This Library allows you to use any pin on the mbed micro as PWM out. It also allows the user to update the Period and Pulse width in realtime.
main.cpp
00001 #include "mbed.h" 00002 #include "PwmOutAny.h" 00003 00004 Timer t; 00005 00006 InterruptIn SquareIn (p22); 00007 DigitalOut status (LED1); 00008 00009 00010 00011 PwmOutAny newPwm (p25,.0001,.50); 00012 00013 void measure_Period() // this is a test function it looks at a square wave on pin p22 and uses its freq. as the base freq. for the PWM. 00014 { 00015 static bool first = true; 00016 static float InputPeriod = .0001; 00017 static unsigned int count = 0; 00018 if(first) 00019 { 00020 t.reset(); 00021 t.start(); 00022 first = false; 00023 } 00024 else 00025 { 00026 00027 InputPeriod = (InputPeriod + t.read())/2; 00028 newPwm.Set_Period(InputPeriod); 00029 status = ! status; 00030 t.reset(); 00031 t.start(); 00032 count++; 00033 } 00034 00035 } 00036 00037 00038 int main() 00039 { 00040 00041 SquareIn.rise(&measure_Period); 00042 00043 while(1) 00044 { 00045 for(float t=0;t<=1;t+=.01) 00046 { 00047 newPwm.Set_PulseWidth(t); 00048 wait(.1); 00049 } 00050 for(float t=1;t>=0;t-=.01) 00051 { 00052 newPwm.Set_PulseWidth(t); 00053 wait(.1); 00054 } 00055 00056 00057 } 00058 00059 } 00060
Generated on Sat Jul 16 2022 03:08:58 by
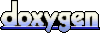