This Library allows you to use any pin on the mbed micro as PWM out. It also allows the user to update the Period and Pulse width in realtime.
PwmOutAny.cpp
00001 #include "PwmOutAny.h" 00002 00003 float _period; 00004 float _PercentHigh; 00005 00006 PwmOutAny::PwmOutAny(PinName pin1, float Period, float PercentHigh):_PwmOut(pin1) 00007 { 00008 _period=Period; 00009 _PercentHigh=PercentHigh ; 00010 _PwmTicker.attach(this,&PwmOutAny::TickerFlipper,_period/2); 00011 } 00012 00013 void PwmOutAny::TickerFlipper() 00014 { 00015 static float CurrentPeriod; 00016 if(!_PwmOut) 00017 { 00018 _PwmTicker.detach(); 00019 _PwmTicker.attach(this,&PwmOutAny::TickerFlipper,CurrentPeriod*(_PercentHigh)); 00020 _PwmOut = true; 00021 } 00022 else 00023 { 00024 CurrentPeriod = _period; 00025 _PwmTicker.detach(); 00026 _PwmTicker.attach(this,&PwmOutAny::TickerFlipper,CurrentPeriod*(1.0-_PercentHigh)); 00027 _PwmOut = false; 00028 } 00029 } 00030 00031 00032 float PwmOutAny::Get_PulseWidth() 00033 { 00034 return (_PercentHigh); 00035 00036 } 00037 void PwmOutAny::Set_PulseWidth(float PW) 00038 { 00039 _PercentHigh = PW; 00040 } 00041 float PwmOutAny::Get_Period() 00042 { 00043 return _period; 00044 } 00045 void PwmOutAny::Set_Period(float ChangePeriod) 00046 { 00047 _period = ChangePeriod; 00048 } 00049
Generated on Sat Jul 16 2022 03:08:58 by
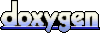