project
Fork of X_NUCLEO_IDB0XA1 by
Embed:
(wiki syntax)
Show/hide line numbers
bluenrg_hal_aci.c
00001 /******************** (C) COPYRIGHT 2013 STMicroelectronics ******************** 00002 * File Name : bluenrg_hci.c 00003 * Author : AMS - HEA&RF BU 00004 * Version : V1.0.0 00005 * Date : 4-Oct-2013 00006 * Description : File with HCI commands for BlueNRG FW6.0 and above. 00007 ******************************************************************************** 00008 * THE PRESENT FIRMWARE WHICH IS FOR GUIDANCE ONLY AIMS AT PROVIDING CUSTOMERS 00009 * WITH CODING INFORMATION REGARDING THEIR PRODUCTS IN ORDER FOR THEM TO SAVE TIME. 00010 * AS A RESULT, STMICROELECTRONICS SHALL NOT BE HELD LIABLE FOR ANY DIRECT, 00011 * INDIRECT OR CONSEQUENTIAL DAMAGES WITH RESPECT TO ANY CLAIMS ARISING FROM THE 00012 * CONTENT OF SUCH FIRMWARE AND/OR THE USE MADE BY CUSTOMERS OF THE CODING 00013 * INFORMATION CONTAINED HEREIN IN CONNECTION WITH THEIR PRODUCTS. 00014 *******************************************************************************/ 00015 00016 #include "hal_types.h" 00017 #include "osal.h" 00018 #include "ble_status.h" 00019 #include "hal.h" 00020 #include "osal.h" 00021 #include "hci_const.h" 00022 #include "bluenrg_aci_const.h" 00023 #include "bluenrg_hal_aci.h" 00024 #include "bluenrg_gatt_server.h" 00025 #include "bluenrg_gap.h" 00026 00027 #define MIN(a,b) ((a) < (b) )? (a) : (b) 00028 #define MAX(a,b) ((a) > (b) )? (a) : (b) 00029 00030 00031 tBleStatus aci_hal_write_config_data(uint8_t offset, 00032 uint8_t len, 00033 const uint8_t *val) 00034 { 00035 struct hci_request rq; 00036 uint8_t status; 00037 uint8_t buffer[HCI_MAX_PAYLOAD_SIZE]; 00038 uint8_t indx = 0; 00039 00040 if ((len+2) > HCI_MAX_PAYLOAD_SIZE) 00041 return BLE_STATUS_INVALID_PARAMS; 00042 00043 buffer[indx] = offset; 00044 indx++; 00045 00046 buffer[indx] = len; 00047 indx++; 00048 00049 Osal_MemCpy(buffer + indx, val, len); 00050 indx += len; 00051 00052 Osal_MemSet(&rq, 0, sizeof(rq)); 00053 rq.ogf = OGF_VENDOR_CMD; 00054 rq.ocf = OCF_HAL_WRITE_CONFIG_DATA; 00055 rq.cparam = (void *)buffer; 00056 rq.clen = indx; 00057 rq.rparam = &status; 00058 rq.rlen = 1; 00059 00060 if (hci_send_req(&rq, FALSE) < 0) 00061 return BLE_STATUS_TIMEOUT; 00062 00063 if (status) { 00064 return status; 00065 } 00066 00067 return 0; 00068 } 00069 00070 tBleStatus aci_hal_set_tx_power_level(uint8_t en_high_power, uint8_t pa_level) 00071 { 00072 struct hci_request rq; 00073 hal_set_tx_power_level_cp cp; 00074 uint8_t status; 00075 00076 cp.en_high_power = en_high_power; 00077 cp.pa_level = pa_level; 00078 00079 Osal_MemSet(&rq, 0, sizeof(rq)); 00080 rq.ogf = OGF_VENDOR_CMD; 00081 rq.ocf = OCF_HAL_SET_TX_POWER_LEVEL; 00082 rq.cparam = &cp; 00083 rq.clen = HAL_SET_TX_POWER_LEVEL_CP_SIZE; 00084 rq.rparam = &status; 00085 rq.rlen = 1; 00086 00087 if (hci_send_req(&rq, FALSE) < 0) 00088 return BLE_STATUS_TIMEOUT; 00089 00090 if (status) { 00091 return status; 00092 } 00093 00094 return 0; 00095 } 00096 00097 tBleStatus aci_hal_device_standby(void) 00098 { 00099 struct hci_request rq; 00100 uint8_t status; 00101 00102 Osal_MemSet(&rq, 0, sizeof(rq)); 00103 rq.ogf = OGF_VENDOR_CMD; 00104 rq.ocf = OCF_HAL_DEVICE_STANDBY; 00105 rq.rparam = &status; 00106 rq.rlen = 1; 00107 00108 if (hci_send_req(&rq, FALSE) < 0) 00109 return BLE_STATUS_TIMEOUT; 00110 00111 return status; 00112 } 00113 00114 tBleStatus aci_hal_tone_start(uint8_t rf_channel) 00115 { 00116 struct hci_request rq; 00117 hal_tone_start_cp cp; 00118 uint8_t status; 00119 00120 cp.rf_channel = rf_channel; 00121 00122 Osal_MemSet(&rq, 0, sizeof(rq)); 00123 rq.ogf = OGF_VENDOR_CMD; 00124 rq.ocf = OCF_HAL_TONE_START; 00125 rq.cparam = &cp; 00126 rq.clen = HAL_TONE_START_CP_SIZE; 00127 rq.rparam = &status; 00128 rq.rlen = 1; 00129 00130 if (hci_send_req(&rq, FALSE) < 0) 00131 return BLE_STATUS_TIMEOUT; 00132 00133 return status; 00134 } 00135 00136 tBleStatus aci_hal_tone_stop(void) 00137 { 00138 struct hci_request rq; 00139 uint8_t status; 00140 00141 Osal_MemSet(&rq, 0, sizeof(rq)); 00142 rq.ogf = OGF_VENDOR_CMD; 00143 rq.ocf = OCF_HAL_TONE_STOP; 00144 rq.rparam = &status; 00145 rq.rlen = 1; 00146 00147 if (hci_send_req(&rq, FALSE) < 0) 00148 return BLE_STATUS_TIMEOUT; 00149 00150 return status; 00151 } 00152 00153 00154
Generated on Tue Jul 12 2022 19:31:15 by
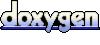