SDL Library
Embed:
(wiki syntax)
Show/hide line numbers
SDL_keyboard.h
Go to the documentation of this file.
00001 /* 00002 Simple DirectMedia Layer 00003 Copyright (C) 1997-2014 Sam Lantinga <slouken@libsdl.org> 00004 00005 This software is provided 'as-is', without any express or implied 00006 warranty. In no event will the authors be held liable for any damages 00007 arising from the use of this software. 00008 00009 Permission is granted to anyone to use this software for any purpose, 00010 including commercial applications, and to alter it and redistribute it 00011 freely, subject to the following restrictions: 00012 00013 1. The origin of this software must not be misrepresented; you must not 00014 claim that you wrote the original software. If you use this software 00015 in a product, an acknowledgment in the product documentation would be 00016 appreciated but is not required. 00017 2. Altered source versions must be plainly marked as such, and must not be 00018 misrepresented as being the original software. 00019 3. This notice may not be removed or altered from any source distribution. 00020 */ 00021 00022 /** 00023 * \file SDL_keyboard.h 00024 * 00025 * Include file for SDL keyboard event handling 00026 */ 00027 00028 #ifndef _SDL_keyboard_h 00029 #define _SDL_keyboard_h 00030 00031 #include "SDL_stdinc.h" 00032 #include "SDL_error.h" 00033 #include "SDL_keycode.h" 00034 #include "SDL_video.h" 00035 00036 #include "begin_code.h" 00037 /* Set up for C function definitions, even when using C++ */ 00038 #ifdef __cplusplus 00039 extern "C" { 00040 #endif 00041 00042 /** 00043 * \brief The SDL keysym structure, used in key events. 00044 * 00045 * \note If you are looking for translated character input, see the ::SDL_TEXTINPUT event. 00046 */ 00047 typedef struct SDL_Keysym 00048 { 00049 SDL_Scancode scancode; /**< SDL physical key code - see ::SDL_Scancode for details */ 00050 SDL_Keycode sym; /**< SDL virtual key code - see ::SDL_Keycode for details */ 00051 Uint16 mod; /**< current key modifiers */ 00052 Uint32 unused; 00053 } SDL_Keysym; 00054 00055 /* Function prototypes */ 00056 00057 /** 00058 * \brief Get the window which currently has keyboard focus. 00059 */ 00060 extern DECLSPEC SDL_Window * SDLCALL SDL_GetKeyboardFocus(void); 00061 00062 /** 00063 * \brief Get a snapshot of the current state of the keyboard. 00064 * 00065 * \param numkeys if non-NULL, receives the length of the returned array. 00066 * 00067 * \return An array of key states. Indexes into this array are obtained by using ::SDL_Scancode values. 00068 * 00069 * \b Example: 00070 * \code 00071 * const Uint8 *state = SDL_GetKeyboardState(NULL); 00072 * if ( state[SDL_SCANCODE_RETURN] ) { 00073 * printf("<RETURN> is pressed.\n"); 00074 * } 00075 * \endcode 00076 */ 00077 extern DECLSPEC const Uint8 *SDLCALL SDL_GetKeyboardState(int *numkeys); 00078 00079 /** 00080 * \brief Get the current key modifier state for the keyboard. 00081 */ 00082 extern DECLSPEC SDL_Keymod SDLCALL SDL_GetModState(void); 00083 00084 /** 00085 * \brief Set the current key modifier state for the keyboard. 00086 * 00087 * \note This does not change the keyboard state, only the key modifier flags. 00088 */ 00089 extern DECLSPEC void SDLCALL SDL_SetModState(SDL_Keymod modstate); 00090 00091 /** 00092 * \brief Get the key code corresponding to the given scancode according 00093 * to the current keyboard layout. 00094 * 00095 * See ::SDL_Keycode for details. 00096 * 00097 * \sa SDL_GetKeyName() 00098 */ 00099 extern DECLSPEC SDL_Keycode SDLCALL SDL_GetKeyFromScancode(SDL_Scancode scancode); 00100 00101 /** 00102 * \brief Get the scancode corresponding to the given key code according to the 00103 * current keyboard layout. 00104 * 00105 * See ::SDL_Scancode for details. 