observe fixes
Dependencies: nanoservice_client_1_12_X Nanostack_lib
Fork of mbedEndpointNetwork_6LowPAN by
nsdl_dbg.cpp
00001 #include "debug.h" 00002 00003 // we have to redefine DBG as its used differently here... 00004 #ifdef DBG 00005 #undef DBG 00006 #define DBG printf 00007 #endif 00008 00009 /* 00010 * \brief Help round function. 00011 * 00012 * \param value Divident 00013 * \param divider Divisor 00014 * 00015 * \return Quotient 00016 */ 00017 uint32_t debug_round(uint32_t value, uint32_t divider) 00018 { 00019 uint32_t tmp = value; 00020 value *= 10; 00021 value /= divider; 00022 tmp = value; 00023 while(tmp > 10) 00024 tmp -= 10; 00025 if(((tmp & 0x0000000f) > 4) && ((tmp & 0x0000000f) < 10)) 00026 value += 10; 00027 if(tmp != 10) 00028 value -= tmp; 00029 value /= 10; 00030 return value; 00031 } 00032 00033 /** 00034 * Print a number to the debug port. 00035 * 00036 * \param width string maximum length 00037 * \param base base number (16 for hex, 10 for decimal etc.) 00038 * \param n number value 00039 * 00040 * \return pointer to the formatted string 00041 */ 00042 void debug_integer(uint8_t width, uint8_t base, int16_t n) 00043 { 00044 uint8_t bfr[8]; 00045 uint8_t *ptr = bfr; 00046 uint8_t ctr = 0; 00047 00048 if (width > 7) width = 7; 00049 00050 ptr += width; 00051 *ptr-- = 0; 00052 00053 if (base == 16) 00054 { 00055 do 00056 { 00057 *ptr = n & 0x0F; 00058 if (*ptr < 10) *ptr += '0'; 00059 else *ptr += ('A'-10); 00060 ptr--; 00061 n >>= 4; 00062 ctr++; 00063 }while((ctr & 1) || (ctr < width)); 00064 } 00065 else 00066 { 00067 uint8_t negative = 0; 00068 if (n < 0) 00069 { negative = 1; 00070 n = -n; 00071 } 00072 ctr++; 00073 do 00074 { 00075 *ptr-- = (n % 10) + '0'; 00076 n /= 10; 00077 ctr++; 00078 }while ((ctr < width) && n); 00079 if (negative) 00080 { 00081 *ptr-- = '-'; 00082 } 00083 else 00084 { 00085 *ptr-- = ' '; 00086 } 00087 } 00088 ptr++; 00089 //debug_send(ptr); 00090 DBG((const char *)ptr); 00091 } 00092 00093 /** 00094 * Print data array in HEX format. Bytes are separated with colon. 00095 * 00096 * \param ptr Pointer to 8-bit data array. 00097 * \param len Amount of printed bytes 00098 * 00099 */ 00100 void printf_array(uint8_t *ptr , uint16_t len) 00101 { 00102 uint16_t i; 00103 for(i=0; i<len; i++) 00104 { 00105 if(i) 00106 { 00107 if(i%16== 0) 00108 { 00109 DBG("\r\n"); 00110 if(len > 64) 00111 { 00112 uint8_t x =254; 00113 while(x--); 00114 } 00115 } 00116 else 00117 { 00118 DBG(":"); 00119 } 00120 } 00121 debug_hex(*ptr++); 00122 } 00123 DBG("\r\n"); 00124 } 00125 00126 /** 00127 * Print a IPv6 address. 00128 * 00129 * \param addr_ptr pointer to ipv6 address 00130 * 00131 */ 00132 void printf_ipv6_address(uint8_t *addr_ptr) 00133 { 00134 if(addr_ptr) 00135 { 00136 uint8_t i, d_colon = 0; 00137 uint16_t current_value = 0, last_value = 0; 00138 00139 for(i=0; i< 16;i += 2) 00140 { 00141 current_value = (*addr_ptr++ << 8); 00142 current_value += *addr_ptr++; 00143 00144 if(i == 0) 00145 { 00146 last_value = current_value; 00147 debug_hex(current_value >> 8); 00148 debug_hex(current_value ); 00149 DBG(":"); 00150 } 00151 else 00152 { 00153 if(current_value == 0) 00154 { 00155 if(i== 14) 00156 { 00157 DBG(":"); 00158 //debug_put('0'); 00159 DBG("0"); 00160 } 00161 else 00162 { 00163 if(last_value == 0) 00164 { 00165 if(d_colon == 0) 00166 { 00167 d_colon=1; 00168 } 00169 } 00170 else 00171 { 00172 if(d_colon == 2) 00173 { 00174 //debug_put('0'); 00175 DBG("0"); 00176 DBG(":"); 00177 } 00178 } 00179 } 00180 } 00181 else 00182 { 00183 if(last_value == 0) 00184 { 00185 if(d_colon == 1) 00186 { 00187 DBG(":"); 00188 d_colon = 2; 00189 } 00190 else 00191 { 00192 //debug_put('0'); 00193 DBG("0"); 00194 DBG(":"); 00195 } 00196 } 00197 if(current_value > 0x00ff) 00198 { 00199 debug_hex(current_value >> 8); 00200 } 00201 debug_hex(current_value ); 00202 if(i< 14) 00203 { 00204 DBG(":"); 00205 } 00206 } 00207 last_value = current_value; 00208 } 00209 } 00210 } 00211 else 00212 { 00213 DBG("Address Print: pointer NULL"); 00214 } 00215 DBG("\r\n"); 00216 }
Generated on Fri Jul 15 2022 08:02:55 by
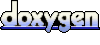