
finish homework2
Embed:
(wiki syntax)
Show/hide line numbers
Car.cpp
00001 //#include "Car.h" 00002 //#include "signal_wrapper.h" 00003 // 00004 //int car_position; 00005 //extern int global_sim; 00006 //// 00007 //Car::Car(int id) { 00008 // this->id = id; 00009 // 00010 // // Include any other necessary initialization 00011 // this->tick = 0; 00012 // 00013 // thread = NULL; // Initialize thread to null, since the starting of the simulation is done in reset(-) 00014 //} 00015 // 00016 //// gets new speed sets tick to 0 00017 //void Car::new_speed() 00018 //{ 00019 // int temp_speed = 0; 00020 // // get a valid speed 5-15 00021 // while( temp_speed < 5 ) 00022 // { 00023 // temp_speed = rand() %16; 00024 // } 00025 // this->speed = temp_speed; 00026 // 00027 // this->tick = 0; 00028 //} 00029 // 00030 //void Car::update_pos() 00031 //{ 00032 // // update speed every 5 ticks 00033 // if(this->tick == 5) 00034 // { 00035 // new_speed(); 00036 // } 00037 // 00038 // // update position based on speed 00039 // this->position += this->speed; 00040 // this->tick++; 00041 // 00042 // // globally shared between car and ACC 00043 // car_position = this->position; 00044 //} 00045 // 00046 //void Car::update() { 00047 // while (true) { 00048 // // wait for a signal from main thread 00049 // uint32_t flags = wait_for_signal( CAR_SIGNAL | SIG_TERM ); 00050 // 00051 // if(flags == SIG_TERM) 00052 // { 00053 // // clean up 00054 // thread->terminate(); 00055 // } 00056 // else if( flags == CAR_SIGNAL ) 00057 // { 00058 // update_pos(); 00059 // } 00060 // // unknown signal 00061 // else 00062 // { 00063 // assert(0); 00064 // } 00065 // 00066 // send_signal( CAR_UPDATE_MAIN_SIGNAL ); 00067 // } 00068 //} 00069 // 00070 //int Car::is_simulating() 00071 //{ 00072 // return simulation; 00073 //} 00074 // 00075 //void Car::reset(int speed, int position) { 00076 // // handle any necessary coordination with main thread 00077 // 00078 // // reset any other attributes that may be necessary 00079 // // ... 00080 // // ... 00081 // 00082 // if (thread != NULL) { // Clear out the existing thread, if it exists, since we don't know how long it has waited for 00083 // delete thread; 00084 // } 00085 // 00086 // thread = new Thread(); 00087 // // Create a new thread for the car 00088 // thread->start( callback(this, &Car::update) ); // Start the thread with the car's update method 00089 // 00090 // this->simulation = 1; 00091 // this->tick = 0; 00092 // this->position = position; 00093 // this->speed = speed; 00094 //}
Generated on Thu Jul 14 2022 17:18:57 by
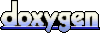