
A program to Interact with Software Driver on the PC that work as a DDE server to be used with SoftPLC\'s it turn Mbed to an I/O card to the SoftPLC
Dependencies: EthernetNetIf mbed
main.cpp
00001 00002 // MBED I/O Server FOR PC Based Control 00003 // 2011/2/7 00004 00005 00006 #include "mbed.h" 00007 #include "EthernetNetIf.h" 00008 #include "TCPSocket.h" 00009 00010 DigitalOut led4(LED4, "led4"); 00011 00012 // Declaring Digital outputs Pins 00013 DigitalOut do1(p30); 00014 DigitalOut do2(p29); 00015 DigitalOut do3(p28); 00016 DigitalOut do4(p27); 00017 DigitalOut do5(p26); 00018 DigitalOut do6(p25); 00019 DigitalOut do7(p24); 00020 DigitalOut do8(p23); 00021 DigitalOut do9(p22); 00022 DigitalOut do10(p21); 00023 // Declaring Digital Inptus Pins 00024 DigitalIn di1(p5); 00025 DigitalIn di2(p6); 00026 DigitalIn di3(p7); 00027 DigitalIn di4(p8); 00028 DigitalIn di5(p9); 00029 DigitalIn di6(p10); 00030 DigitalIn di7(p11); 00031 DigitalIn di8(p12); 00032 DigitalIn di9(p13); 00033 DigitalIn di10(p14); 00034 DigitalIn di11(p15); 00035 DigitalIn di12(p16); 00036 DigitalIn di13(p17); 00037 DigitalIn di14(p18); 00038 DigitalIn di15(p19); 00039 DigitalIn di16(p20); 00040 00041 char x[10],y[16]; 00042 00043 // EthernetNetIf eth; 00044 EthernetNetIf eth( 00045 IpAddr(192,168,1,25), //IP Address 00046 IpAddr(255,255,255,0), //Network Mask 00047 IpAddr(192,168,1,1), //Gateway 00048 IpAddr(192,168,1,1) //DNS 00049 ); 00050 00051 #define TCP_LISTENING_PORT 12345 00052 00053 TCPSocket ListeningSock; 00054 TCPSocket* pConnectedSock; // for ConnectedSock 00055 Host client; 00056 TCPSocketErr err; 00057 00058 void onConnectedTCPSocketEvent(TCPSocketEvent e) 00059 { 00060 switch(e) 00061 { 00062 case TCPSOCKET_CONNECTED: 00063 printf("TCP Socket Connected\r\n"); 00064 break; 00065 case TCPSOCKET_WRITEABLE: 00066 //Can now write some data... 00067 printf("TCP Socket Writable\r\n"); 00068 break; 00069 case TCPSOCKET_READABLE: 00070 //Can now read dome data... 00071 printf("TCP Socket Readable\r\n"); 00072 // Read in any available data into the buffer 00073 00074 //Parsing for the messege from I/O Server 00075 //1)Updating thr output status 00076 while ( pConnectedSock->recv(x, 10) ) { 00077 if(x[0]==0) 00078 { 00079 do1=0; 00080 } 00081 if(x[0]==1) 00082 { 00083 00084 do1=1; 00085 } 00086 if(x[1]==0) 00087 { 00088 00089 do2=0; 00090 } 00091 if(x[1]==1) 00092 { 00093 00094 do2=1; 00095 } 00096 if(x[2]==0) 00097 { 00098 00099 do3=0; 00100 } 00101 if(x[2]==1) 00102 { 00103 00104 do3=1; 00105 } 00106 if(x[3]==0) 00107 { 00108 do4=0; 00109 } 00110 if(x[3]==1) 00111 { 00112 do4=1; 00113 } 00114 if(x[4]==0) 00115 { 00116 do5=0; 00117 } 00118 if(x[4]==1) 00119 { 00120 do5=1; 00121 } 00122 if(x[5]==0) 00123 { 00124 do6=0; 00125 } 00126 if(x[5]==1) 00127 { 00128 do6=1; 00129 } 00130 