observe fixes
Dependencies: nanoservice_client_1_12_X Nanostack_lib
Fork of mbedEndpointNetwork_6LowPAN by
ns_6lowpan_support.cpp
00001 #include "configuration.h" 00002 #include "mbedConnectorInterface.h" 00003 #include "ns_6lowpan_support.h" 00004 #include "nsdl_support.h" 00005 #include "nsdl_utils.h" 00006 #include "debug.h" 00007 00008 // we have to redefine DBG as its used differently here... 00009 #ifdef DBG 00010 #undef DBG 00011 #define DBG printf 00012 #endif 00013 00014 // externally defined configurable... 00015 extern uint8_t app_MAC_address[8]; 00016 extern uint32_t channel_list; 00017 00018 // Globals 00019 static int8_t rf_phy_device_register_id = -1; 00020 static int8_t net_6lowpan_id = -1; 00021 int8_t coap_udp_socket = -1; 00022 static int access_point_status = 0; 00023 uint8_t app_defined_stack_heap[APP_DEV_HEAP_SIZE]; 00024 00025 /** Used for Receive Data source Address store*/ 00026 static ns_address_t app_src; 00027 static sn_nsdl_addr_s sn_addr_s; 00028 00029 extern void app_heap_error_handler(heap_fail_t event); 00030 00031 link_layer_setups_s app_link_info; 00032 link_layer_address_s app_link_address_info; 00033 network_layer_address_s app_nd_address_info; 00034 00035 // initialize the 6LowPAN network 00036 void init_network(bool canActAsRouterNode) { 00037 /* 00038 * Currently based on non-Thread 6LowPAN stack 00039 */ 00040 DBG("\r\ninit_network: Initializing network stack heap and MAC address...\r\n"); 00041 ns_dyn_mem_init(app_defined_stack_heap, APP_DEV_HEAP_SIZE, app_heap_error_handler,0); 00042 rf_set_mac_address(app_MAC_address); 00043 00044 // init RF interface 00045 DBG("init_network: Initializing the RF Interface...\r\n"); 00046 rf_phy_device_register_id = rf_device_register(); 00047 randLIB_seed_random(); 00048 00049 //Init network core 00050 DBG("init_network: Initializing 6LowPAN network core stack...\r\n"); 00051 net_init_core(); 00052 } 00053 00054 void NSDL_receive_socket(void * cb) 00055 { 00056 socket_callback_t * cb_res =0; 00057 int16_t length; 00058 cb_res = (socket_callback_t *) cb; 00059 uint8_t *payload; 00060 00061 if(cb_res->event_type == SOCKET_DATA) 00062 { 00063 DBG("LINK LQI:"); 00064 debug_hex(cb_res->LINK_LQI); 00065 create_lqi_resource(cb_res->LINK_LQI); 00066 DBG("\r\n"); 00067 00068 if ( cb_res->d_len > 0) 00069 { 00070 payload = (uint8_t *) own_alloc(cb_res->d_len); 00071 if(payload) 00072 { 00073 //Read data to the RX buffer 00074 length = socket_read(cb_res->socket_id, &app_src, payload, cb_res->d_len); //replace rx_buffer payload 00075 if(length) 00076 { 00077 if(cb_res->socket_id == coap_udp_socket) 00078 { 00079 // Handles data received in UDP socket 00080 // Call application protocol parser. 00081 sn_addr_s.type = SN_NSDL_ADDRESS_TYPE_IPV6; 00082 sn_addr_s.addr_len = 16; 00083 sn_addr_s.port = app_src.identifier; 00084 sn_addr_s.addr_ptr = app_src.address; 00085 printf("Data 1\r\n"); 00086 if(sn_nsdl_process_coap(payload, length, &sn_addr_s)) // 0= ok, -1=failure 00087 { 00088 DBG("Error processing CoAP packet\r\n"); 00089 } 00090 printf("Data 4\r\n"); 00091 } 00092 } 00093 own_free(payload); 00094 } 00095 } 00096 } 00097 #if 1 // enabled for debug 00098 else if(cb_res->event_type == SOCKET_TX_DONE) 00099 { 00100 //DBG("*"); 00101 } 00102 else if(cb_res->event_type == SOCKET_NO_ROUTE) 00103 { 00104 DBG("SOCKET_NO_ROUTE\r\n"); 00105 } 00106 else if(cb_res->event_type == SOCKET_TX_FAIL) 00107 { 00108 DBG("SOCKET_TX_FAIL\r\n"); 00109 } 00110 #endif 00111 } 00112 00113 00114 /** 00115 * \brief Network state event handler. 00116 * \param event show network start response or current network state. 