Library for Modtronix NZ32 STM32 boards, like the NZ32-SC151, NZ32-SB072, NZ32-SE411 and others
MxCmdBuffer< BufferSize, Commands, CounterType > Class Template Reference
Templated Circular buffer class. More...
#include <mx_cmd_buffer.h>
Inherits MxBuffer.
Public Member Functions | |
bool | put (const uint8_t &data) |
Adds a byte to the current command in the buffer. | |
bool | put (const char *str) |
Adds given NULL terminated string to the buffer. | |
bool | putArray (uint8_t *buf, uint16_t bufSize) |
Adds given array to the buffer. | |
uint8_t | get () |
Gets and object from the buffer. | |
bool | getAndCheck (uint8_t &data) |
Gets and object from the buffer. | |
uint8_t | peek () |
Gets and object from the buffer, but do NOT remove it. | |
uint8_t | peekAt (CounterType offset) |
Gets an object from the buffer at given offset, but do NOT remove it. | |
uint8_t | peekLastAdded () |
Gets the last object added to the buffer, but do NOT remove it. | |
uint16_t | peekArray (uint8_t *buf, uint16_t bufSize, CounterType lenReq) |
Gets and array of given size, and write it to given buffer. | |
bool | hasCommand () |
Check if the buffer has a complete command. | |
uint8_t | getCommandLength () |
Get length of next command in buffer, excluding "end of command" character! For example, the command "r=100;" will return 5. | |
uint8_t | getCommandsAvailable () |
Get number of commands waiting in buffer. | |
uint16_t | getCommandName (uint8_t *buf, uint16_t bufSize, bool &isNameValue, bool nullTerminate=true) |
If current command has "name=value" format, the 'name' part is returned. | |
uint16_t | getCommandValue (uint8_t *buf, uint16_t bufSize, uint16_t offset=-1, bool nullTerminate=true) |
For commands with "name=value" format, returns the 'value' part. | |
bool | search (uint8_t c, uint16_t *offsetFound) |
Search current command for given character. | |
bool | isEmpty () |
Check if the buffer is empty. | |
bool | isFull () |
Check if the buffer is full. | |
CounterType | getAvailable () |
Get number of available bytes in buffer. | |
CounterType | getFree () |
Get number of free bytes in buffer available for writing data to. | |
void | enableReplaceCrLfWithEoc () |
Replaces any LF or CR characters with an "End of Command" character = ';'. | |
void | disableReplaceCrLfWithEoc () |
Do NOT replaces LF and CR characters with an "End of Command" character = ';'. | |
bool | checkBufferFullError () |
Check if an overwrite error occurred. | |
void | reset () |
Reset the buffer. | |
void | removeCommand () |
Remove a command from buffer. |
Detailed Description
template<uint32_t BufferSize, uint8_t Commands = 16, typename CounterType = uint32_t>
class MxCmdBuffer< BufferSize, Commands, CounterType >
Templated Circular buffer class.
Definition at line 105 of file mx_cmd_buffer.h.
Member Function Documentation
bool checkBufferFullError | ( | ) |
Check if an overwrite error occurred.
It resets the error flag if it was set
- Returns:
- True if overwrite error occurred since last time this function was called, else false
Definition at line 627 of file mx_cmd_buffer.h.
void disableReplaceCrLfWithEoc | ( | ) |
Do NOT replaces LF and CR characters with an "End of Command" character = ';'.
Definition at line 620 of file mx_cmd_buffer.h.
void enableReplaceCrLfWithEoc | ( | ) |
Replaces any LF or CR characters with an "End of Command" character = ';'.
Definition at line 614 of file mx_cmd_buffer.h.
uint8_t get | ( | ) |
Gets and object from the buffer.
Ensure buffer is NOT empty before calling this function!
- Returns:
- Read data
Definition at line 269 of file mx_cmd_buffer.h.
bool getAndCheck | ( | uint8_t & | data ) |
Gets and object from the buffer.
