
An I/O controller for virtual pinball machines: accelerometer nudge sensing, analog plunger input, button input encoding, LedWiz compatible output controls, and more.
Dependencies: mbed FastIO FastPWM USBDevice
Fork of Pinscape_Controller by
MMA8451Q Class Reference
MMA8451Q accelerometer example. More...
#include <MMA8451Q.h>
Public Member Functions | |
MMA8451Q (PinName sda, PinName scl, int addr) | |
MMA8451Q constructor. | |
~MMA8451Q () | |
MMA8451Q destructor. | |
void | init () |
Reset the accelerometer hardware and set our initial parameters. | |
void | standby () |
Enter standby mode. | |
void | active () |
Enter active mode. | |
uint8_t | getWhoAmI () |
Get the value of the WHO_AM_I register. | |
float | getAccX () |
Get X axis acceleration. | |
float | getAccY () |
Get Y axis acceleration. | |
void | getAccXY (float &x, float &y) |
Read an X,Y pair. | |
void | getAccXYZ (float &x, float &y, float &z) |
Read X,Y,Z as floats. | |
void | getAccXYZ (int &x, int &y, int &z) |
Read X,Y,Z as integers. | |
float | getAccZ () |
Get Z axis acceleration. | |
void | getAccAllAxis (float *res) |
Get XYZ axis acceleration. | |
void | setInterruptMode (int pin) |
Set interrupt mode. | |
void | setRange (int g) |
Set the hardware dynamic range, in G. | |
void | clearInterruptMode () |
Disable interrupts. | |
bool | sampleReady () |
Is a sample ready? | |
int | getFIFOCount () |
Get the number of FIFO samples available. |
Detailed Description
MMA8451Q accelerometer example.
#include "mbed.h" #include "MMA8451Q.h" #define MMA8451_I2C_ADDRESS (0x1d<<1) int main(void) { MMA8451Q acc(P_E25, P_E24, MMA8451_I2C_ADDRESS); PwmOut rled(LED_RED); PwmOut gled(LED_GREEN); PwmOut bled(LED_BLUE); while (true) { rled = 1.0 - abs(acc.getAccX()); gled = 1.0 - abs(acc.getAccY()); bled = 1.0 - abs(acc.getAccZ()); wait(0.1); } }
Definition at line 49 of file MMA8451Q.h.
Constructor & Destructor Documentation
MMA8451Q | ( | PinName | sda, |
PinName | scl, | ||
int | addr | ||
) |
MMA8451Q constructor.
- Parameters:
-
sda SDA pin sdl SCL pin addr addr of the I2C peripheral
Definition at line 89 of file MMA8451Q.cpp.
~MMA8451Q | ( | ) |
MMA8451Q destructor.
Definition at line 150 of file MMA8451Q.cpp.
Member Function Documentation
void active | ( | ) |
Enter active mode.
Definition at line 246 of file MMA8451Q.cpp.
void clearInterruptMode | ( | ) |
Disable interrupts.
Definition at line 197 of file MMA8451Q.cpp.
void getAccAllAxis | ( | float * | res ) |
Get XYZ axis acceleration.
- Parameters:
-
res array where acceleration data will be stored
Definition at line 321 of file MMA8451Q.cpp.
float getAccX | ( | ) |
void getAccXY | ( | float & | x, |
float & | y | ||
) |
Read an X,Y pair.
Definition at line 267 of file MMA8451Q.cpp.
void getAccXYZ | ( | float & | x, |
float & | y, | ||
float & | z | ||
) |
Read X,Y,Z as floats.
This is the second most efficient way to fetch all three axes (after the integer version), since it fetches all axes in a single I2C transaction.
Definition at line 282 of file MMA8451Q.cpp.
void getAccXYZ | ( | int & | x, |
int & | y, | ||
int & | z | ||
) |
Read X,Y,Z as integers.
This reads the three axes in a single I2C transaction and returns them in the native integer scale, so it's the most efficient way to read the current 3D status. Each axis value is represented an an integer using the device's native 14-bit scale, so each is in the range -8192..+8191.
Definition at line 301 of file MMA8451Q.cpp.
float getAccY | ( | ) |
float getAccZ | ( | ) |
int getFIFOCount | ( | ) |
Get the number of FIFO samples available.
Definition at line 159 of file MMA8451Q.cpp.
uint8_t getWhoAmI | ( | ) |
Get the value of the WHO_AM_I register.
- Returns:
- WHO_AM_I value
Definition at line 257 of file MMA8451Q.cpp.
void init | ( | ) |
Reset the accelerometer hardware and set our initial parameters.
Definition at line 99 of file MMA8451Q.cpp.
bool sampleReady | ( | ) |
Is a sample ready?
Definition at line 152 of file MMA8451Q.cpp.
void setInterruptMode | ( | int | pin ) |
Set interrupt mode.
'pin' is 1 for INT1_ACCEL (PTA14) and 2 for INT2_ACCEL (PTA15). The caller is responsible for setting up an interrupt handler on the corresponding PTAxx pin.
Definition at line 166 of file MMA8451Q.cpp.
void setRange | ( | int | g ) |
Set the hardware dynamic range, in G.
Valid ranges are 2, 4, and 8.
Definition at line 210 of file MMA8451Q.cpp.
void standby | ( | ) |
Enter standby mode.
Definition at line 230 of file MMA8451Q.cpp.
Generated on Wed Jul 13 2022 03:30:11 by
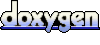