-1]); 00110 //fflush(_fp); 00111 } 00112 00113 /** This function writes to a single position in the file 00114 * @param index write to this index of the file, or at offset index*6 from the start 00115 * @param value the new value to be written to that position 00116 * @author Michael Ling 00117 * @date 4/24/2015 00118 */ 00119 void SDFile::write_to_index(int index, int value) 00120 { 00121 fseek(_fp, 6*index, SEEK_SET); 00122 //pc.printf("Index %d, position %ld\r\n", 6*index, ftell(_fp)); 00123 //fscanf(_fp, "%x, ", &val); 00124 //pc.printf("Val is %x\r\n", val); 00125 //pc.printf("%d, %d\r\n", sizeof value, sizeof 0xdead); 00126 //pc.printf("%04x, ", value); 00127 //The &0xffff forces the float to write only 4 characters--without it floats become 8 chars long, for some reason 00128 int w = fprintf(_fp, "%04x, ", value & 0xffff); 00129 //pc.printf("Wrote %d, now at %ld\r\n", w, ftell(_fp)); 00130 fflush(_fp); 00131 } 00132 00133 void SDFile::append(int length, int *array) { 00134 fseek(_fp, 0, SEEK_END); 00135 for(int i = 0; i < length-1; i++) { 00136 00137 fprintf(_fp, "%04x, ", array[i]); 00138 } 00139 fprintf(_fp, "%04x\r\n", array[length-1]); 00140 } 00141 /* 00142 void SDFile::changeAccess(string path, string filename) { 00143 fclose(_fp); 00144 char *a = new char[path.size()+1]; 00145 a[path.size()] = 0; 00146 memcpy(a,path.c_str(),path.size()); 00147 //Calculates the full filename, then creates the file 00148 std::string fullname = path + filename; 00149 00150 char *b = new char[fullname.size()+1]; 00151 00152 b[fullname.size()] = 0; 00153 memcpy(b,fullname.c_str(),fullname.size()); 00154 printf("Using name %s\r\n", b); 00155 _fp = fopen((const char *)b, "r+"); 00156 printf("new FP is %d\r\n", _fp); 00157 }*/ 00158 00159 void SDFile::open_for_read(string path, string filename) { 00160 //Creates the necessary directory 00161 //printf("Trying to open for read\r\n"); 00162 char *a = new char[path.size()+1]; 00163 a[path.size()] = 0; 00164 memcpy(a,path.c_str(),path.size()); 00165 //Calculates the full filename, then creates the file 00166 std::string fullname = path + filename; 00167 00168 char *b = new char[fullname.size()+1]; 00169 b[fullname.size()] = 0; 00170 memcpy(b,fullname.c_str(),fullname.size()); 00171 _fp = fopen((const char*)b, "r"); 00172 00173 //printf("fp is %d\r\n", _fp); 00174 } 00175 00176 void SDFile::open_for_write(string path, string filename) { 00177 00178 //Creates the necessary directory 00179 00180 char *a = new char[path.size()+1]; 00181 a[path.size()] = 0; 00182 memcpy(a,path.c_str(),path.size()); 00183 //Calculates the full filename, then creates the file 00184 std::string fullname = path + filename; 00185 00186 char *b = new char[fullname.size()+1]; 00187 b[fullname.size()] = 0; 00188 memcpy(b,fullname.c_str(),fullname.size()); 00189 _fp = fopen((const char*)b, "w+"); 00190 00191 //printf("fp is %d\r\n", _fp); 00192 } 00193 00194 void SDFile::close() { 00195 fclose(_fp); 00196 }
Generated on Tue Jul 19 2022 21:06:39 by
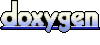