00117 * 00118 * - NET_READY: Save NVK peristant data to NVM and Net role 00119 * - NET_NO_BEACON: Link Layer Active Scan Fail, Stack is Already at Idle state 00120 * - NET_NO_ND_ROUTER: No ND Router at current Channel Stack is Already at Idle state 00121 * - NET_BORDER_ROUTER_LOST: Connection to Access point is lost wait for Scan Result 00122 * - NET_PARENT_POLL_FAIL: Host should run net start without any PAN-id filter and all channels 00123 * - NET_PANA_SERVER_AUTH_FAIL: Pana Authentication fail, Stack is Already at Idle state 00124 */ 00125 void app_parse_network_event(arm_event_s *event ) 00126 { 00127 arm_nwk_interface_status_type_e status = (arm_nwk_interface_status_type_e)event->event_data; 00128 switch (status) 00129 { 00130 case ARM_NWK_BOOTSTRAP_READY: 00131 /* NEtwork is ready and node is connected to Access Point */ 00132 if(access_point_status==0) 00133 { 00134 uint8_t temp_ipv6[16]; 00135 DBG("Network Connection Ready\r\n"); 00136 access_point_status=1; 00137 //Read Address 00138 00139 if( arm_nwk_nd_address_read(net_6lowpan_id,&app_nd_address_info) != 0) 00140 { 00141 DBG("ND Address read fail\r\n"); 00142 } 00143 else 00144 { 00145 DBG("ND Access Point: "); 00146 printf_ipv6_address(app_nd_address_info.border_router); //REVIEW 00147 00148 DBG("ND Prefix 64: "); 00149 printf_array(app_nd_address_info.prefix, 8); //REVIEW 00150 00151 if(arm_net_address_get(net_6lowpan_id,ADDR_IPV6_GP,temp_ipv6) == 0) 00152 { 00153 DBG("GP IPv6: "); 00154 printf_ipv6_address(temp_ipv6); //REVIEW 00155 } 00156 } 00157 00158 if( arm_nwk_mac_address_read(net_6lowpan_id,&app_link_address_info) != 0) 00159 { 00160 DBG("MAC Address read fail\r\n"); 00161 } 00162 else 00163 { 00164 uint8_t temp[2]; 00165 common_write_16_bit(app_link_address_info.mac_short,temp); 00166 DBG("MAC 16-bit: "); 00167 printf_array(temp, 2); //REVIEW 00168 common_write_16_bit(app_link_address_info.PANId,temp); 00169 DBG("PAN-ID: "); 00170 printf_array(temp, 2); //REVIEW 00171 DBG("MAC 64-bit: "); 00172 printf_array(app_link_address_info.long_euid64, 8); //REVIEW 00173 DBG("EUID64(Based on MAC 64-bit address): "); 00174 printf_array(app_link_address_info.euid64, 8); //REVIEW 00175 } 00176 } 00177 break; 00178 00179 case ARM_NWK_NWK_SCAN_FAIL: 00180 /* Link Layer Active Scan Fail, Stack is Already at Idle state */ 00181 DBG("Link Layer Scan Fail: No Beacons\r\n"); 00182 access_point_status=0; 00183 //dnssd_disable(1); 00184 break; 00185 00186 case ARM_NWK_IP_ADDRESS_ALLOCATION_FAIL: 00187 /* No ND Router at current Channel Stack is Already at Idle state */ 00188 DBG("ND Scan/ GP REG fail\r\n"); 00189 access_point_status=0; 00190 //dnssd_disable(1); 00191 break; 00192 00193 case ARM_NWK_NWK_CONNECTION_DOWN: 00194 /* Connection to Access point is lost wait for Scan Result */ 00195 DBG("ND/RPL scan new network\r\n"); 00196 access_point_status=0; 00197 break; 00198 00199 case ARM_NWK_NWK_PARENT_POLL_FAIL: 00200 DBG("Network parent poll failed\r\n"); 00201 access_point_status=0; 00202 break; 00203 00204 case ARM_NWK_AUHTENTICATION_FAIL: 00205 DBG("Network authentication fail\r\n"); 00206 access_point_status=0; 00207 break; 00208 00209 default: 00210 debug_hex(status); //REVIEW 00211 DBG("Unknown event"); 00212 break; 00213 } 00214 00215 if(access_point_status == 0) 00216 { 00217 //Set Timer for new Trig 00218 timer_sys_event(RETRY_TIMER, 10000); 00219 } 00220 } 00221 00222 00223 /** 00224 * \brief Handler for events sent to the application. 00225 * \param event received event. 