
example-reading-imei-imsi
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 u-blox 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "ATCmdParser.h" 00019 #include "FileHandle.h" 00020 #include "onboard_modem_api.h" 00021 00022 /* Definitions */ 00023 #define OUTPUT_ENTER_KEY "\r" 00024 #define AT_PARSER_BUFFER_SIZE 256 00025 #define AT_PARSER_TIMEOUT 8*1000 // Milliseconds 00026 00027 /* The example program for the u-blox C030 boards. It sets up the Cellular Module then read out the IMSI of the eUICC/SIM. 00028 * The Uer LEDs (RGB) and User Button are utilised to show the activity on the board. 00029 * When GNSS has a location fix, the Red Timepulse LED, next to the User LEDs, blinks every 1 second. 00030 */ 00031 00032 /* File handler */ 00033 FileHandle *fh; 00034 00035 /* AT Command Parser handle */ 00036 ATCmdParser *at; 00037 00038 // User LEDs 00039 DigitalOut ledRed(LED1, 1); 00040 DigitalOut ledGreen(LED2, 1); 00041 DigitalOut ledBlue(LED3, 1); 00042 00043 // Ethernet socket LED 00044 DigitalOut ledYellow(LED4,1); 00045 00046 // User Button 00047 #ifdef TARGET_UBLOX_C027 00048 // No user button on C027 00049 InterruptIn userButton(NC); 00050 #else 00051 InterruptIn userButton(SW0); 00052 #endif 00053 00054 // Delay between LED changes in second 00055 volatile float delay = 0.5; 00056 00057 // To check if the user pressed the User Button or not 00058 void threadBodyUserButtonCheck(void const *args){ 00059 float delayToggle = delay; 00060 while (1){ 00061 if (userButton.read() == 1 ) { 00062 // User Button is pressed 00063 delay = 0.1; 00064 // Indicate with the Yellow LED on Ethernet socket 00065 ledYellow = 0; 00066 } 00067 else { 00068 // User button is released 00069 delay = 0.5; 00070 // Turn off the Yellow LED on Ethernet socket 00071 ledYellow = 1; 00072 } 00073 } 00074 } 00075 00076 // Setup the modem 00077 bool setup_modem(){ 00078 00079 bool success = false; 00080 00081 /* Initialize GPIO lines */ 00082 onboard_modem_init(); 00083 00084 /* Give modem a little time to settle down */ 00085 wait_ms(250); 00086 00087 printf("Powering up the modem\r\n"); 00088 onboard_modem_power_up(); 00089 #ifdef TARGET_UBLOX_C030_N211 00090 wait_ms(5000); 00091 #else 00092 wait_ms(500); 00093 #endif 00094 00095 // Set AT parser timeout to 1sec for AT OK check 00096 at->set_timeout(1000); 00097 00098 printf("Checking for AT response from the modem\r\n"); 00099 for (int retry_count = 0; !success && (retry_count < 20); retry_count++) { 00100 printf("...wait\r\n"); 00101 // The modem tends to sends out some garbage during power up. 00102 at->flush(); 00103 00104 // AT OK talk to the modem 00105 if (at->send("AT")) { 00106 wait_ms(100); 00107 if (at->recv("OK")) { 00108 success = true; 00109 } 00110 } 00111 } 00112 00113 // Increase the parser time to 8 sec 00114 at->set_timeout(8000); 00115 00116 if (success) { 00117 printf("Configuring the modem\r\n"); 00118 00119 #ifdef TARGET_UBLOX_C030_N211 00120 // Turn off modem echoing and turn on verbose responses 00121 success = at->send("AT+CMEE=1"); 00122 #else 00123 // Set the final baud rate 00124 if (at->send("AT+IPR=%d", 115200) && at->recv("OK")) { 00125 // Need to wait for things to be sorted out on the modem side 00126 wait_ms(100); 00127 ((UARTSerial *)fh)->set_baud(115200); 00128 } 00129 00130 // Turn off modem echoing and turn on verbose responses 00131 success = at->send("ATE0;+CMEE=2") && at->recv("OK") && 00132 // The following commands are best sent separately 00133 // Turn off RTC/CTS handshaking 00134 at->send("AT&K0") && at->recv("OK") && 00135 // Set DCD circuit(109), changes in accordance with 00136 // the carrier detect status 00137 at->send("AT&C1") && at->recv("OK") && 00138 // Set DTR circuit, we ignore the state change of DTR 00139 at->send("AT&D0") && at->recv("OK"); 00140 #endif 00141 } 00142 00143 return success; 00144 } 00145 /* 00146 ** Reading the modem IMEI and eUICC/SIM IMSI 00147 ** 00148 */ 00149 00150 int main() 00151 { 00152 bool success = false; 00153 00154 printf("\n\r\n\ru-blox C030 reading the modem IMEI and eUICC/SIM IMSI\n\r"); 00155 printf("Initialising UART for modem communication"); 00156 fh = new UARTSerial(MDMTXD, MDMRXD, 9600); 00157 printf("...DONE\r\n"); 00158 00159 // NOTE: if you are experiencing problems with the AT command 00160 // exchange, change the "false" below to "true" to get debug output 00161 // from the AT command parser 00162 printf("Initialising the modem AT command parser"); 00163 at = new ATCmdParser(fh, OUTPUT_ENTER_KEY, AT_PARSER_BUFFER_SIZE, 00164 AT_PARSER_TIMEOUT, false); 00165 printf("...DONE\r\n"); 00166 00167 printf("Initializing the modem\r\n"); 00168 if (setup_modem()) { 00169 printf("...DONE\r\nThe modem powered up\r\n"); 00170 char imei[25+1]; 00171 char imsi[15+1]; 00172 printf("Reading IMEI and IMSI\r\n"); 00173 00174 #ifdef TARGET_UBLOX_C030_N211 00175 if (at->send("AT+CGSN=1") && at->recv("\nOK\n%25[^\n]\nOK\n", imei)){ 00176 printf("IMEI: %s\r\n",imei + 6); // Avoid the "+CGSN:" prefix 00177 } 00178 #else 00179 if (at->send("AT+CGSN") && at->recv("%15[^\n]\nOK\n", imei)){ 00180 printf("IMEI: %s\r\n",imei); 00181 } 00182 #endif 00183 // Sometimes it takes a little while for the SIM to be initialised, 00184 // so retry this until done 00185 at->set_timeout(1000); 00186 for (int retry_count = 0; !success && (retry_count < 10); retry_count++) { 00187 if (at->send("AT+CIMI") && at->recv("%15[^\n]\nOK\n", imsi)){ 00188 printf("IMSI: %s\r\n",imsi); 00189 success = true; 00190 } 00191 } 00192 if (!success) { 00193 printf("Unable to read IMSI: has a SIM been inserted?\r\n"); 00194 } 00195 } else { 00196 printf("Unable to intialize modem\r\n"); 00197 } 00198 00199 printf("FINISHED...\r\n"); 00200 00201 // Create threadUserButtonCheck thread 00202 Thread threadUserButtonCheck(threadBodyUserButtonCheck); 00203 00204 // Set the LED states 00205 ledRed = 0; 00206 ledGreen = 1; 00207 ledBlue = 1; 00208 00209 // Main loop 00210 while(1) { 00211 wait(delay); 00212 // Shift the LED states 00213 int carry = ledBlue; 00214 ledBlue = ledRed; 00215 ledRed = ledGreen; 00216 ledGreen = carry; 00217 } 00218 } 00219 00220 // End Of File
Generated on Sat Jul 16 2022 21:11:32 by
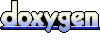