it seems that this is working stepper library
Fork of Stepper by
Embed:
(wiki syntax)
Show/hide line numbers
Stepper.h
00001 #ifndef STEPPER_H 00002 #define STEPPER_H 00003 00004 #include "mbed.h" 00005 /** Stepper class. 00006 * Used for drive Stepper motor 00007 * 00008 * Example: 00009 * @code 00010 * #include "mbed.h" 00011 * #include "Stepper.h" 00012 * 00013 * DigitalIn home(p20); 00014 * Stepper mot(p21,p22); 00015 * 00016 * int main() 00017 * { 00018 * mot.setSpeed(1200); 00019 * mot.setAcceleration(4000); 00020 * mot.setDeceleration(4000); 00021 * 00022 * mot.rotate(CW); 00023 * while(!home); 00024 * mot.stop(); 00025 * mot.setPositionZero(); 00026 * 00027 * mot.goesTo(1600); 00028 * while(!mot.stopped()); 00029 * 00030 * while(1) 00031 * { 00032 * 00033 * } 00034 * } 00035 * @endcode 00036 */ 00037 class Stepper 00038 { 00039 public: 00040 /** Create Stepper instance connected to pin clk & dir 00041 * @param clk pin to connect at clk/step output 00042 * @param dir pin to connect at dir output 00043 */ 00044 Stepper(PinName clk, PinName dir); 00045 00046 /** Moving the motor relative to a reference position (set by setPositionZero()) 00047 * @param position steps from position zero 00048 */ 00049 void goesTo(int position); 00050 00051 /** Moving the motor for given steps 00052 * @param steps number of steps to go(>0 CW ; <0 CCW) 00053 */ 00054 void move(int steps); 00055 00056 /** Moving the motor until user stop 00057 * @param direction rotation Stepper::CC or Stepper::CCW 00058 */ 00059 void rotate(bool direction); 00060 00061 /** Stop the motor as fast as possible 00062 */ 00063 void stop(void); 00064 00065 /**Set Rotation Speed 00066 * @param speed [steps/sec] 00067 */ 00068 void setSpeed(float speed); 00069 00070 /**Set Acceleration ramp 00071 * @param acc acceleration [steps/sec²] (0 = infinite acceleration 1st step @ max speed) 00072 */ 00073 void setAcceleration(float acc); 00074 00075 /**Set Deceleration ramp 00076 * @param dec deceleration [steps/sec²] (0 = infinite deceleration last step @ max speed) 00077 */ 00078 void setDeceleration(float dec); 00079 00080 /**Give Origin for motor absolute movement 00081 */ 00082 void setPositionZero(void); 00083 00084 /**Check if motor is stopped 00085 * @returns true if motor stopped 00086 */ 00087 bool stopped(void); 00088 00089 /**Get absolute position from origin 00090 * @returns position [steps] from origin set by setPositionZero() 00091 */ 00092 int getPosition(void); 00093 00094 /**Get Acceleration 00095 * @returns acceleration [steps/sec²] 00096 */ 00097 float getAcceleration(void); 00098 00099 /**Get Deceleration 00100 * @returns deceleration [steps/sec²] 00101 */ 00102 float getDeceleration(void); 00103 00104 /**Get Speed 00105 * @returns speed [steps/sec] 00106 */ 00107 float getSpeed(void); 00108 00109 /**Enum for direction 00110 * CW=true or 1; CCW=false or 0 00111 */ 00112 typedef enum {CW=1,CCW=0} direction; 00113 00114 protected: 00115 void run(void); //step generator thread 00116 unsigned int nTo(float speed,float acc); 00117 00118 private: 00119 float _acc; //Acceleration [step/s²] 00120 float _dec; //Decceleration [step/s²] 00121 float _spd; //Speed [step/s] 00122 unsigned int _steps; //nbr total of steps per mov 00123 DigitalOut _clk; //output clock pin 00124 DigitalOut _dir; //output dir pin 00125 Timeout _timer; //step timer 00126 enum {STOP,ACCEL,CRUISE,DECEL}_state; //Motor state 00127 unsigned int _dt0; //initial delay [µs] 00128 unsigned int _dtmin; //delay minimum [µs] 00129 int _pos; //motor position 00130 }; 00131 00132 #endif
Generated on Fri Jul 29 2022 01:30:15 by
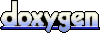