SDL Library
Embed:
(wiki syntax)
Show/hide line numbers
SDL_endian.h
Go to the documentation of this file.
00001 /* 00002 Simple DirectMedia Layer 00003 Copyright (C) 1997-2014 Sam Lantinga <slouken@libsdl.org> 00004 00005 This software is provided 'as-is', without any express or implied 00006 warranty. In no event will the authors be held liable for any damages 00007 arising from the use of this software. 00008 00009 Permission is granted to anyone to use this software for any purpose, 00010 including commercial applications, and to alter it and redistribute it 00011 freely, subject to the following restrictions: 00012 00013 1. The origin of this software must not be misrepresented; you must not 00014 claim that you wrote the original software. If you use this software 00015 in a product, an acknowledgment in the product documentation would be 00016 appreciated but is not required. 00017 2. Altered source versions must be plainly marked as such, and must not be 00018 misrepresented as being the original software. 00019 3. This notice may not be removed or altered from any source distribution. 00020 */ 00021 00022 /** 00023 * \file SDL_endian.h 00024 * 00025 * Functions for reading and writing endian-specific values 00026 */ 00027 00028 #ifndef _SDL_endian_h 00029 #define _SDL_endian_h 00030 00031 #include "SDL_stdinc.h" 00032 00033 /** 00034 * \name The two types of endianness 00035 */ 00036 /* @{ */ 00037 #define SDL_LIL_ENDIAN 1234 00038 #define SDL_BIG_ENDIAN 4321 00039 /* @} */ 00040 00041 #ifndef SDL_BYTEORDER /* Not defined in SDL_config.h? */ 00042 #ifdef __linux__ 00043 #include <endian.h> 00044 #define SDL_BYTEORDER __BYTE_ORDER 00045 #else /* __linux __ */ 00046 #if defined(__hppa__) || \ 00047 defined(__m68k__) || defined(mc68000) || defined(_M_M68K) || \ 00048 (defined(__MIPS__) && defined(__MISPEB__)) || \ 00049 defined(__ppc__) || defined(__POWERPC__) || defined(_M_PPC) || \ 00050 defined(__sparc__) 00051 #define SDL_BYTEORDER SDL_BIG_ENDIAN 00052 #else 00053 #define SDL_BYTEORDER SDL_LIL_ENDIAN 00054 #endif 00055 #endif /* __linux __ */ 00056 #endif /* !SDL_BYTEORDER */ 00057 00058 00059 #include "begin_code.h" 00060 /* Set up for C function definitions, even when using C++ */ 00061 #ifdef __cplusplus 00062 extern "C" { 00063 #endif 00064 00065 /** 00066 * \file SDL_endian.h 00067 */ 00068 #if defined(__GNUC__) && defined(__i386__) && \ 00069 !(__GNUC__ == 2 && __GNUC_MINOR__ == 95 /* broken gcc version */) 00070 SDL_FORCE_INLINE Uint16 00071 SDL_Swap16(Uint16 x) 00072 { 00073 __asm__("xchgb %b0,%h0": "=q"(x):"0"(x)); 00074 return x; 00075 } 00076 #elif defined(__GNUC__) && defined(__x86_64__) 00077 SDL_FORCE_INLINE Uint16 00078 SDL_Swap16(Uint16 x) 00079 { 00080 __asm__("xchgb %b0,%h0": "=Q"(x):"0"(x)); 00081 return x; 00082 } 00083 #elif defined(__GNUC__) && (defined(__powerpc__) || defined(__ppc__)) 00084 SDL_FORCE_INLINE Uint16 00085 SDL_Swap16(Uint16 x) 00086 { 00087 int result; 00088 00089 __asm__("rlwimi %0,%2,8,16,23": "=&r"(result):"0"(x >> 8), "r"(x)); 00090 return (Uint16)result; 00091 } 00092 #elif defined(__GNUC__) && (defined(__M68000__) || defined(__M68020__)) && !defined(__mcoldfire__) 00093 SDL_FORCE_INLINE Uint16 00094 SDL_Swap16(Uint16 x) 00095 { 00096 __asm__("rorw #8,%0": "=d"(x): "0"(x):"cc"); 00097 return x; 00098 } 00099 #else 00100 SDL_FORCE_INLINE Uint16 00101 SDL_Swap16(Uint16 x) 00102 { 00103 return SDL_static_cast(Uint16, ((x << 8) | (x >> 8))); 00104 } 00105 #endif 00106 00107 #if defined(__GNUC__) && defined(__i386__) 00108 SDL_FORCE_INLINE Uint32 00109 SDL_Swap32(Uint32 