fork of smartwav interface
Fork of SMARTWAV by
Embed:
(wiki syntax)
Show/hide line numbers
SMARTWAV.cpp
00001 /********************************************************* 00002 VIZIC TECHNOLOGIES. COPYRIGHT 2012. 00003 THE DATASHEETS, SOFTWARE AND LIBRARIES ARE PROVIDED "AS IS." 00004 VIZIC EXPRESSLY DISCLAIM ANY WARRANTY OF ANY KIND, WHETHER 00005 EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO, THE IMPLIED 00006 WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE, 00007 OR NONINFRINGEMENT. IN NO EVENT SHALL VIZIC BE LIABLE FOR 00008 ANY INCIDENTAL, SPECIAL, INDIRECT OR CONSEQUENTIAL DAMAGES, 00009 LOST PROFITS OR LOST DATA, HARM TO YOUR EQUIPMENT, COST OF 00010 PROCUREMENT OF SUBSTITUTE GOODS, TECHNOLOGY OR SERVICES, 00011 ANY CLAIMS BY THIRD PARTIES (INCLUDING BUT NOT LIMITED TO 00012 ANY DEFENCE THEREOF), ANY CLAIMS FOR INDEMNITY OR CONTRIBUTION, 00013 OR OTHER SIMILAR COSTS. 00014 *********************************************************/ 00015 00016 /******************************************************** 00017 IMPORTANT : This library is created for the mbed Microcontroller Software IDE 00018 ********************************************************/ 00019 00020 00021 #include "mbed.h" 00022 #include "SMARTWAV.h" 00023 00024 // SMART WAV DEFAULT BAUD RATE: 9600bps 00025 //It shoud be used like this : SMARTWAV audio(p13,p14,p15); for serial communication with SMARTWAV 00026 SMARTWAV::SMARTWAV(PinName TXPin, PinName RXPin, PinName resetPin): _serialSMARTWAV(TXPin,RXPin), _resetPin(resetPin){ 00027 init(); 00028 } 00029 00030 /********** high level commands, for the user! */ 00031 void SMARTWAV::init(){ //configure the mbed for SMARTWAV board 00032 _serialSMARTWAV.baud(9600); 00033 _resetPin=1; //set the pin to 3.3v to avoid reset 00034 } 00035 00036 void SMARTWAV::reset(){ //Reset the SMARTWAV board 00037 _resetPin=0; //set the pin to GND to reset 00038 wait_ms(500); 00039 _resetPin=1; //set the pin to 3.3v to end reset 00040 wait_ms(500); 00041 } 00042 00043 unsigned char SMARTWAV::sleep(){ //Send SMARTWAV to sleep mode / awake from sleep mode 00044 _serialSMARTWAV.putc('Z'); 00045 return _serialSMARTWAV.getc(); 00046 } 00047 00048 unsigned char SMARTWAV::getStatus(){ //Check active status 00049 unsigned char status; 00050 00051 _serialSMARTWAV.putc('A'); 00052 status = _serialSMARTWAV.getc(); 00053 _serialSMARTWAV.getc(); 00054 return status; 00055 } 00056 00057 unsigned char SMARTWAV::playTracks(){ //Start playing any song on current Folder/Dir 00058 _serialSMARTWAV.putc('T'); 00059 return _serialSMARTWAV.getc(); 00060 } 00061 00062 unsigned char SMARTWAV::pausePlay(){ //Pause / play song 00063 _serialSMARTWAV.putc('P'); 00064 return _serialSMARTWAV.getc(); 00065 } 00066 00067 unsigned char SMARTWAV::rewindTrack(){ //Rewind Track 00068 _serialSMARTWAV.putc('R'); 00069 return _serialSMARTWAV.getc(); 00070 } 00071 00072 unsigned char SMARTWAV::nextTrack(){ //Next Track 00073 _serialSMARTWAV.putc('N'); 00074 return _serialSMARTWAV.getc(); 00075 } 00076 00077 unsigned char SMARTWAV::playTrackName(char name[]){ //play an Audio file contained on the micro SD card with the given name. 00078 unsigned char counter=0; 00079 00080 //if (_serialSMARTWAV.writeable()) { 00081 _serialSMARTWAV.putc('F'); 00082 //} 00083 while(1){ 00084 //if (_serialSMARTWAV.writeable()) { 00085 _serialSMARTWAV.putc(name[counter]); 00086 //} else { 00087 // break; 00088 //} 00089 if(name[counter]==0x00){ 00090 break; 00091 } 00092 counter++; 00093 } 00094 00095 return _serialSMARTWAV.getc(); 