
Clock Timer
Dependencies: LCD_DISCO_F429ZI mbed BSP_DISCO_F429ZI
main.cpp
00001 #include "mbed.h" 00002 #include "LCD_DISCO_F429ZI.h" 00003 #include <math.h> 00004 00005 LCD_DISCO_F429ZI lcd; 00006 00007 DigitalOut green(LED1, 0); 00008 DigitalOut red(LED2, 1); 00009 00010 InterruptIn button(USER_BUTTON); 00011 00012 uint8_t str1[30], str2[30]; 00013 00014 int qsecs = 0; 00015 int seconds = 0; 00016 int minutes = 0; 00017 int hours = 0; 00018 00019 float multiplier = 9.5; 00020 00021 int radius = 70; 00022 int centerx = 120; 00023 int centery = 240; 00024 00025 float delaytime = 0.25; 00026 00027 struct save 00028 { 00029 bool exists; 00030 int h; 00031 int m; 00032 int s; 00033 }one, two; 00034 00035 void button_pressed() 00036 { 00037 00038 one.exists = 1; 00039 one.h = hours; 00040 one.m = minutes; 00041 one.s = seconds; 00042 00043 red = 0; 00044 green = 1; 00045 hours = 0; 00046 minutes = 0; 00047 seconds = 0; 00048 qsecs = 0; 00049 00050 lcd.Clear(LCD_COLOR_WHITE); 00051 } 00052 00053 void button_notpressed() 00054 { 00055 green = 0; 00056 red = 0; 00057 } 00058 00059 void DrawClock(int second) 00060 { 00061 00062 if (second == 0) 00063 lcd.Clear(LCD_COLOR_WHITE); 00064 00065 double angle = second/multiplier; 00066 double endx = centerx+radius*sin(angle); 00067 double endy = centery-radius*cos(angle); 00068 lcd.DrawCircle(centerx, centery, radius); 00069 lcd.DrawLine(centerx, centery, endx, endy); 00070 } 00071 00072 int main() 00073 { 00074 BSP_LCD_SetFont(&Font20); 00075 lcd.DisplayStringAt(0, LINE(5), (uint8_t *)"Timer Program", CENTER_MODE); 00076 lcd.DisplayStringAt(0, LINE(6), (uint8_t *)"Mohammad Mustafa", CENTER_MODE); 00077 wait(2); 00078 lcd.Clear(LCD_COLOR_WHITE); 00079 00080 one.exists = 0; 00081 00082 while(1) 00083 { 00084 button.rise(&button_pressed); 00085 button.fall(&button_notpressed); 00086 00087 if(button.read()==0) 00088 { 00089 red = !red; 00090 qsecs++; 00091 } 00092 if(qsecs%4 == 0 && qsecs != 0) 00093 { 00094 qsecs = 0; 00095 seconds++; 00096 } 00097 00098 if(seconds%60 == 0 && seconds != 0) 00099 { 00100 seconds = 0; 00101 minutes++; 00102 } 00103 if(minutes%12 == 0 && minutes != 0) 00104 { 00105 minutes = 0; 00106 hours++; 00107 } 00108 00109 if(one.exists) 00110 { 00111 sprintf((char*)str1, "Last Time: %d:%d:%d", one.h, one.m, one.s); 00112 lcd.DisplayStringAt(0, LINE(0), (uint8_t *)&str1, LEFT_MODE); 00113 } 00114 00115 sprintf((char*)str1, "HR:MIN:SEC:qSEC"); 00116 sprintf((char*)str2, "%d:%d:%d:%d", hours, minutes, seconds, qsecs); 00117 lcd.DisplayStringAt(0, LINE(6), (uint8_t *)&str1, CENTER_MODE); 00118 lcd.DisplayStringAt(0, LINE(7), (uint8_t *)&str2, CENTER_MODE); 00119 00120 DrawClock(seconds%60); 00121 wait(delaytime); 00122 } 00123 } 00124 00125 00126
Generated on Fri Aug 19 2022 21:37:43 by
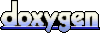