
An input/output controller for virtual pinball machines, with plunger position tracking, accelerometer-based nudge sensing, button input encoding, and feedback device control.
Dependencies: USBDevice mbed FastAnalogIn FastIO FastPWM SimpleDMA
USBJoystick Class Reference
USBJoystick example. More...
#include <USBJoystick.h>
Inherited by MyUSBJoystick.
Public Member Functions | |
USBJoystick (uint16_t vendor_id=0x1234, uint16_t product_id=0x0100, uint16_t product_release=0x0001, int waitForConnect=true) | |
Constructor. | |
bool | update (int16_t x, int16_t y, int16_t z, uint32_t buttons, uint16_t status) |
Write a state of the mouse. | |
bool | updateStatus (uint32_t stat) |
Update just the status. | |
bool | updateExposure (int &idx, int npix, const uint16_t *pix) |
Write an exposure report. | |
bool | reportConfig (int numOutputs, int unitNo) |
Write a configuration report. | |
bool | update () |
Write a state of the mouse. | |
bool | move (int16_t x, int16_t y) |
Move the cursor to (x, y) | |
bool | setZ (int16_t z) |
Set the z position. | |
bool | buttons (uint32_t buttons) |
Press one or several buttons. |
Detailed Description
USBJoystick example.
#include "mbed.h" #include "USBJoystick.h" USBJoystick joystick; int main(void) { while (1) { joystick.move(20, 0); wait(0.5); } }
#include "mbed.h" #include "USBJoystick.h" #include <math.h> USBJoystick joystick; int main(void) { while (1) { // Basic Joystick joystick.update(tx, y, z, buttonBits); wait(0.001); } }
Definition at line 83 of file USBJoystick.h.
Constructor & Destructor Documentation
USBJoystick | ( | uint16_t | vendor_id = 0x1234 , |
uint16_t | product_id = 0x0100 , |
||
uint16_t | product_release = 0x0001 , |
||
int | waitForConnect = true |
||
) |
Constructor.
- Parameters:
-
vendor_id Your vendor_id (default: 0x1234) product_id Your product_id (default: 0x0002) product_release Your product_release (default: 0x0001)
Definition at line 93 of file USBJoystick.h.
Member Function Documentation
bool buttons | ( | uint32_t | buttons ) |
Press one or several buttons.
- Parameters:
-
buttons button state, as a bitwise combination of JOY_Bn values
- Returns:
- true if there is no error, false otherwise
Definition at line 132 of file USBJoystick.cpp.
bool move | ( | int16_t | x, |
int16_t | y | ||
) |
Move the cursor to (x, y)
- Parameters:
-
x x-axis position y y-axis position
- Returns:
- true if there is no error, false otherwise
Definition at line 119 of file USBJoystick.cpp.
bool reportConfig | ( | int | numOutputs, |
int | unitNo | ||
) |
Write a configuration report.
- Parameters:
-
numOutputs the number of configured output channels unitNo the device unit number
Definition at line 97 of file USBJoystick.cpp.
bool setZ | ( | int16_t | z ) |
bool update | ( | ) |
Write a state of the mouse.
- Returns:
- true if there is no error, false otherwise
Definition at line 43 of file USBJoystick.cpp.
bool update | ( | int16_t | x, |
int16_t | y, | ||
int16_t | z, | ||
uint32_t | buttons, | ||
uint16_t | status | ||
) |
Write a state of the mouse.
- Parameters:
-
x x-axis position y y-axis position z z-axis position buttons buttons state, as a bit mask (combination with '|' of JOY_Bn values)
- Returns:
- true if there is no error, false otherwise
Definition at line 30 of file USBJoystick.cpp.
bool updateExposure | ( | int & | idx, |
int | npix, | ||
const uint16_t * | pix | ||
) |
Write an exposure report.
We'll fill out a report with as many pixels as will fit in the packet, send the report, and update the index to the next pixel to send. The caller should call this repeatedly to send reports for all pixels.
- Parameters:
-
idx current index in pixel array, updated to point to next pixel to send npix number of pixels in the overall array pix pixel array
Definition at line 65 of file USBJoystick.cpp.
bool updateStatus | ( | uint32_t | stat ) |
Update just the status.
Definition at line 141 of file USBJoystick.cpp.
Generated on Fri Jul 15 2022 08:43:32 by
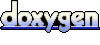