if(x[6]==0) 00131 { 00132 do7=0; 00133 } 00134 if(x[6]==1) 00135 { 00136 do7=1; 00137 } 00138 if(x[7]==0) 00139 { 00140 do8=0; 00141 } 00142 if(x[7]==1) 00143 { 00144 do8=1; 00145 } 00146 if(x[8]==0) 00147 { 00148 do9=0; 00149 } 00150 if(x[8]==1) 00151 { 00152 do9=1; 00153 } 00154 if(x[9]==0) 00155 { 00156 do10=0; 00157 } 00158 if(x[9]==1) 00159 { 00160 do10=1; 00161 } 00162 00163 00164 00165 // 2) send the inputs status to I/O Server 00166 if (di1==1) 00167 { 00168 y[0]=1; 00169 } 00170 if (di1==0) 00171 { 00172 y[0]=0; 00173 } 00174 if (di2==1) 00175 { 00176 y[1]=1; 00177 } 00178 if (di2==0) 00179 { 00180 y[1]=0; 00181 } 00182 if (di3==1) 00183 { 00184 y[2]=1; 00185 } 00186 if (di3==0) 00187 { 00188 y[2]=0; 00189 } 00190 if (di4==1) 00191 { 00192 y[3]=1; 00193 } 00194 if (di4==0) 00195 { 00196 y[3]=0; 00197 } 00198 if (di1==5) 00199 { 00200 y[4]=1; 00201 } 00202 if (di5==0) 00203 { 00204 y[4]=0; 00205 } 00206 if (di6==1) 00207 { 00208 y[5]=1; 00209 } 00210 if (di6==0) 00211 { 00212 y[5]=0; 00213 } 00214 if (di7==1) 00215 { 00216 y[6]=1; 00217 } 00218 if (di7==0) 00219 { 00220 y[6]=0; 00221 } 00222 if (di8==1) 00223 { 00224 y[7]=1; 00225 } 00226 if (di8==0) 00227 { 00228 y[7]=0; 00229 } 00230 if (di9==1) 00231 { 00232 y[8]=1; 00233 } 00234 if (di9==0) 00235 { 00236 y[8]=0; 00237 } 00238 if (di10==1) 00239 { 00240 y[9]=1; 00241 } 00242 if (di10==0) 00243 { 00244 y[9]=0; 00245 } 00246 if (di11==1) 00247 { 00248 y[10]=1; 00249 } 00250 if (di11==0) 00251 { 00252 y[10]=0; 00253 } 00254 if (di12==1) 00255 { 00256 y[11]=1; 00257 } 00258 if (di12==0) 00259 { 00260 y[11]=0; 00261 } 00262 if (di13==1) 00263 { 00264 y[12]=1; 00265 } 00266 if (di13==0) 00267 { 00268 y[12]=0; 00269 } 00270 if (di14==1) 00271 { 00272 y[13]=1; 00273 } 00274 if (di14==0) 00275 { 00276 y[13]=0; 00277 } 00278 if (di15==1) 00279 { 00280 y[14]=1; 00281 } 00282 if (di15==0) 00283 { 00284 y[14]=0; 00285 } 00286 if (di16==1) 00287 { 00288 y[15]=1; 00289 } 00290 if (di16==0) 00291 { 00292 y[15]=0; 00293 } 00294 00295 00296 pConnectedSock->send(y, 16); 00297 // make terminater 00298 00299 } 00300 break; 00301 case TCPSOCKET_CONTIMEOUT: 00302 printf("TCP Socket Timeout\r\n"); 00303 break; 00304 case TCPSOCKET_CONRST: 00305 printf("TCP Socket CONRST\r\n"); 00306 break; 00307 case TCPSOCKET_CONABRT: 00308 printf("TCP Socket CONABRT\r\n"); 00309 break; 00310 case TCPSOCKET_ERROR: 00311 printf("TCP Socket Error\r\n"); 00312 break; 00313 case TCPSOCKET_DISCONNECTED: 00314 //Close socket... 00315 printf("TCP Socket Disconnected\r\n"); 00316 pConnectedSock->close(); 00317 break; 00318 default: 00319 printf("DEFAULT\r\n"); 00320 } 00321 } 00322 00323 00324 void onListeningTCPSocketEvent(TCPSocketEvent e) 00325 { 00326 switch(e) 00327 { 00328 case TCPSOCKET_ACCEPT: 00329 printf("Listening: TCP Socket Accepted\r\n"); 00330 // Accepts connection from client and gets connected socket. 