
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "ucam.h" 00003 #define BUFFER_LENGTH 4096 00004 00005 Serial firefly(p28, p27); 00006 Serial debug(USBTX, USBRX); 00007 00008 DigitalOut firefly_led(LED1); 00009 UCam ucam(p13, p14, LED2, 1228800); // tx, rx, led, baud 00010 00011 uint16_t len; 00012 uint8_t ubuf[BUFFER_LENGTH]; 00013 uint8_t take_snapshot =0; 00014 00015 void firefly_isr() 00016 { 00017 while(firefly.readable()) 00018 firefly.getc(); 00019 take_snapshot = 1; 00020 } 00021 00022 int main() 00023 { 00024 debug.puts("initiating firefly...\r\n"); 00025 firefly.baud(921600); 00026 firefly.attach(firefly_isr); 00027 firefly_led = 1; 00028 00029 debug.puts("initiating ucam...\r\n"); 00030 00031 if (ucam.connect() == 0){ 00032 debug.puts("connection to ucam established\r\n"); 00033 } else { 00034 debug.puts("connection to ucam failed\r\n"); 00035 return -1; 00036 } 00037 00038 00039 if (ucam.start_video(COLOR_JPEG, JPEG_160x128, JPEG_160x128) != 0) { 00040 debug.printf("failed to start video\r\n"); 00041 return -1; 00042 } 00043 00044 Timer t; 00045 uint16_t i; 00046 00047 while (1) { 00048 00049 t.reset(); 00050 00051 if (take_snapshot 00052 && ucam.next_frame(ubuf, &len) == 0) { 00053 00054 firefly.putc(((char*)&len)[0]); //size lsb 00055 firefly.putc(((char*)&len)[1]); //size msb 00056 00057 for(i=0; i < len; i++){ 00058 firefly.putc(ubuf[i]); 00059 } 00060 00061 take_snapshot = 0; 00062 debug.printf("frame %f \r\n", t.read()); 00063 } 00064 wait_ms(10); 00065 } 00066 }
Generated on Fri Jul 22 2022 08:07:26 by
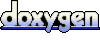