Implementation of 1-Wire with added Alarm Search Functionality
Dependents: Max32630_One_Wire_Interface
DS18B20 Class Reference
DS18B20 Programmable Resolution 1-Wire Digital Thermometer. More...
#include <DS18B20.h>
Inherits OneWire::OneWireSlave.
Public Types | |
enum | Resolution |
Available resolutions of the DS18B20. More... | |
Public Member Functions | |
DS18B20 (RandomAccessRomIterator &selector) | |
DS18B20 constructor. | |
OneWireSlave::CmdResult | writeScratchPad (uint8_t th, uint8_t tl, Resolution res) |
Write Scratchpad Command. | |
OneWireSlave::CmdResult | readScratchPad (uint8_t *scratchPadBuff) |
Read Scratchpad Command. | |
OneWireSlave::CmdResult | copyScratchPad (void) |
Copy Scratchpad Command. | |
OneWireSlave::CmdResult | readPowerSupply (bool &localPower) |
Read Power Supply command. | |
OneWireSlave::CmdResult | convertTemperature (float &temp) |
Convert Temperature Command. | |
OneWireSlave::CmdResult | recallEEPROM (void) |
Recall Command. | |
RomId | romId () const |
Protected Member Functions | |
OneWireMaster::CmdResult | selectDevice () const |
Select this slave device by ROM ID. | |
OneWireMaster & | master () const |
The 1-Wire master for this slave device. |
Detailed Description
DS18B20 Programmable Resolution 1-Wire Digital Thermometer.
The DS18B20 digital thermometer provides 9-bit to 12-bit Celsius temperature measurements and has an alarm function with nonvolatile user-programmable upper and lower trigger points. The DS18B20 communicates over a 1-Wire bus that by definition requires only one data line (and ground) for communication with a central microprocessor. In addition, the DS18B20 can derive power directly from the data line ("parasite power"), eliminating the need for an external power supply.
Definition at line 57 of file DS18B20.h.
Member Enumeration Documentation
enum Resolution |
Constructor & Destructor Documentation
DS18B20 | ( | RandomAccessRomIterator & | selector ) |
DS18B20 constructor.
On Entry:
- Parameters:
-
[in] selector - Reference to RandomAccessRomiteraor object that encapsulates owm master that has access to this device and ROM function commands used to a select device
On Exit:
- Returns:
Definition at line 54 of file DS18B20.cpp.
Member Function Documentation
OneWireSlave::CmdResult convertTemperature | ( | float & | temp ) |
Convert Temperature Command.
This command begins a temperature conversion.
On Entry:
- Parameters:
-
[in] On Exit: [out] temp - temperature conversion results
- Returns:
- CmdResult - result of operation
Definition at line 227 of file DS18B20.cpp.
OneWireSlave::CmdResult copyScratchPad | ( | void | ) |
Copy Scratchpad Command.
This command copies from the scratchpad into the EEPROM of the DS18B20, storing the temperature trigger bytes and resolution in nonvolatile memory.
On Entry:
- Parameters:
-
[in] On Exit: [out]
- Returns:
- CmdResult - result of operation
Definition at line 155 of file DS18B20.cpp.
OneWireMaster& master | ( | ) | const [protected, inherited] |
The 1-Wire master for this slave device.
Definition at line 77 of file OneWireSlave.h.
OneWireSlave::CmdResult readPowerSupply | ( | bool & | localPower ) |
Read Power Supply command.
This command determines if the DS18B20 is parasite powered or has a local supply
On Entry:
- Parameters:
-
[in] On Exit: [out] localPower - Will be False on exit if the DS18B20 is parasite powered
- Returns:
- CmdResult - result of operation
Definition at line 122 of file DS18B20.cpp.
OneWireSlave::CmdResult readScratchPad | ( | uint8_t * | scratchPadBuff ) |
Read Scratchpad Command.
This command reads the complete scratchpad.
On Entry:
- Parameters:
-
[in] scratchPadBuff - array for receiving contents of scratchpad, this buffer will be over written
On Exit:
- Parameters:
-
[out] scratchPadBuff - contents of scratchpad
- Returns:
- CmdResult - result of operation
Definition at line 86 of file DS18B20.cpp.
OneWireSlave::CmdResult recallEEPROM | ( | void | ) |
Recall Command.
This command recalls the temperature trigger values and resolution stored in EEPROM to the scratchpad
On Entry:
- Parameters:
-
[in] On Exit: [out]
- Returns:
- CmdResult - result of operation
Definition at line 329 of file DS18B20.cpp.
RomId romId | ( | ) | const [inherited] |
1-Wire ROM ID for this slave device.
Definition at line 59 of file OneWireSlave.h.
OneWireMaster::CmdResult selectDevice | ( | ) | const [protected, inherited] |
Select this slave device by ROM ID.
Definition at line 74 of file OneWireSlave.h.
OneWireSlave::CmdResult writeScratchPad | ( | uint8_t | th, |
uint8_t | tl, | ||
Resolution | res | ||
) |
Write Scratchpad Command.
If the result of a temperature measurement is higher than TH or lower than TL, an alarm flag inside the device is set. This flag is updated with every temperature measurement. As long as the alarm flag is set, the DS1920 will respond to the alarm search command.
On Entry:
- Parameters:
-
[in] th - 8-bit upper temperature threshold, MSB indicates sign [in] tl - 8-bit lower temperature threshold, LSB indicates sign [in] res - Resolution of the DS18B20
On Exit:
- Parameters:
-
[out]
- Returns:
- CmdResult - result of operation
Definition at line 60 of file DS18B20.cpp.
Generated on Thu Jul 14 2022 03:09:07 by
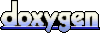