succes
Embed:
(wiki syntax)
Show/hide line numbers
motor.cpp
00001 #include "mbed.h" 00002 #include "motor.h" 00003 00004 00005 Motor::Motor(MicroBit & bit) 00006 : _bit(bit), 00007 _speed(0), 00008 _targetSpeed(0) 00009 { 00010 // 50% duty cycle will make a nice step pulse 00011 SetPWMDutyCycle(900); 00012 } 00013 00014 void Motor::SetSpeed(float targetSpeed) 00015 { 00016 _targetSpeed = targetSpeed; 00017 } 00018 00019 float Motor::Speed() 00020 { 00021 return _speed; 00022 } 00023 00024 void Motor::Step() 00025 { 00026 if ((_speed - _targetSpeed) > ACCELERATION) 00027 _speed -= ACCELERATION; 00028 else if ((_speed - _targetSpeed) < -ACCELERATION) 00029 _speed += ACCELERATION; 00030 else 00031 _speed = _targetSpeed; 00032 00033 if ((_speed < 0.00005) && (_speed > -0.00005)) 00034 _speed = 0.00005; // Prevent int overflow in SetPWMPeriod. 00035 00036 SetPWMPeriod(abs(100000/_speed)); 00037 } 00038 00039 void Motor::SetPWMDutyCycle(int value) const 00040 { 00041 _bit.io.P3.setAnalogValue(value); 00042 _bit.io.P4.setAnalogValue(value); 00043 } 00044 00045 void Motor::SetPWMPeriod(int usValue) const 00046 { 00047 _bit.io.P3.setAnalogPeriodUs(usValue); 00048 _bit.io.P4.setAnalogPeriodUs(usValue); 00049 } 00050 00051 void Motor::SetDirection(STEP_DIRECTION direction) const 00052 { 00053 _bit.io.P8.setDigitalValue(direction); 00054 _bit.io.P16.setDigitalValue(direction); 00055 } 00056
Generated on Wed Jul 13 2022 09:29:34 by
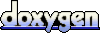