
Test function for CThunk class
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include <mbed.h> 00002 #include <thunk.h> 00003 00004 class CTest 00005 { 00006 public: 00007 CTest(void); 00008 void test(void); 00009 void hexdump(const void* data, int length); 00010 00011 Serial pc; 00012 CThunk<CTest> thunk; 00013 00014 void callback2(void* context); 00015 00016 private: 00017 CThunkIRQ<CTest> m_irq; 00018 DigitalOut m_led1, m_led2, m_led3; 00019 00020 void callback1(void); 00021 void callback_irq(void); 00022 00023 uint32_t m_counter; 00024 }; 00025 00026 CTest::CTest(void) 00027 :pc(USBTX, USBRX), 00028 thunk(this, &CTest::callback1), 00029 m_irq(this, TIMER0_IRQn, &CTest::callback_irq), 00030 m_led1(LED1), 00031 m_led2(LED2), 00032 m_led3(LED3) 00033 { 00034 m_counter = 0; 00035 00036 pc.baud(115200); 00037 00038 /* enable timer */ 00039 LPC_SC->PCONP |= 2; 00040 LPC_TIM0->TCR = 2; 00041 LPC_TIM0->MR0 = 2398000; 00042 LPC_TIM0->MCR = 3; 00043 LPC_TIM0->TCR = 1; 00044 } 00045 00046 void CTest::callback1(void) 00047 { 00048 pc.printf("callback1: called (this=0x%0X)\n", this); 00049 00050 /* turn on LED1 */ 00051 m_led1 = 1; 00052 00053 /* increment member variable */ 00054 pc.printf("callback1: m_counter before: %i\n", m_counter); 00055 m_counter++; 00056 pc.printf("callback1: m_counter after : %i\n", m_counter); 00057 } 00058 00059 void CTest::callback2(void* context) 00060 { 00061 pc.printf("callback2: called with context value 0x%08X\n", context); 00062 00063 /* turn on LED2 */ 00064 m_led2 = 1; 00065 00066 /* increment member variable */ 00067 pc.printf("callback2: m_counter before: %i\n", m_counter); 00068 m_counter+=2; 00069 pc.printf("callback2: m_counter after : %i\n", m_counter); 00070 } 00071 00072 void CTest::callback_irq(void) 00073 { 00074 uint32_t reason = LPC_TIM0->IR; 00075 00076 /* verify reason */ 00077 if(reason & 1) 00078 m_led3 = !m_led3; 00079 00080 /* acknowledge IRQ */ 00081 LPC_TIM0->IR = reason; 00082 } 00083 00084 void CTest::hexdump(const void* data, int length) 00085 { 00086 int i; 00087 00088 pc.printf("Dump %u bytes from 0x%08X\n", length, data); 00089 00090 for(i=0; i<length; i++) 00091 { 00092 if((i%16) == 0) 00093 pc.printf("\n"); 00094 else 00095 if((i%8) == 0) 00096 pc.printf(" - "); 00097 pc.printf("0x%02X ", ((uint8_t*)data)[i]); 00098 } 00099 pc.printf("\n"); 00100 } 00101 00102 int main(void) 00103 { 00104 /* allocate thunking test class */ 00105 CTest test; 00106 /* allocate 32 bit pointer to thunk-entry */ 00107 CThunkEntry entry; 00108 00109 /* to make a point: get 32 bit entry point pointer from thunk */ 00110 entry = test.thunk; 00111 00112 /* TEST1: */ 00113 /* callback function has been set in the CTest constructor */ 00114 test.hexdump((const void*)entry, 20); 00115 /* call entry point */ 00116 entry(); 00117 00118 /* TEST2: */ 00119 /* assign a context ... */ 00120 test.thunk.context(0xDEADBEEF); 00121 /* and switch callback to callback2 */ 00122 test.thunk.callback(&CTest::callback2); 00123 /* call entry point */ 00124 entry(); 00125 00126 /* sit waiting for timer interrupts */ 00127 while(1) 00128 __WFI(); 00129 }
Generated on Sun Jul 24 2022 10:41:13 by
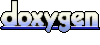