
LED式日時計
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 // for debug 00004 DigitalOut leds[] = { 00005 DigitalOut( LED1 ), 00006 DigitalOut( LED2 ), 00007 DigitalOut( LED3 ), 00008 DigitalOut( LED4 ) 00009 }; 00010 Serial pc( USBTX, USBRX ); 00011 00012 //TA7774PG 00013 class Stepper { 00014 public: 00015 Stepper(PinName in_a, PinName in_b, PinName pws) : _in_a(in_a), _in_b(in_b), _pws(pws) { 00016 _in_a = 0; 00017 _in_b = 0; 00018 _pws = 0; 00019 _next_a = true; 00020 } 00021 void next() { 00022 //_pws = 0; 00023 //wait(1.0); // for pws 00024 if( _next_a ) { 00025 _in_a = !_in_a; 00026 } else { 00027 _in_b = !_in_b; 00028 } 00029 //_pws = 1; 00030 _next_a = !_next_a; 00031 } 00032 private: 00033 DigitalOut _in_a; 00034 DigitalOut _in_b; 00035 DigitalOut _pws; 00036 bool _next_a; 00037 }; 00038 00039 Ticker ti; 00040 Stepper st( p23, p22, p21 ); 00041 00042 // 12hour = 720min to 360 step (mode 1) 00043 const float DAILY_INTERVAL = 120.0; 00044 // 1hour = 60min to 720 step (mode 2) 00045 const float HOURLY_INTERVAL = 10.0; 00046 // for adjust (mode 3) 00047 const float ADJUST_INTERVAL = 1.0; 00048 00049 // switch mode 00050 DigitalIn sw_a(p24, PullUp); 00051 DigitalIn sw_b(p25, PullUp); 00052 int mode = 1; 00053 00054 int main() { 00055 ti.attach(&st, &Stepper::next, DAILY_INTERVAL); 00056 wait(2.0); 00057 // for( int i=0; i < 360; i++ ){ 00058 // leds[0] = !leds[0]; 00059 // st.next(); 00060 // wait(2.0); 00061 // } 00062 00063 while ( true ) { 00064 int previous_mode = mode; 00065 if ( sw_a && sw_b ) { 00066 mode = 1; 00067 } else if ( sw_a ) { 00068 mode = 2; 00069 } else { 00070 mode = 3; 00071 } 00072 if ( previous_mode != mode ) { 00073 ti.detach(); 00074 switch ( mode ) { 00075 case 1: 00076 ti.attach(&st, &Stepper::next, DAILY_INTERVAL); 00077 break; 00078 case 2: 00079 ti.attach(&st, &Stepper::next, HOURLY_INTERVAL); 00080 break; 00081 case 3: 00082 ti.attach(&st, &Stepper::next, ADJUST_INTERVAL); 00083 break; 00084 } 00085 } 00086 leds[3] = !leds[3]; 00087 wait(1.0); 00088 } 00089 }
Generated on Thu Jul 14 2022 07:10:50 by
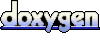