Returns true if OK, else false
- Parameters:
-
data Variable to put read data into
- Returns:
- True if the buffer is not empty and data contains a transaction, false otherwise
Definition at line 285 of file mx_cmd_buffer.h.
CounterType getAvailable | ( | ) |
Get number of available bytes in buffer.
- Returns:
- Number of available bytes in buffer
Definition at line 576 of file mx_cmd_buffer.h.
uint8_t getCommandLength | ( | ) |
Get length of next command in buffer, excluding "end of command" character! For example, the command "r=100;" will return 5.
- Returns:
- Length of next command in buffer
Definition at line 365 of file mx_cmd_buffer.h.
uint16_t getCommandName | ( | uint8_t * | buf, |
uint16_t | bufSize, | ||
bool & | isNameValue, | ||
bool | nullTerminate = true |
||
) |
If current command has "name=value" format, the 'name' part is returned.
Else, the whole command is returned(excluding a possible trailing '=' character). Returned string is NULL terminated by default. Nothing is removed from the buffer!
If true is returned in the "isNameValue" parameter, this is a "name=value" command, with a name part of at lease 1 character long. The offset of the 'value' part will be the returned
If false is returned in the "isNameValue" parameter, there is no 'value' part of at least 1 character. There could however still be a '=' character with no 'value' following it. Any possible trailing '=' character is removed string returned in 'buf'.
- Parameters:
-
buf Destination buffer to write string to bufSize Maximum size to write to destination buffer isNameValue Returns true if a '=' character was found -AND- at least 1 char following it. This indicates this is a "name=value" command, and has a value part(of at least 1 character) following the '='. First character of 'value' is value returned by this function + 1!
- Returns:
- Size in bytes of string returned in 'buf' parameter(is 'name' if isNameValue=true). If true is returned in 'isNameValue', 'buf' contains the 'name' part of a 'name=value' command. This returned offset points to '='. If false is returned in 'isNameValue', 'buf' contains the whole command string(excluding possible trailing '=' character).
Definition at line 408 of file mx_cmd_buffer.h.
uint8_t getCommandsAvailable | ( | ) |
Get number of commands waiting in buffer.
- Returns:
- Number of commands waiting in buffer
Definition at line 381 of file mx_cmd_buffer.h.
uint16_t getCommandValue | ( | uint8_t * | buf, |
uint16_t | bufSize, | ||
uint16_t | offset = -1 , |
||
bool | nullTerminate = true |
||
) |
For commands with "name=value" format, returns the 'value' part.
Returned string is NULL terminated by default. Nothing is removed from the buffer!
To optimize this function, call getCommandName() before calling this function. The getCommandName() return value(+1) can be used as 'offset' parameter to this function. This will save this function from searching for '=' character.
- Parameters:
-
buf Destination buffer to write string to bufSize Maximum size to write to destination buffer offset Gives offset of value string if known. This can be value(+1) returned by getCommandName() function. Use -1 if unknown.
- Returns:
- Size in bytes of returned string
Definition at line 463 of file mx_cmd_buffer.h.
CounterType getFree | ( | ) |
Get number of free bytes in buffer available for writing data to.
- Returns:
- Number of free bytes in buffer available for writing data to.
Definition at line 597 of file mx_cmd_buffer.h.
bool hasCommand | ( | ) |
Check if the buffer has a complete command.
- Returns:
- True if the buffer has a command, false if not
Definition at line 357 of file mx_cmd_buffer.h.
bool isEmpty | ( | ) |
Check if the buffer is empty.
- Returns:
- True if the buffer is empty, false if not
Definition at line 561 of file mx_cmd_buffer.h.
bool isFull | ( | ) |
Check if the buffer is full.
- Returns:
- True if the buffer is full, false if not
Definition at line 569 of file mx_cmd_buffer.h.
uint8_t peek | ( | ) |
Gets and object from the buffer, but do NOT remove it.
Ensure buffer is NOT empty before calling this function! If buffer is empty, will return an undefined value.
