Library for Modtronix NZ32 STM32 boards, like the NZ32-SC151, NZ32-SB072, NZ32-SE411 and others
MxCircularBuffer< T, BufferSize, CounterType > Class Template Reference
Templated Circular buffer class. More...
#include <mx_circular_buffer.h>
Inherits MxBuffer.
Public Member Functions | |
bool | put (const T &data) |
Adds an object to the buffer. | |
bool | putArray (uint8_t *buf, uint16_t bufSize) |
Adds given array to the buffer. | |
T | get () |
Gets and remove and object from the buffer. | |
T | peek () |
Gets and object from the buffer, but do NOT remove it. | |
T | peekAt (CounterType offset) |
Gets an object from the buffer at given offset, but do NOT remove it. | |
T | peekLastAdded () |
Gets the last object added to the buffer, but do NOT remove it. | |
bool | getAndCheck (T &data) |
Gets and object from the buffer. | |
bool | isEmpty () |
Check if the buffer is empty. | |
bool | isFull () |
Check if the buffer is full. | |
CounterType | getAvailable () |
Get number of available bytes in buffer. | |
CounterType | getFree () |
Get number of free bytes in buffer available for writing data to. | |
void | reset () |
Reset the buffer. |
Detailed Description
template<typename T, uint32_t BufferSize, typename CounterType = uint32_t>
class MxCircularBuffer< T, BufferSize, CounterType >
Templated Circular buffer class.
Definition at line 30 of file mx_circular_buffer.h.
Member Function Documentation
T get | ( | ) |
Gets and remove and object from the buffer.
Ensure buffer is NOT empty before calling this function!
- Returns:
- Read data
Definition at line 87 of file mx_circular_buffer.h.
bool getAndCheck | ( | T & | data ) |
Gets and object from the buffer.
Returns true if OK, else false
- Parameters:
-
data Variable to put read data into
- Returns:
- True if the buffer is not empty and data contains a transaction, false otherwise
Definition at line 135 of file mx_circular_buffer.h.
CounterType getAvailable | ( | ) |
Get number of available bytes in buffer.
- Returns:
- Number of available bytes in buffer
Definition at line 167 of file mx_circular_buffer.h.
CounterType getFree | ( | ) |
Get number of free bytes in buffer available for writing data to.
- Returns:
- Number of free bytes in buffer available for writing data to.
Definition at line 188 of file mx_circular_buffer.h.
bool isEmpty | ( | ) |
Check if the buffer is empty.
- Returns:
- True if the buffer is empty, false if not
Definition at line 150 of file mx_circular_buffer.h.
bool isFull | ( | ) |
Check if the buffer is full.
- Returns:
- True if the buffer is full, false if not
Definition at line 159 of file mx_circular_buffer.h.
T peek | ( | ) |
Gets and object from the buffer, but do NOT remove it.
Ensure buffer is NOT empty before calling this function! If buffer is empty, will return an undefined value.
- Returns:
- Read data
Definition at line 104 of file mx_circular_buffer.h.
T peekAt | ( | CounterType | offset ) |
Gets an object from the buffer at given offset, but do NOT remove it.
Given offset is a value from 0 to n. Ensure buffer has as many objects as the offset requested! For example, if buffer has 5 objects available, given offset can be a value from 0 to 4.
- Parameters:
-
offset Offset of requested object. A value from 0-n, where (n+1) = available objects = getAvailable()
- Returns:
- Object at given offset
Definition at line 116 of file mx_circular_buffer.h.
T peekLastAdded | ( | ) |
Gets the last object added to the buffer, but do NOT remove it.
- Returns:
- Object at given offset
Definition at line 125 of file mx_circular_buffer.h.
bool put | ( | const T & | data ) |
Adds an object to the buffer.
If no space in buffer, this function does nothing. do anything! Use getFree() function prior to this function to ensure buffer has enough free space!
- Parameters:
-
data Data to be pushed to the buffer
- Returns:
- Returns true if character added to buffer, else false
Definition at line 47 of file mx_circular_buffer.h.
bool putArray | ( | uint8_t * | buf, |
uint16_t | bufSize | ||
) |
Adds given array to the buffer.
This function checks if buffer has enough space. If not enough space available, nothing is added, and this function returns 0.
- Parameters:
-
buf Source buffer containing array to add to buffer bufSize Size of array to add to buffer
- Returns:
- Returns true if character added to buffer, else false
Definition at line 68 of file mx_circular_buffer.h.
void reset | ( | ) |
Reset the buffer.
Definition at line 206 of file mx_circular_buffer.h.
Generated on Tue Jul 12 2022 15:45:26 by
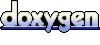