SDL Library
Embed:
(wiki syntax)
Show/hide line numbers
SDL_messagebox.h
00001 /* 00002 Simple DirectMedia Layer 00003 Copyright (C) 1997-2014 Sam Lantinga <slouken@libsdl.org> 00004 00005 This software is provided 'as-is', without any express or implied 00006 warranty. In no event will the authors be held liable for any damages 00007 arising from the use of this software. 00008 00009 Permission is granted to anyone to use this software for any purpose, 00010 including commercial applications, and to alter it and redistribute it 00011 freely, subject to the following restrictions: 00012 00013 1. The origin of this software must not be misrepresented; you must not 00014 claim that you wrote the original software. If you use this software 00015 in a product, an acknowledgment in the product documentation would be 00016 appreciated but is not required. 00017 2. Altered source versions must be plainly marked as such, and must not be 00018 misrepresented as being the original software. 00019 3. This notice may not be removed or altered from any source distribution. 00020 */ 00021 00022 #ifndef _SDL_messagebox_h 00023 #define _SDL_messagebox_h 00024 00025 #include "SDL_stdinc.h" 00026 #include "SDL_video.h" /* For SDL_Window */ 00027 00028 #include "begin_code.h" 00029 /* Set up for C function definitions, even when using C++ */ 00030 #ifdef __cplusplus 00031 extern "C" { 00032 #endif 00033 00034 /** 00035 * \brief SDL_MessageBox flags. If supported will display warning icon, etc. 00036 */ 00037 typedef enum 00038 { 00039 SDL_MESSAGEBOX_ERROR = 0x00000010, /**< error dialog */ 00040 SDL_MESSAGEBOX_WARNING = 0x00000020, /**< warning dialog */ 00041 SDL_MESSAGEBOX_INFORMATION = 0x00000040 /**< informational dialog */ 00042 } SDL_MessageBoxFlags; 00043 00044 /** 00045 * \brief Flags for SDL_MessageBoxButtonData. 00046 */ 00047 typedef enum 00048 { 00049 SDL_MESSAGEBOX_BUTTON_RETURNKEY_DEFAULT = 0x00000001, /**< Marks the default button when return is hit */ 00050 SDL_MESSAGEBOX_BUTTON_ESCAPEKEY_DEFAULT = 0x00000002 /**< Marks the default button when escape is hit */ 00051 } SDL_MessageBoxButtonFlags; 00052 00053 /** 00054 * \brief Individual button data. 00055 */ 00056 typedef struct 00057 { 00058 Uint32 flags; /**< ::SDL_MessageBoxButtonFlags */ 00059 int buttonid; /**< User defined button id (value returned via SDL_ShowMessageBox) */ 00060 const char * text; /**< The UTF-8 button text */ 00061 } SDL_MessageBoxButtonData; 00062 00063 /** 00064 * \brief RGB value used in a message box color scheme 00065 */ 00066 typedef struct 00067 { 00068 Uint8 r, g, b; 00069 } SDL_MessageBoxColor; 00070 00071 typedef enum 00072 { 00073 SDL_MESSAGEBOX_COLOR_BACKGROUND, 00074 SDL_MESSAGEBOX_COLOR_TEXT, 00075 SDL_MESSAGEBOX_COLOR_BUTTON_BORDER, 00076 SDL_MESSAGEBOX_COLOR_BUTTON_BACKGROUND, 00077 SDL_MESSAGEBOX_COLOR_BUTTON_SELECTED, 00078 SDL_MESSAGEBOX_COLOR_MAX 00079 } SDL_MessageBoxColorType; 00080 00081 /** 00082 * \brief A set of colors to use for message box dialogs 00083 */ 00084 typedef struct 00085 { 00086 SDL_MessageBoxColor colors[SDL_MESSAGEBOX_COLOR_MAX]; 00087 } SDL_MessageBoxColorScheme; 00088 00089 /** 00090 * \brief MessageBox structure containing title, text, window, etc. 00091 */ 00092 typedef struct 00093 { 00094 Uint32 flags; /**< ::SDL_MessageBoxFlags */ 00095 SDL_Window *window; /**< Parent window, can be NULL */ 00096 const char *title; /**< UTF-8 title */ 00097 const char *message; /**< UTF-8 message text */ 00098 00099 int numbuttons; 00100 const SDL_MessageBoxButtonData *buttons; 00101 00102 const SDL_MessageBoxColorScheme *colorScheme; /**< ::SDL_MessageBoxColorScheme, can be NULL to use system settings */ 00103 } SDL_MessageBoxData; 00104 00105 /** 00106 * \brief Create a modal message box. 00107 * 00108 * \param messageboxdata The SDL_MessageBoxData structure with title, text, etc. 00109 * \param buttonid The pointer to which user id of hit button should be copied. 00110 * 00111 * \return -1 on error, otherwise 0 and buttonid contains user id of button 00112 * hit or -1 if dialog was closed. 00113 * 00114 * \note This function should be called on the thread that created the parent 00115 * window, or on the main thread if the messagebox has no parent. It will 00116 * block execution of that thread until the user clicks a button or 00117 * closes the messagebox. 00118 */ 00119 extern DECLSPEC int SDLCALL SDL_ShowMessageBox(const SDL_MessageBoxData *messageboxdata, int *buttonid); 00120 00121 /** 00122 * \brief Create a simple modal message box 00123 * 00124 * \param flags ::SDL_MessageBoxFlags 00125 * \param title UTF-8 title text 00126 * \param message UTF-8 message text 00127 * \param window The parent window, or NULL for no parent 00128 * 00129 * \return 0 on success, -1 on error 00130 * 00131 * \sa SDL_ShowMessageBox 00132 */ 00133 extern DECLSPEC int SDLCALL SDL_ShowSimpleMessageBox(Uint32 flags, const char *title, const char *message, SDL_Window *window); 00134 00135 00136 /* Ends C function definitions when using C++ */ 00137 #ifdef __cplusplus 00138 } 00139 #endif 00140 #include "close_code.h" 00141 00142 #endif /* _SDL_messagebox_h */ 00143 00144 /* vi: set ts=4 sw=4 expandtab: */
Generated on Tue Jul 12 2022 15:10:19 by
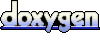