A library for the AX-12+ servo using external ICs instead of the SerialHalf-Duplex class
Embed:
(wiki syntax)
Show/hide line numbers
AX12.h
00001 /* Copyright (c) 2012 Martin Smith, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef MBED_AX12_H 00020 #define MBED_AX12_H 00021 00022 #include "mbed.h" 00023 00024 #define AX12_WRITE_DEBUG 0 00025 #define AX12_READ_DEBUG 0 00026 #define AX12_TRIGGER_DEBUG 0 00027 #define AX12_DEBUG 0 00028 00029 #define AX12_REG_GOAL_POSITION 0x1E 00030 #define AX12_REG_MOVING_SPEED 0x20 00031 #define AX12_REG_POSITION 0x24 00032 00033 #define TRANSMIT 1 00034 #define RECEIVE 0 00035 00036 /** mbed AX-12+ Servo Library - External hardware version 00037 * 00038 * Example: 00039 * @code 00040 * // Move a servo back and forth... 00041 * #include "mbed.h" 00042 * #include "Servo.h" 00043 * 00044 * Serial ax12_bus(p28,p27); 00045 * AX12 my_ax12(ax12_bus, p29, 1); 00046 * 00047 * int main() { 00048 * while(1) { 00049 * my_ax12.SetGoal(0); // move to 0 00050 * wait(2); 00051 * my_ax12.SetGoal(300); // move to 300 00052 * wait(2); 00053 * } 00054 * } 00055 * @endcode 00056 */ 00057 00058 class AX12 00059 { 00060 00061 public: 00062 /** define which pins are used, and the ID of this instance 00063 */ 00064 AX12(Serial& bus, PinName dir, int ID); 00065 00066 /** Read info from the specified AX-12 register 00067 * 00068 * @param ID the ID of the AX-12 servo 00069 * @param start the address of the 1st register to be read 00070 * @param length the number of bytes to read 00071 * @param data where the read data is stored 00072 * @returns servo status 00073 */ 00074 int read(int ID, int start, int length, char* data); 00075 00076 /** Write info to the specified AX-12 register 00077 * 00078 * @param ID the ID of the AX-12 servo 00079 * @param start the address of the 1st register to be written to 00080 * @param length the number of bytes to write 00081 * @param data where the data to be written is stored 00082 * @returns servo status 00083 */ 00084 int write(int ID, int start, int length, char* data); 00085 00086 /** Sets the goal position for the servo 00087 * 00088 * @param degrees the new target position 00089 * @returns error code 00090 */ 00091 int SetGoal(int degrees); 00092 00093 /** Getss the current position for the servo 00094 * 00095 * @returns current position 00096 */ 00097 float GetPosition(); 00098 00099 private : 00100 Serial& _bus; 00101 DigitalOut _dir; 00102 int _ID; 00103 }; 00104 #endif
Generated on Fri Jul 15 2022 12:14:15 by
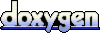