00331 err=ListeningSock.accept(&client, &pConnectedSock); 00332 if (err) { 00333 printf("onListeningTcpSocketEvent : Could not accept connection.\r\n"); 00334 return; //Error in accept, discard connection 00335 } 00336 // Setup the new socket events 00337 pConnectedSock->setOnEvent(&onConnectedTCPSocketEvent); 00338 // We can find out from where the connection is coming by looking at the 00339 // Host parameter of the accept() method 00340 IpAddr clientIp = client.getIp(); 00341 printf("Listening: Incoming TCP connection from %d.%d.%d.%d\r\n", 00342 clientIp[0], clientIp[1], clientIp[2], clientIp[3]); 00343 break; 00344 // the following cases will not happen 00345 case TCPSOCKET_CONNECTED: 00346 printf("Listening: TCP Socket Connected\r\n"); 00347 break; 00348 case TCPSOCKET_WRITEABLE: 00349 printf("Listening: TCP Socket Writable\r\n"); 00350 break; 00351 case TCPSOCKET_READABLE: 00352 printf("Listening: TCP Socket Readable\r\n"); 00353 break; 00354 case TCPSOCKET_CONTIMEOUT: 00355 printf("Listening: TCP Socket Timeout\r\n"); 00356 break; 00357 case TCPSOCKET_CONRST: 00358 printf("Listening: TCP Socket CONRST\r\n"); 00359 break; 00360 case TCPSOCKET_CONABRT: 00361 printf("Listening: TCP Socket CONABRT\r\n"); 00362 break; 00363 case TCPSOCKET_ERROR: 00364 printf("Listening: TCP Socket Error\r\n"); 00365 break; 00366 case TCPSOCKET_DISCONNECTED: 00367 //Close socket... 00368 printf("Listening: TCP Socket Disconnected\r\n"); 00369 ListeningSock.close(); 00370 break; 00371 default: 00372 printf("DEFAULT\r\n"); 00373 }; 00374 } 00375 00376 00377 int main() { 00378 printf("\r\n"); 00379 printf("Setting up...\r\n"); 00380 EthernetErr ethErr = eth.setup(); 00381 if(ethErr) 00382 { 00383 printf("Error %d in setup.\r\n", ethErr); 00384 return -1; 00385 } 00386 printf("Setup OK\r\n"); 00387 00388 IpAddr ip = eth.getIp(); 00389 printf("mbed IP Address is %d.%d.%d.%d\r\n", ip[0], ip[1], ip[2], ip[3]); 00390 00391 00392 // Set the callbacks for Listening 00393 ListeningSock.setOnEvent(&onListeningTCPSocketEvent); 00394 00395 // bind and listen on TCP 00396 err=ListeningSock.bind(Host(IpAddr(), TCP_LISTENING_PORT)); 00397 printf("Binding..\r\n"); 00398 if(err) 00399 { 00400 //Deal with that error... 00401 printf("Binding Error\n"); 00402 } 00403 err=ListeningSock.listen(); // Starts listening 00404 printf("Listening...\r\n"); 00405 if(err) 00406 { 00407 printf("Listening Error\r\n"); 00408 } 00409 00410 Timer tmr; 00411 tmr.start(); 00412 00413 while(true) 00414 { 00415 Net::poll(); 00416 if(tmr.read() > 0.2) // sec 00417 { 00418 led4=!led4; //Show that we are alive 00419 tmr.reset(); 00420 } 00421 } 00422 }
Generated on Wed Sep 14 2022 05:50:03 by
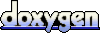