Library for Modtronix NZ32 STM32 boards, like the NZ32-SC151, NZ32-SB072, NZ32-SE411 and others
MxTick Class Reference
A general purpose milli-second and second timer. More...
#include <mx_tick.h>
Static Public Member Functions | |
static int | read_ms () |
Get the current 32-bit milli-second tick value. | |
static int | read_ms10 () |
Get the current 32-bit "10 milli-second" tick value. | |
static int | read_ms100 () |
Get the current 32-bit "100 milli-second" tick value. | |
static int | read_sec () |
Get the current 32-bit second tick value. | |
Static Protected Attributes | |
static uint16_t | countMs = 1000 |
File: mx_timer.cpp. |
Detailed Description
A general purpose milli-second and second timer.
Because the read_ms() function return a 32 bit value, it can be used to time up to a maximum of 2^31-1 millseconds = 596 Hours = 24.8 Days
Example:
// Count the time to toggle a LED #include "mbed.h" #include "mx_tick.h" MxTimer mxTmr; int tmrLED = 0; DigitalOut led(LED1); int main() { //Main loop while(1) { //Flash LED if (mxTmr.read_ms() >= tmrLED) { tmrLED += 1000; //Wait 1000mS before next LED toggle led = !led; } } }
Definition at line 57 of file mx_tick.h.
Member Function Documentation
static int read_ms | ( | ) | [static] |
static int read_ms10 | ( | ) | [static] |
Get the current 32-bit "10 milli-second" tick value.
It is incremented each 10ms. Because the read_ms() function return a 32 bit value, it can be used to time up to a maximum of 2^31-1 * 10 millseconds = 5960 Hours = 248 Days
This function is NOT thread save. Takes multiple cycles to calculate value.
Note this function takes MUCH longer than read_ms() and read_sec().
- Returns:
- The tick value in 10 x milli-seconds
static int read_ms100 | ( | ) | [static] |
Get the current 32-bit "100 milli-second" tick value.
It is incremented each 100ms. Because the read_ms() function return a 32 bit value, it can be used to time up to a maximum of 2^31-1 * 100 millseconds = 59600 Hours = 2480 Days = 6.79 Years
This function is NOT thread save. Takes multiple cycles to calculate value.
Note this function takes MUCH longer than read_ms() and read_sec().
- Returns:
- The tick value in 100 x milli-seconds
static int read_sec | ( | ) | [static] |
Get the current 32-bit second tick value.
Because the read_sec() function return a 32 bit value, it can be used to time up to a maximum of 2^31-1 seconds = 596,523 Hours = 24855 Days = 68 Years
This function is thread save!
- Returns:
- The tick value in seconds
Field Documentation
uint16_t countMs = 1000 [static, protected] |
File: mx_timer.cpp.
Author: Modtronix Engineering - www.modtronix.com
Description:
Software License Agreement: This software has been written or modified by Modtronix Engineering. The code may be modified and can be used free of charge for commercial and non commercial applications. If this is modified software, any license conditions from original software also apply. Any redistribution must include reference to 'Modtronix Engineering' and web link(www.modtronix.com) in the file header.
THIS SOFTWARE IS PROVIDED IN AN 'AS IS' CONDITION. NO WARRANTIES, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. THE COMPANY SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES, FOR ANY REASON WHATSOEVER.
Generated on Tue Jul 12 2022 15:45:26 by
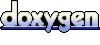