
a simple c coroutine for mbed paltform
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "coroutine.h" 00003 00004 volatile uint16_t cr_time1; 00005 volatile uint16_t cr_time2; 00006 00007 void user_thread1(void); 00008 void user_thread2(void); 00009 00010 Ticker toggle_led_ticker; 00011 DigitalOut led1(LED1); 00012 DigitalOut led2(LED2); 00013 00014 void isr_ticker() { 00015 cr_time1++; 00016 cr_time2++; 00017 } 00018 00019 00020 00021 int main(void) { 00022 // Init the ticker with the address of the function (isr_ticker) to be attached and the interval (1 ms) 00023 toggle_led_ticker.attach(&isr_ticker, 0.001); 00024 while (true) { 00025 // Do other things... 00026 user_thread1(); 00027 user_thread2(); 00028 } 00029 } 00030 00031 void user_thread1(void) 00032 { 00033 cr_start(); 00034 00035 // inital once each loop 00036 cr_time1 = 0; 00037 // waiting for condition is satisfied, or will be yield 00038 cr_yield(cr_time1 != 1000); 00039 led1 = !led1; 00040 00041 cr_end(); 00042 } 00043 00044 void user_thread2(void) 00045 { 00046 cr_start(); 00047 00048 // inital once each loop 00049 cr_time2 = 0; 00050 // waiting for condition is satisfied, or will be yield 00051 cr_yield(cr_time2 != 800); 00052 led2 = !led2; 00053 00054 cr_end(); 00055 }
Generated on Tue Jul 26 2022 09:48:13 by
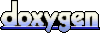