00106 * 00107 * \sa SDL_GetScancodeName() 00108 */ 00109 extern DECLSPEC SDL_Scancode SDLCALL SDL_GetScancodeFromKey(SDL_Keycode key); 00110 00111 /** 00112 * \brief Get a human-readable name for a scancode. 00113 * 00114 * \return A pointer to the name for the scancode. 00115 * If the scancode doesn't have a name, this function returns 00116 * an empty string (""). 00117 * 00118 * \sa SDL_Scancode 00119 */ 00120 extern DECLSPEC const char *SDLCALL SDL_GetScancodeName(SDL_Scancode scancode); 00121 00122 /** 00123 * \brief Get a scancode from a human-readable name 00124 * 00125 * \return scancode, or SDL_SCANCODE_UNKNOWN if the name wasn't recognized 00126 * 00127 * \sa SDL_Scancode 00128 */ 00129 extern DECLSPEC SDL_Scancode SDLCALL SDL_GetScancodeFromName(const char *name); 00130 00131 /** 00132 * \brief Get a human-readable name for a key. 00133 * 00134 * \return A pointer to a UTF-8 string that stays valid at least until the next 00135 * call to this function. If you need it around any longer, you must 00136 * copy it. If the key doesn't have a name, this function returns an 00137 * empty string (""). 00138 * 00139 * \sa SDL_Key 00140 */ 00141 extern DECLSPEC const char *SDLCALL SDL_GetKeyName(SDL_Keycode key); 00142 00143 /** 00144 * \brief Get a key code from a human-readable name 00145 * 00146 * \return key code, or SDLK_UNKNOWN if the name wasn't recognized 00147 * 00148 * \sa SDL_Keycode 00149 */ 00150 extern DECLSPEC SDL_Keycode SDLCALL SDL_GetKeyFromName(const char *name); 00151 00152 /** 00153 * \brief Start accepting Unicode text input events. 00154 * This function will show the on-screen keyboard if supported. 00155 * 00156 * \sa SDL_StopTextInput() 00157 * \sa SDL_SetTextInputRect() 00158 * \sa SDL_HasScreenKeyboardSupport() 00159 */ 00160 extern DECLSPEC void SDLCALL SDL_StartTextInput(void); 00161 00162 /** 00163 * \brief Return whether or not Unicode text input events are enabled. 00164 * 00165 * \sa SDL_StartTextInput() 00166 * \sa SDL_StopTextInput() 00167 */ 00168 extern DECLSPEC SDL_bool SDLCALL SDL_IsTextInputActive(void); 00169 00170 /** 00171 * \brief Stop receiving any text input events. 00172 * This function will hide the on-screen keyboard if supported. 00173 * 00174 * \sa SDL_StartTextInput() 00175 * \sa SDL_HasScreenKeyboardSupport() 00176 */ 00177 extern DECLSPEC void SDLCALL SDL_StopTextInput(void); 00178 00179 /** 00180 * \brief Set the rectangle used to type Unicode text inputs. 00181 * This is used as a hint for IME and on-screen keyboard placement. 00182 * 00183 * \sa SDL_StartTextInput() 00184 */ 00185 extern DECLSPEC void SDLCALL SDL_SetTextInputRect(SDL_Rect *rect); 00186 00187 /** 00188 * \brief Returns whether the platform has some screen keyboard support. 00189 * 00190 * \return SDL_TRUE if some keyboard support is available else SDL_FALSE. 00191 * 00192 * \note Not all screen keyboard functions are supported on all platforms. 00193 * 00194 * \sa SDL_IsScreenKeyboardShown() 00195 */ 00196 extern DECLSPEC SDL_bool SDLCALL SDL_HasScreenKeyboardSupport(void); 00197 00198 /** 00199 * \brief Returns whether the screen keyboard is shown for given window. 00200 * 00201 * \param window The window for which screen keyboard should be queried. 00202 * 00203 * \return SDL_TRUE if screen keyboard is shown else SDL_FALSE. 00204 * 00205 * \sa SDL_HasScreenKeyboardSupport() 00206 */ 00207 extern DECLSPEC SDL_bool SDLCALL SDL_IsScreenKeyboardShown(SDL_Window *window); 00208 00209 /* Ends C function definitions when using C++ */ 00210 #ifdef __cplusplus 00211 } 00212 #endif 00213 #include "close_code.h" 00214 00215 #endif /* _SDL_keyboard_h */ 00216 00217 /* vi: set ts=4 sw=4 expandtab: */
Generated on Tue Jul 12 2022 15:10:18 by
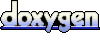