
Knight Rider PIO sample for teckBASIC, konashi.js
Dependencies: BLE_API_Native_IRC mbed
Fork of BLE_konashi_PIO_test by
binary.h
00001 /**************************************************************************/ 00002 /*! 00003 @file binary.h 00004 @author hathach (tinyusb.org) 00005 00006 @section LICENSE 00007 00008 Software License Agreement (BSD License) 00009 00010 Copyright (c) 2013, K. Townsend (microBuilder.eu) 00011 All rights reserved. 00012 00013 Redistribution and use in source and binary forms, with or without 00014 modification, are permitted provided that the following conditions are met: 00015 1. Redistributions of source code must retain the above copyright 00016 notice, this list of conditions and the following disclaimer. 00017 2. Redistributions in binary form must reproduce the above copyright 00018 notice, this list of conditions and the following disclaimer in the 00019 documentation and/or other materials provided with the distribution. 00020 3. Neither the name of the copyright holders nor the 00021 names of its contributors may be used to endorse or promote products 00022 derived from this software without specific prior written permission. 00023 00024 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS ''AS IS'' AND ANY 00025 EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00026 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER BE LIABLE FOR ANY 00028 DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00029 INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00030 LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION HOWEVER CAUSED AND 00031 ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00032 INCLUDING NEGLIGENCE OR OTHERWISE ARISING IN ANY WAY OUT OF THE USE OF THIS 00033 SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 /**************************************************************************/ 00036 00037 /** \ingroup TBD 00038 * \defgroup TBD 00039 * \brief TBD 00040 * 00041 * @{ 00042 */ 00043 00044 #ifndef _BINARY_H_ 00045 #define _BINARY_H_ 00046 00047 #ifdef __cplusplus 00048 extern "C" { 00049 #endif 00050 00051 /// n-th Bit 00052 #define BIT(n) (1 << (n)) 00053 00054 /// set n-th bit of x to 1 00055 #define BIT_SET(x, n) ( (x) | BIT(n) ) 00056 00057 /// clear n-th bit of x 00058 #define BIT_CLR(x, n) ( (x) & (~BIT(n)) ) 00059 00060 /// test n-th bit of x 00061 #define BIT_TEST(x, n) ( (x) & BIT(n) ) 00062 00063 #if defined(__GNUC__) && !defined(__CC_ARM) // keil does not support binary format 00064 00065 #define BIN8(x) ((uint8_t) (0b##x)) 00066 #define BIN16(b1, b2) ((uint16_t) (0b##b1##b2)) 00067 #define BIN32(b1, b2, b3, b4) ((uint32_t) (0b##b1##b2##b3##b4)) 00068 00069 #else 00070 00071 // internal macro of B8, B16, B32 00072 #define _B8__(x) (((x&0x0000000FUL)?1:0) \ 00073 +((x&0x000000F0UL)?2:0) \ 00074 +((x&0x00000F00UL)?4:0) \ 00075 +((x&0x0000F000UL)?8:0) \ 00076 +((x&0x000F0000UL)?16:0) \ 00077 +((x&0x00F00000UL)?32:0) \ 00078 +((x&0x0F000000UL)?64:0) \ 00079 +((x&0xF0000000UL)?128:0)) 00080 00081 #define BIN8(d) ((uint8_t) _B8__(0x##d##UL)) 00082 #define BIN16(dmsb,dlsb) (((uint16_t)BIN8(dmsb)<<8) + BIN8(dlsb)) 00083 #define BIN32(dmsb,db2,db3,dlsb) \ 00084 (((uint32_t)BIN8(dmsb)<<24) \ 00085 + ((uint32_t)BIN8(db2)<<16) \ 00086 + ((uint32_t)BIN8(db3)<<8) \ 00087 + BIN8(dlsb)) 00088 #endif 00089 00090 #ifdef __cplusplus 00091 } 00092 #endif 00093 00094 #endif /* _BINARY_H_ */ 00095 00096 /** @} */
Generated on Tue Jul 12 2022 16:55:06 by
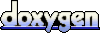