project
Fork of X_NUCLEO_IDB0XA1 by
Embed:
(wiki syntax)
Show/hide line numbers
BlueNRGGap.h
Go to the documentation of this file.
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 /** 00018 ****************************************************************************** 00019 * @file BlueNRGGap.h 00020 * @author STMicroelectronics 00021 * @brief Header file for BlueNRG BLE_API Gap Class 00022 ****************************************************************************** 00023 * @copy 00024 * 00025 * THE PRESENT FIRMWARE WHICH IS FOR GUIDANCE ONLY AIMS AT PROVIDING CUSTOMERS 00026 * WITH CODING INFORMATION REGARDING THEIR PRODUCTS IN ORDER FOR THEM TO SAVE 00027 * TIME. AS A RESULT, STMICROELECTRONICS SHALL NOT BE HELD LIABLE FOR ANY 00028 * DIRECT, INDIRECT OR CONSEQUENTIAL DAMAGES WITH RESPECT TO ANY CLAIMS ARISING 00029 * FROM THE CONTENT OF SUCH FIRMWARE AND/OR THE USE MADE BY CUSTOMERS OF THE 00030 * CODING INFORMATION CONTAINED HEREIN IN CONNECTION WITH THEIR PRODUCTS. 00031 * 00032 * <h2><center>© COPYRIGHT 2013 STMicroelectronics</center></h2> 00033 */ 00034 00035 #ifndef __BLUENRG_GAP_H__ 00036 #define __BLUENRG_GAP_H__ 00037 00038 #include "mbed.h" 00039 #include "ble/blecommon.h" 00040 #include "btle.h" 00041 #include "ble/GapAdvertisingParams.h" 00042 #include "ble/GapAdvertisingData.h" 00043 #include "ble/Gap.h" 00044 00045 #define BLE_CONN_HANDLE_INVALID 0x0 00046 #define BDADDR_SIZE 6 00047 00048 #define BLUENRG_GAP_ADV_INTERVAL_MIN (0) 00049 #define BLUENRG_GAP_ADV_INTERVAL_MAX (0) 00050 #define BLE_GAP_ADV_NONCON_INTERVAL_MIN (0) 00051 00052 // Scanning and Connection Params used by Central for creating connection 00053 #define LIMITED_DISCOVERY_PROCEDURE 0x01 00054 #define GENERAL_DISCOVERY_PROCEDURE 0x02 00055 00056 #define SCAN_P (0x4000) 00057 #define SCAN_L (0x4000) 00058 #define SUPERV_TIMEOUT (600) 00059 #define CONN_P(x) ((int)((x)/1.25f)) 00060 #define CONN_L(x) ((int)((x)/0.625f)) 00061 #define CONN_P1 (CONN_P(50))//(CONN_P(1000)) 00062 #define CONN_P2 (CONN_P(50))//(CONN_P(1000)) 00063 #define CONN_L1 (CONN_L(5)) 00064 #define CONN_L2 (CONN_L(5)) 00065 00066 #define UUID_BUFFER_SIZE 17 //Either 8*2(16-bit UUIDs) or 4*4(32-bit UUIDs) or 1*16(128-bit UUIDs) +1 00067 #define ADV_DATA_MAX_SIZE 31 00068 00069 /**************************************************************************/ 00070 /*! 00071 \brief 00072 00073 */ 00074 /**************************************************************************/ 00075 class BlueNRGGap : public Gap 00076 { 00077 public: 00078 static BlueNRGGap &getInstance() { 00079 static BlueNRGGap m_instance; 00080 return m_instance; 00081 } 00082 00083 // <<<ANDREA>>> 00084 /* 00085 enum AdvType_t { 00086 ADV_IND = GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED,//Gap::ADV_IND, 00087 ADV_DIRECT_IND = GapAdvertisingParams::ADV_CONNECTABLE_DIRECTED,//Gap::ADV_DIRECT_IND, 00088 ADV_SCAN_IND = GapAdvertisingParams::ADV_SCANNABLE_UNDIRECTED,//Gap::ADV_SCAN_IND, 00089 ADV_NONCONN_IND = GapAdvertisingParams::ADV_NON_CONNECTABLE_UNDIRECTED//Gap::ADV_NONCONN_IND 00090 }; 00091 */ 00092 enum Reason_t { 00093 DEVICE_FOUND, 00094 DISCOVERY_COMPLETE 00095 }; 00096 00097 /* Functions that must be implemented from Gap */ 00098 virtual ble_error_t setAddress(addr_type_t type, const Address_t address); 00099 virtual ble_error_t getAddress(addr_type_t *typeP, Address_t address); 00100 virtual ble_error_t setAdvertisingData(const GapAdvertisingData &, const GapAdvertisingData &); 00101 virtual ble_error_t startAdvertising(const GapAdvertisingParams &); 00102 virtual ble_error_t stopAdvertising(void); 00103 virtual ble_error_t stopScan(); 00104 virtual uint16_t getMinAdvertisingInterval(void) const; 00105 virtual uint16_t getMinNonConnectableAdvertisingInterval(void) const; 00106 virtual uint16_t getMaxAdvertisingInterval(void) const; 00107 virtual ble_error_t disconnect(DisconnectionReason_t reason); 00108 virtual ble_error_t disconnect(Handle_t connectionHandle, DisconnectionReason_t reason); 00109 virtual ble_error_t getPreferredConnectionParams(ConnectionParams_t *params); 00110 virtual ble_error_t setPreferredConnectionParams(const ConnectionParams_t *params); 00111 virtual ble_error_t updateConnectionParams(Handle_t handle, const ConnectionParams_t *params); 00112 00113 virtual ble_error_t setDeviceName(const uint8_t *deviceName); 00114 virtual ble_error_t getDeviceName(uint8_t *deviceName, unsigned *lengthP); 00115 virtual ble_error_t setAppearance(GapAdvertisingData::Appearance appearance); 00116 virtual ble_error_t getAppearance(GapAdvertisingData::Appearance *appearanceP); 00117 00118 virtual ble_error_t setTxPower(int8_t txPower); 00119 virtual void getPermittedTxPowerValues(const int8_t **, size_t *); 00120 // <<<ANDREA>>> 00121 virtual ble_error_t connect(const Address_t peerAddr, 00122 Gap::AddressType_t peerAddrType, 00123 const ConnectionParams_t *connectionParams, 00124 const GapScanningParams *scanParams); 00125 00126 00127 void Discovery_CB(Reason_t reason, 00128 uint8_t adv_type, 00129 uint8_t *addr_type, 00130 uint8_t *addr, 00131 uint8_t *data_length, 00132 uint8_t *data, 00133 uint8_t *RSSI); 00134 ble_error_t createConnection(void); 00135 00136 void setConnectionHandle(uint16_t con_handle); 00137 uint16_t getConnectionHandle(void); 00138 00139 bool getIsSetAddress(); 00140 00141 Timeout getAdvTimeout(void) const { 00142 return advTimeout; 00143 } 00144 uint8_t getAdvToFlag(void) { 00145 return AdvToFlag; 00146 } 00147 void setAdvToFlag(void); 00148 00149 void Process(void); 00150 00151 GapScanningParams* getScanningParams(void); 00152 00153 virtual ble_error_t startRadioScan(const GapScanningParams &scanningParams); 00154 00155 private: 00156 uint16_t m_connectionHandle; 00157 AddressType_t addr_type; 00158 Address_t _peerAddr; 00159 uint8_t bdaddr[BDADDR_SIZE]; 00160 bool _scanning; 00161 bool _connecting; 00162 bool isSetAddress; 00163 bool btle_reinited; 00164 uint8_t *DeviceName; 00165 uint8_t deviceAppearance[2]; 00166 00167 uint8_t *local_name; 00168 uint8_t local_name_length; 00169 00170 uint8_t servUuidlength; 00171 uint8_t servUuidData[UUID_BUFFER_SIZE]; 00172 00173 uint8_t AdvLen; 00174 uint8_t AdvData[ADV_DATA_MAX_SIZE]; 00175 00176 Timeout advTimeout; 00177 bool AdvToFlag; 00178 00179 BlueNRGGap() { 00180 m_connectionHandle = BLE_CONN_HANDLE_INVALID; 00181 addr_type = BLEProtocol::AddressType::PUBLIC; 00182 isSetAddress = false; 00183 btle_reinited = false; 00184 DeviceName = NULL; 00185 } 00186 00187 BlueNRGGap(BlueNRGGap const &); 00188 void operator=(BlueNRGGap const &); 00189 }; 00190 00191 #endif // ifndef __BLUENRG_GAP_H__
Generated on Tue Jul 12 2022 19:31:15 by
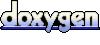