00226 * 00227 * - EV_NETWORK event, Network Event state event handler 00228 * - EV_INIT, Set Certificate Chain list, init multicast, Start net start if NVM have session 00229 * - EV_DEBUG, Terminal handler 00230 */ 00231 void tasklet_main(arm_event_s *event) 00232 { 00233 if(event->sender == 0) 00234 { 00235 arm_library_event_type_e event_type; 00236 event_type = (arm_library_event_type_e)event->event_type; 00237 00238 switch(event_type) 00239 { 00240 case ARM_LIB_NWK_INTERFACE_EVENT: 00241 /* Network Event state event handler */ 00242 DBG("Event: ARM_LIB_NWK_INTERFACE\r\n"); 00243 app_parse_network_event(event); 00244 break; 00245 00246 case ARM_LIB_TASKLET_INIT_EVENT: 00247 /*Init event from stack at start-up*/ 00248 DBG("Event: ARM_LIB_TASKLET_INIT\r\n"); 00249 multicast_set_parameters(10,0,20,3,75 ); 00250 00251 net_6lowpan_id = arm_nwk_interface_init(NET_INTERFACE_RF_6LOWPAN, rf_phy_device_register_id, "6LoWPAN_BORDER_ROUTER"); 00252 if(net_6lowpan_id < 0) 00253 { 00254 DBG("Interface Generate Fail\r\n"); 00255 while(1); 00256 } 00257 else 00258 { 00259 //SET Bootsrap 00260 if(arm_nwk_interface_configure_6lowpan_bootstrap_set(net_6lowpan_id, NET_6LOWPAN_HOST, 1) != 0) // Last parameter enables MLE protocol 00261 { 00262 //Bootsrap SET fail 00263 DBG("Bootstrap Fail\r\n"); 00264 while(1); 00265 } 00266 else 00267 { 00268 int8_t retval = -1; 00269 arm_nwk_6lowpan_gp_address_mode(net_6lowpan_id, NET_6LOWPAN_GP16_ADDRESS, NODE_SHORT_ADDRESS, 1); // 5 = short address for link-layer // 1 = generate automatically if duplicate address is encountered 00270 arm_nwk_link_layer_security_mode(net_6lowpan_id, NET_SEC_MODE_NO_LINK_SECURITY, 0, 0); 00271 arm_nwk_6lowpan_link_scan_paramameter_set(rf_phy_device_register_id, channel_list, 5); 00272 retval = arm_nwk_interface_up(net_6lowpan_id); 00273 if(retval != 0) 00274 { 00275 //6Lowpan Bootsrap start fail 00276 DBG("6LowPAN Bootstrap start Fail\r\n"); 00277 while(1); 00278 } 00279 else 00280 { 00281 //6Lowpan Bootsrap start OK 00282 DBG("6LowPAN Bootstrap Start OK\r\n"); 00283 } 00284 // open sockets 00285 coap_udp_socket = socket_open(SOCKET_UDP, AUDP_SOCKET_PORT, NSDL_receive_socket); 00286 } 00287 timer_sys_event(NSP_REGISTRATION_TIMER, NSP_RD_UPDATE_PERIOD); 00288 } 00289 break; 00290 00291 case ARM_LIB_SYSTEM_TIMER_EVENT: 00292 timer_sys_event_cancel(event->event_id); 00293 if (event->event_id == NSP_REGISTRATION_TIMER) 00294 { 00295 printf("Time to register...\r\n"); 00296 NSP_registration(); 00297 timer_sys_event(NSP_REGISTRATION_TIMER, NSP_RD_UPDATE_PERIOD); 00298 } 00299 else if (event->event_id == RETRY_TIMER) 00300 { 00301 DBG("Event: ARM_LIB_SYSTEM_TIMER (event_id = 1)\r\n"); 00302 int8_t retval = -1; 00303 retval = arm_nwk_interface_up(net_6lowpan_id); 00304 if(retval != 0) 00305 { 00306 //6Lowpan Bootsrap start fail 00307 DBG("6LowPAN Bootstrap Start Failure\r\n"); 00308 while(1); 00309 } 00310 else 00311 { 00312 //6Lowpan Bootsrap start OK 00313 DBG("6LowPAN Bootstrap Start OK\r\n"); 00314 } 00315 } 00316 break; 00317 00318 default: 00319 break; 00320 } 00321 } 00322 } 00323 00324 void app_heap_error_handler(heap_fail_t event) { 00325 switch (event) 00326 { 00327 case NS_DYN_MEM_NULL_FREE: 00328 break; 00329 case NS_DYN_MEM_DOUBLE_FREE: 00330 break; 00331 case NS_DYN_MEM_ALLOCATE_SIZE_NOT_VALID: 00332 break; 00333 case NS_DYN_MEM_POINTER_NOT_VALID: 00334 break; 00335 case NS_DYN_MEM_HEAP_SECTOR_CORRUPTED: 00336 break; 00337 case NS_DYN_MEM_HEAP_SECTOR_UNITIALIZED: 00338 break; 00339 default: 00340 break; 00341 } 00342 while(1); 00343 } 00344 00345
Generated on Fri Jul 15 2022 08:02:55 by
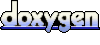