- Returns:
- Read data
Definition at line 301 of file mx_cmd_buffer.h.
uint16_t peekArray | ( | uint8_t * | buf, |
uint16_t | bufSize, | ||
CounterType | lenReq | ||
) |
Gets and array of given size, and write it to given buffer.
Nothing is removed from buffer
- Parameters:
-
buf Destination buffer to array to bufSize Maximum size to write to destination buffer lenReq Requested length
- Returns:
- Number of bytes written to given buffer
Definition at line 334 of file mx_cmd_buffer.h.
uint8_t peekAt | ( | CounterType | offset ) |
Gets an object from the buffer at given offset, but do NOT remove it.
Given offset is a value from 0 to n. Ensure buffer has as many objects as the offset requested! For example, if buffer has 5 objects available, given offset can be a value from 0 to 4.
- Parameters:
-
offset Offset of requested object. A value from 0-n, where (n+1) = available objects = getAvailable()
- Returns:
- Object at given offset
Definition at line 312 of file mx_cmd_buffer.h.
uint8_t peekLastAdded | ( | ) |
Gets the last object added to the buffer, but do NOT remove it.
- Returns:
- Object at given offset
Definition at line 320 of file mx_cmd_buffer.h.
bool put | ( | const uint8_t & | data ) |
Adds a byte to the current command in the buffer.
This function checks for the "End of Command" character, and if found, increments the "command count" register indicating how many commands this buffer has.
If the buffer becomes full before the "End of Command" character is reached, all bytes added for current command are removed. And, all following bytes added until the next "End of Command" character will be ignored.
The checkBufferFullError() function can be used to check if this function caused an error, and command was not added to buffer.
- Parameters:
-
data Data to be pushed to the buffer
- Returns:
- true if character added to buffer, else false. Important to note that all characters added to current command CAN BE REMOVED if buffer gets full before "End of Command" added to buffer!
Definition at line 130 of file mx_cmd_buffer.h.
bool put | ( | const char * | str ) |
Adds given NULL terminated string to the buffer.
This function checks for the "End of Command" character, and if found, increments the "command count" register indicating how many commands this buffer has.
If the buffer becomes full before the "End of Command" character is reached, all bytes added for current command are removed. And, all following bytes added until the next "End of Command" character will be ignored.
The checkBufferFullError() function can be used to check if this function caused an error, and command was not added to buffer.
- Parameters:
-
buf Source buffer containing array to add to buffer bufSize Size of array to add to buffer
- Returns:
- Returns true if something added to buffer, else false. Important to note that all characters added to current command CAN BE REMOVED if buffer gets full before "End of Command" added to buffer!
Definition at line 217 of file mx_cmd_buffer.h.
bool putArray | ( | uint8_t * | buf, |
uint16_t | bufSize | ||
) |
Adds given array to the buffer.
This function checks for the "End of Command" character, and if found, increments the "command count" register indicating how many commands this buffer has.
If the buffer becomes full before the "End of Command" character is reached, all bytes added for current command are removed. And, all following bytes added until the next "End of Command" character will be ignored.
The checkBufferFullError() function can be used to check if this function caused an error, and command was not added to buffer.
- Parameters:
-
buf Source buffer containing array to add to buffer bufSize Size of array to add to buffer
- Returns:
- Returns true if something added to buffer, else false. Important to note that all characters added to current command CAN BE REMOVED if buffer gets full before "End of Command" added to buffer!
Definition at line 249 of file mx_cmd_buffer.h.
void removeCommand | ( | ) |
Remove a command from buffer.
Definition at line 645 of file mx_cmd_buffer.h.
void reset | ( | ) |
Reset the buffer.
Definition at line 635 of file mx_cmd_buffer.h.
bool search | ( | uint8_t | c, |
uint16_t * | offsetFound | ||
) |
Search current command for given character.
- Parameters:
-
offset Returns offset of found character. If NULL, this parameter is not used.
- Returns:
- Returns true if found, else false
Definition at line 524 of file mx_cmd_buffer.h.
Generated on Tue Jul 12 2022 15:45:26 by
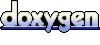