
A scripting environment used to define precise output/input temporal relationships.
Dependencies: SMARTWAV mbed HelloWorld
Dependents: perturbRoom_legacy
Fork of HelloWorld by
soundControl.cpp
00001 #include "soundControl.h" 00002 00003 extern SMARTWAV sWav; 00004 extern Serial pc; 00005 00006 soundControl::soundControl(void): 00007 fileNameExists(false), 00008 volumePtr(NULL), 00009 volume(-1), 00010 play(true), 00011 reset(false) { 00012 00013 } 00014 00015 void soundControl::setFile(string fileNameIn) { 00016 for (int i = 0; i < 20; i++) { 00017 fileName[i] = NULL; 00018 } 00019 std::size_t length = fileNameIn.size(); 00020 if (length <= 20) { 00021 fileNameIn.copy(fileName, length, 0); 00022 fileNameExists = true; 00023 } 00024 } 00025 void soundControl::setVolume(int volumeIn) { 00026 00027 if ((volumeIn >= 0) && (volumeIn < 256)) { 00028 volume = volumeIn; 00029 volumePtr = NULL; 00030 } 00031 } 00032 00033 void soundControl::setVolume(int* volumeIn) { 00034 00035 volume = -1; 00036 volumePtr = volumeIn; 00037 00038 } 00039 00040 void soundControl::setPlayback(bool playIn) { 00041 play = playIn; 00042 } 00043 00044 void soundControl::setReset() { 00045 reset = true; 00046 } 00047 00048 void soundControl::execute() { 00049 00050 if (reset) { 00051 sWav.reset(); 00052 } else if (!play) { 00053 sWav.stopTrack(); 00054 } else { 00055 if (volume > -1) { 00056 sWav.volume(volume); 00057 } else if (volumePtr != NULL) { 00058 sWav.volume(*volumePtr); 00059 } 00060 00061 if (fileNameExists) { 00062 //sWav.playTracks(); 00063 sWav.stopTrack(); 00064 sWav.playTrackName(fileName); 00065 00066 00067 00068 } 00069 } 00070 } 00071 00072 00073
Generated on Sun Jul 17 2022 23:34:35 by
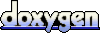