HW-6.1
Fork of MMA8451Q by
Embed:
(wiki syntax)
Show/hide line numbers
MMA8451Q.cpp
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 00020 00021 #include "MMA8451Q.h" 00022 00023 #define REG_WHO_AM_I 0x0D 00024 #define REG_CTRL_REG_1 0x2A 00025 #define REG_OUT_X_MSB 0x01 00026 #define REG_OUT_Y_MSB 0x03 00027 #define REG_OUT_Z_MSB 0x05 00028 #define XYZ_DATA_CFG 0x0E 00029 00030 #define UINT14_MAX 16383 00031 00032 #define MAX_2G 0x00 00033 #define MAX_4G 0X01 00034 #define MAX_8G 0X10 00035 00036 #define GSCALING 1024.0 00037 00038 MMA8451Q::MMA8451Q(PinName sda, PinName scl, int addr) : m_i2c(sda, scl), m_addr(addr) { 00039 // activate the peripheral 00040 uint8_t data[2] = {REG_CTRL_REG_1, 0x01}; 00041 writeRegs(data, 2); 00042 } 00043 00044 void MMA8451Q::setGLImit() 00045 { 00046 uint8_t data[2] = {REG_CTRL_REG_1, 0x00}; 00047 writeRegs(data, 2); //put in standby 00048 data[0] = XYZ_DATA_CFG; 00049 data[1] = 0x02; 00050 writeRegs(data, 2); 00051 data[0] = REG_CTRL_REG_1; 00052 data[1] = 0x01; 00053 writeRegs(data, 2); //make active 00054 } 00055 00056 MMA8451Q::~MMA8451Q() { } 00057 00058 //uint8_t MMA8451Q::getGLimit(uint8_t regData) 00059 //{ 00060 // acc.getGLimit(); 00061 // acc.readRegs(XYZ_DATA_CFG, ®Data, 1); 00062 // sprintf(lcdData, "%d", regData); 00063 // LCDMess(lcdData, BLINKTIME); 00064 // acc.readRegs(REG_WHO_AM_I, ®Data, 1); 00065 // springf(lcdData, "%d", regData); 00066 // LCDMess(lcdData, BLINKTIME); 00067 // 00068 // return regDate 00069 //} 00070 00071 uint8_t MMA8451Q::getWhoAmI() { 00072 uint8_t who_am_i = 0; 00073 readRegs(REG_WHO_AM_I, &who_am_i, 1); 00074 return who_am_i; 00075 } 00076 00077 float MMA8451Q::getAccX() { 00078 return (float(getAccAxis(REG_OUT_X_MSB))/4096.0); 00079 } 00080 00081 float MMA8451Q::getAccY() { 00082 return (float(getAccAxis(REG_OUT_Y_MSB))/4096.0); 00083 } 00084 00085 float MMA8451Q::getAccZ() { 00086 return (float(getAccAxis(REG_OUT_Z_MSB))/4096.0); 00087 } 00088 00089 void MMA8451Q::getAccAllAxis(float * res) { 00090 res[0] = getAccX(); 00091 res[1] = getAccY(); 00092 res[2] = getAccZ(); 00093 } 00094 00095 int16_t MMA8451Q::getAccAxis(uint8_t addr) { 00096 int16_t acc; 00097 uint8_t res[2]; 00098 readRegs(addr, res, 2); 00099 00100 acc = (res[0] << 6) | (res[1] >> 2); 00101 if (acc > UINT14_MAX/2) 00102 acc -= UINT14_MAX; 00103 00104 return acc; 00105 } 00106 00107 void MMA8451Q::readRegs(int addr, uint8_t * data, int len) { 00108 char t[1] = {addr}; 00109 m_i2c.write(m_addr, t, 1, true); 00110 m_i2c.read(m_addr, (char *)data, len); 00111 } 00112 00113 void MMA8451Q::writeRegs(uint8_t * data, int len) { 00114 m_i2c.write(m_addr, (char *)data, len); 00115 }
Generated on Wed Jul 13 2022 23:06:09 by
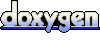