x) 00110 { 00111 __asm__("bswap %0": "=r"(x):"0"(x)); 00112 return x; 00113 } 00114 #elif defined(__GNUC__) && defined(__x86_64__) 00115 SDL_FORCE_INLINE Uint32 00116 SDL_Swap32(Uint32 x) 00117 { 00118 __asm__("bswapl %0": "=r"(x):"0"(x)); 00119 return x; 00120 } 00121 #elif defined(__GNUC__) && (defined(__powerpc__) || defined(__ppc__)) 00122 SDL_FORCE_INLINE Uint32 00123 SDL_Swap32(Uint32 x) 00124 { 00125 Uint32 result; 00126 00127 __asm__("rlwimi %0,%2,24,16,23": "=&r"(result):"0"(x >> 24), "r"(x)); 00128 __asm__("rlwimi %0,%2,8,8,15": "=&r"(result):"0"(result), "r"(x)); 00129 __asm__("rlwimi %0,%2,24,0,7": "=&r"(result):"0"(result), "r"(x)); 00130 return result; 00131 } 00132 #elif defined(__GNUC__) && (defined(__M68000__) || defined(__M68020__)) && !defined(__mcoldfire__) 00133 SDL_FORCE_INLINE Uint32 00134 SDL_Swap32(Uint32 x) 00135 { 00136 __asm__("rorw #8,%0\n\tswap %0\n\trorw #8,%0": "=d"(x): "0"(x):"cc"); 00137 return x; 00138 } 00139 #else 00140 SDL_FORCE_INLINE Uint32 00141 SDL_Swap32(Uint32 x) 00142 { 00143 return SDL_static_cast(Uint32, ((x << 24) | ((x << 8) & 0x00FF0000) | 00144 ((x >> 8) & 0x0000FF00) | (x >> 24))); 00145 } 00146 #endif 00147 00148 #if defined(__GNUC__) && defined(__i386__) 00149 SDL_FORCE_INLINE Uint64 00150 SDL_Swap64(Uint64 x) 00151 { 00152 union 00153 { 00154 struct 00155 { 00156 Uint32 a, b; 00157 } s; 00158 Uint64 u; 00159 } v; 00160 v.u = x; 00161 __asm__("bswapl %0 ; bswapl %1 ; xchgl %0,%1": "=r"(v.s.a), "=r"(v.s.b):"0"(v.s.a), 00162 "1"(v.s. 00163 b)); 00164 return v.u; 00165 } 00166 #elif defined(__GNUC__) && defined(__x86_64__) 00167 SDL_FORCE_INLINE Uint64 00168 SDL_Swap64(Uint64 x) 00169 { 00170 __asm__("bswapq %0": "=r"(x):"0"(x)); 00171 return x; 00172 } 00173 #else 00174 SDL_FORCE_INLINE Uint64 00175 SDL_Swap64(Uint64 x) 00176 { 00177 Uint32 hi, lo; 00178 00179 /* Separate into high and low 32-bit values and swap them */ 00180 lo = SDL_static_cast(Uint32, x & 0xFFFFFFFF); 00181 x >>= 32; 00182 hi = SDL_static_cast(Uint32, x & 0xFFFFFFFF); 00183 x = SDL_Swap32(lo); 00184 x <<= 32; 00185 x |= SDL_Swap32(hi); 00186 return (x); 00187 } 00188 #endif 00189 00190 00191 SDL_FORCE_INLINE float 00192 SDL_SwapFloat(float x) 00193 { 00194 union 00195 { 00196 float f; 00197 Uint32 ui32; 00198 } swapper; 00199 swapper.f = x; 00200 swapper.ui32 = SDL_Swap32(swapper.ui32); 00201 return swapper.f; 00202 } 00203 00204 00205 /** 00206 * \name Swap to native 00207 * Byteswap item from the specified endianness to the native endianness. 00208 */ 00209 /* @{ */ 00210 #if SDL_BYTEORDER == SDL_LIL_ENDIAN 00211 #define SDL_SwapLE16(X) (X) 00212 #define SDL_SwapLE32(X) (X) 00213 #define SDL_SwapLE64(X) (X) 00214 #define SDL_SwapFloatLE(X) (X) 00215 #define SDL_SwapBE16(X) SDL_Swap16(X) 00216 #define SDL_SwapBE32(X) SDL_Swap32(X) 00217 #define SDL_SwapBE64(X) SDL_Swap64(X) 00218 #define SDL_SwapFloatBE(X) SDL_SwapFloat(X) 00219 #else 00220 #define SDL_SwapLE16(X) SDL_Swap16(X) 00221 #define SDL_SwapLE32(X) SDL_Swap32(X) 00222 #define SDL_SwapLE64(X) SDL_Swap64(X) 00223 #define SDL_SwapFloatLE(X) SDL_SwapFloat(X) 00224 #define SDL_SwapBE16(X) (X) 00225 #define SDL_SwapBE32(X) (X) 00226 #define SDL_SwapBE64(X) (X) 00227 #define SDL_SwapFloatBE(X) (X) 00228 #endif 00229 /* @} *//* Swap to native */ 00230 00231 /* Ends C function definitions when using C++ */ 00232 #ifdef __cplusplus 00233 } 00234 #endif 00235 #include "close_code.h" 00236 00237 #endif /* _SDL_endian_h */ 00238 00239 /* vi: set ts=4 sw=4 expandtab: */
Generated on Tue Jul 12 2022 15:10:18 by
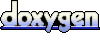