This library sets up and LED volume indication function and reading values from the Electret Microphone - MAX4466 Amplifier
Dependents: MAX4466_Hello_World
MAX4466.cpp
00001 #include "mbed.h" 00002 #include "MAX4466.h" 00003 00004 MAX4466::MAX4466(PinName pin): _pin(pin), _led1(LED1), _led2(LED2), _led3(LED3), _led4(LED4) { 00005 calibration(); 00006 } 00007 00008 //CALIBRATION FUNCTION: 00009 //Find average sound level at the intialization of the microphone 00010 //Use this in the calculation of the LED indication array values 00011 float MAX4466::calibration() { 00012 00013 _t.start(); 00014 _t1.start(); 00015 float peakToPeak=0, signalMax=0, signalMin=1024; 00016 00017 while (_t1.read()<1) { 00018 while (_t.read_ms()<50) { 00019 _sample=_pin.read(); 00020 00021 if (_sample<1024) { 00022 if (_sample>signalMax) 00023 signalMax=_sample; 00024 00025 else if (_sample<signalMin) 00026 signalMin=_sample; 00027 } 00028 } 00029 _t.reset(); 00030 peakToPeak=signalMax-signalMin; 00031 _value= (peakToPeak*3.3); 00032 _value = floor(_value * 100) / 100; 00033 _sum+=_value; 00034 _count++; 00035 } 00036 _average=_sum/_count; 00037 _t1.reset(); 00038 00039 return _average; 00040 } 00041 00042 //LED ARRAY FUNCTION: 00043 //Setup array of 4 LEDs 00044 //The input to this function is the average from the calibration function 00045 //The variable _value which indicates the current value from the microphone is a global variable updated in the sound_level function 00046 //As different threshholds of volume are met more LEDs will light up 00047 void MAX4466::led_array(float x ) { 00048 00049 if (_value<x+0.05) { 00050 _led1=0; 00051 _led2=0; 00052 _led3=0; 00053 _led4=0; 00054 } 00055 if (_value>x+0.05&&_value<0.5+x) { 00056 _led1=1; 00057 _led2=0; 00058 _led3=0; 00059 _led4=0; 00060 } 00061 if (_value>0.5+x&&_value<1+x) { 00062 _led1=1; 00063 _led2=1; 00064 _led3=0; 00065 _led4=0; 00066 } 00067 if (_value>1+x&&_value<1.2+x) { 00068 _led1=1; 00069 _led2=1; 00070 _led3=1; 00071 _led4=0; 00072 } 00073 if (_value>1.2+x&&_value<2.8+x) { 00074 _led1=1; 00075 _led2=1; 00076 _led3=1; 00077 _led4=1; 00078 } 00079 } 00080 00081 //SOUND LEVEL FUNCTIOM: 00082 //Read in current sound level from the microphone 00083 //Update the global variable _value which will in turn be read by the LED array function 00084 float MAX4466::sound_level() { 00085 00086 _t.start(); 00087 float peakToPeak=0, signalMax=0, signalMin=1024; 00088 00089 while (_t.read_ms()<50) { 00090 _sample=_pin.read(); 00091 00092 if (_sample<1024) { 00093 if (_sample>signalMax) 00094 signalMax=_sample; 00095 00096 else if (_sample<signalMin) 00097 signalMin=_sample; 00098 } 00099 } 00100 _t.reset(); 00101 peakToPeak=signalMax-signalMin; 00102 _value= (peakToPeak*3.3); 00103 _value = floor(_value * 100) / 100; 00104 _sum+=_value; 00105 _count++; 00106 00107 return _value; 00108 } 00109 00110 //VOLUME INDICATOR FUNCTION: 00111 //To be called in main function 00112 //Updates the led array 00113 //outputs current numerical value 00114 void MAX4466::volume_indicator() { 00115 led_array(_average); 00116 sound_level(); 00117 }
Generated on Fri Jul 15 2022 02:23:38 by
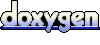