
This is my first project using the mBed to an extent. The software is a basic traffic light with a blinking LED to show it is running and a warning LED before it changes.
main.cpp
00001 #include "mbed.h" 00002 00003 DigitalOut boardLed(LED1); 00004 DigitalOut getReady(LED2); 00005 00006 DigitalOut redOne(p5); 00007 DigitalOut greenOne(p6); 00008 00009 DigitalOut redTwo(p9); 00010 DigitalOut greenTwo(p10); 00011 00012 //a traffic light 00013 00014 int main() { 00015 00016 while(1) { 00017 boardLed = 1; 00018 00019 redOne = 1; 00020 redTwo = 0; 00021 greenOne = 0; 00022 greenTwo = 1; 00023 getReady = 0; 00024 00025 wait(5); 00026 boardLed = 0; 00027 wait(5); 00028 boardLed = 1; 00029 wait(5); 00030 boardLed = 0; 00031 getReady = 1; 00032 wait(5); 00033 00034 boardLed = 1; 00035 00036 redOne = 0; 00037 redTwo = 1; 00038 greenOne = 1; 00039 greenTwo = 0; 00040 getReady = 0; 00041 00042 wait(5); 00043 boardLed = 0; 00044 wait(5); 00045 boardLed = 1; 00046 wait(5); 00047 boardLed = 0; 00048 getReady = 1; 00049 wait(5); 00050 } 00051 }
Generated on Sun Jul 17 2022 07:35:30 by
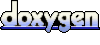