
Embed:
(wiki syntax)
Show/hide line numbers
ucam.h
00001 #ifndef __UCAM__H__ 00002 #define __UCAM__H__ 00003 #include <mbed.h> 00004 /*color space*/ 00005 #define COLOR_YUV_2 0x01 00006 #define COLOR_YUV_4 0x02 00007 #define COLOR_YUV_8 0x03 00008 #define COLOR_RGB_332 0x04 00009 #define COLOR_RGB_444 0x05 00010 #define COLOR_RGB_565 0x06 00011 #define COLOR_JPEG 0x07 00012 /*raw resolution*/ 00013 #define RAW_80x60 0x01 00014 #define RAW_160x120 0x03 00015 #define RAW_320x240 0x05 00016 #define RAW_640x480 0x07 00017 #define RAW_128x128 0x09 00018 #define RAW_128x96 0x0B 00019 /*jpeg resolution*/ 00020 #define JPEG_80x64 0x01 00021 #define JPEG_160x128 0x03 00022 #define JPEG_320x240 0x05 00023 #define JPEG_640x480 0x07 00024 struct ucam_command; 00025 00026 class UCam{ 00027 public: 00028 Serial *ucam; 00029 DigitalOut led; 00030 00031 public: 00032 UCam(PinName tx_pin, PinName rx_pin, PinName led_pin, uint32_t baudrate); 00033 ~UCam(); 00034 int8_t connect(); 00035 int8_t snapshot(uint8_t *buf, uint16_t *len, uint8_t colorspace, uint8_t raw_size, uint8_t jpeg_size); 00036 int8_t start_video(uint8_t colorspace, uint8_t raw_size, uint8_t jpeg_size); 00037 int8_t next_frame(uint8_t *buf, uint16_t *len); 00038 int8_t end_video(); 00039 00040 private: 00041 void uart_isr(); 00042 int8_t send_cmd(uint8_t cmd, uint8_t p1=0, uint8_t p2=0, uint8_t p3=0, uint8_t p4=0, uint8_t noack=0); 00043 int8_t recv_cmd(struct ucam_command *cmd); 00044 }; 00045 #endif/*#define __UCAM_H__*/
Generated on Fri Jul 22 2022 08:07:26 by
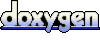