
This program plays QuickTime movies on GR-Peach
Dependencies: AsciiFont GR-PEACH_video GraphicsFramework LCD_shield_config R_BSP TLV320_RBSP mbed-rtos mbed
MovPlayer.hpp
00001 #ifndef __MovPlayer__ 00002 #define __MovPlayer__ 00003 00004 #include "rtos.h" 00005 #include "LCD.hpp" 00006 #include "R_BSP_Ssif.h" 00007 #include "MovFile.hpp" 00008 #include "CppStandardHelper.hpp" 00009 00010 class MovPlayer { 00011 public: 00012 static constexpr int BufferLength = 20; 00013 typedef mbed::Callback<int32_t (void * const, uint32_t, const rbsp_data_conf_t *)> AudioCallback; 00014 00015 private: 00016 struct VideoBuffer { 00017 char MBED_ALIGN(0x20) buf[65535]; 00018 }; 00019 struct AudioBuffer { 00020 char MBED_ALIGN(4) buf[0x1B90]; 00021 uint32_t size; 00022 }; 00023 static VideoBuffer videoBuf[BufferLength]; 00024 static AudioBuffer __attribute__((section("NC_BSS"))) audioBuf[BufferLength]; 00025 static MovPlayer singleton; 00026 static unsigned char audioThreadStack[DEFAULT_STACK_SIZE]; 00027 rtos::Thread audioThread; 00028 rtos::RtosTimer frameTimer; 00029 rtos::Semaphore frameSemaphore; 00030 rtos::Queue<VideoBuffer, BufferLength - 2> videoQueue; 00031 MovFile *mov; 00032 AudioCallback output; 00033 volatile uint32_t queueTimeout; 00034 MovPlayer(); 00035 void frameTimerCallback(); 00036 void audioProcessor(); 00037 00038 public: 00039 static MovPlayer *defaultPlayer() { 00040 return &singleton; 00041 } 00042 void play(MovFile *mov, LCD *lcd, AudioCallback callback); 00043 }; 00044 00045 #endif 00046
Generated on Fri Jul 15 2022 12:17:04 by
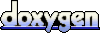