00096 //return 1; 00097 } 00098 00099 unsigned char SMARTWAV::stopTrack(){ //Stop playing any active song 00100 00101 //if (_serialSMARTWAV.writeable()) { 00102 _serialSMARTWAV.putc('S'); 00103 //} 00104 return _serialSMARTWAV.getc(); 00105 //return 1; 00106 } 00107 00108 unsigned char SMARTWAV::continuousPlay(unsigned char enable){ //Enable/Disable continuous play, also disabled by calling stopTrack() 00109 unsigned char status; 00110 00111 _serialSMARTWAV.putc('C'); 00112 _serialSMARTWAV.putc(enable); 00113 status = _serialSMARTWAV.getc(); 00114 _serialSMARTWAV.getc(); 00115 return status; 00116 } 00117 00118 unsigned char SMARTWAV::volume(unsigned char vol){ //Set Volume 0-255 00119 _serialSMARTWAV.putc('V'); 00120 _serialSMARTWAV.putc(vol); 00121 //return _serialSMARTWAV.getc(); 00122 return 1; 00123 } 00124 00125 unsigned char SMARTWAV::setFolder(char name[]){ //Set/Enters inside a folder/path on the micro SD card with the given name. 00126 unsigned char counter=0; 00127 00128 _serialSMARTWAV.putc('D'); 00129 while(1){ 00130 _serialSMARTWAV.putc(name[counter]); 00131 if(name[counter]==0x00){ 00132 break; 00133 } 00134 counter++; 00135 } 00136 return _serialSMARTWAV.getc(); 00137 } 00138 00139 unsigned char SMARTWAV::getFileName(char name[]){ //Reads the name of the current/ last audio file being played on the SMARTWAV, and stores it on the name[] buffer. 00140 unsigned int i=0; 00141 00142 _serialSMARTWAV.putc('I'); 00143 _serialSMARTWAV.putc('S'); 00144 //receive all the file name 00145 while(1){ 00146 name[i]=_serialSMARTWAV.getc(); 00147 if(name[i]==0x00){ 00148 break; 00149 } 00150 i++; 00151 } 00152 return _serialSMARTWAV.getc(); 00153 } 00154 00155 unsigned char SMARTWAV::getFolderName(char name[]){ //Reads the name of the current folderDir/path name the SMARTWAV, and stores it on the name[] buffer. 00156 unsigned int i=0; 00157 00158 _serialSMARTWAV.putc('I'); 00159 _serialSMARTWAV.putc('D'); 00160 //receive all the folder/path name 00161 while(1){ 00162 name[i]=_serialSMARTWAV.getc(); 00163 if(name[i]==0x00){ 00164 break; 00165 } 00166 i++; 00167 } 00168 return _serialSMARTWAV.getc(); 00169 } 00170 00171 unsigned char SMARTWAV::getFileList(char list[]){ //Reads all the names of the .WAV files on the current folder/dir on the SMARTWAV, and stores it on the list[] buffer separated by comma ','. 00172 unsigned int i=0; 00173 00174 _serialSMARTWAV.putc('I'); 00175 _serialSMARTWAV.putc('L'); 00176 //receive all the file names 00177 while(1){ 00178 list[i]=_serialSMARTWAV.getc(); 00179 if(list[i]==0x00){ 00180 break; 00181 } 00182 i++; 00183 } 00184 return _serialSMARTWAV.getc(); 00185 } 00186 00187 unsigned char SMARTWAV::getFolderList(char list[]){ //Reads all the folders on the current folder/dir on the SMARTWAV, and stores it on the list[] buffer separated by comma ','. 00188 unsigned int i=0; 00189 00190 _serialSMARTWAV.putc('I'); 00191 _serialSMARTWAV.putc('X'); 00192 //receive all the folder names 00193 while(1){ 00194 list[i]=_serialSMARTWAV.getc(); 00195 if(list[i]==0x00){ 00196 break; 00197 } 00198 i++; 00199 } 00200 return _serialSMARTWAV.getc(); 00201 } 00202 00203 unsigned char SMARTWAV::playSpeed(unsigned char speed){ //Change current Play Speed: X0.5, X1, X1.5, X2, if track ends, play speed returns to 1.0X 00204 00205 _serialSMARTWAV.putc('M'); 00206 _serialSMARTWAV.putc(speed); 00207 _serialSMARTWAV.getc(); 00208 return _serialSMARTWAV.getc(); 00209 } 00210
Generated on Thu Jul 14 2022 01:13:04 by
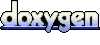